
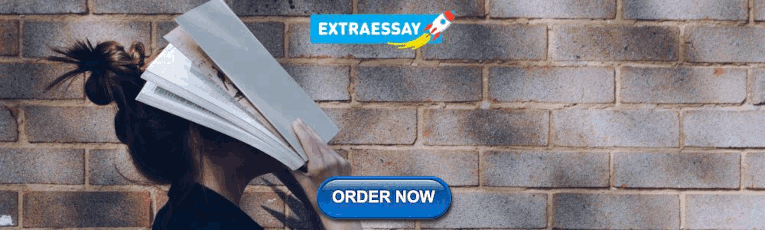
Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! đ

- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- PortuguĂȘs (do Brasil)
Conditional (ternary) operator
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark ( ? ), then an expression to execute if the condition is truthy followed by a colon ( : ), and finally the expression to execute if the condition is falsy . This operator is frequently used as an alternative to an if...else statement.
An expression whose value is used as a condition.
An expression which is executed if the condition evaluates to a truthy value (one which equals or can be converted to true ).
An expression which is executed if the condition is falsy (that is, has a value which can be converted to false ).
Description
Besides false , possible falsy expressions are: null , NaN , 0 , the empty string ( "" ), and undefined . If condition is any of these, the result of the conditional expression will be the result of executing the expression exprIfFalse .
A simple example
Handling null values.
One common usage is to handle a value that may be null :
Conditional chains
The ternary operator is right-associative, which means it can be "chained" in the following way, similar to an if ⊠else if ⊠else if ⊠else chain:
This is equivalent to the following if...else chain.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Nullish coalescing operator ( ?? )
- Optional chaining ( ?. )
- Making decisions in your code â conditionals
- Expressions and operators guide
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?: operator - the ternary conditional operator
- 11 contributors
The conditional operator ?: , also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false , as the following example shows:
As the preceding example shows, the syntax for the conditional operator is as follows:
The condition expression must evaluate to true or false . If condition evaluates to true , the consequent expression is evaluated, and its result becomes the result of the operation. If condition evaluates to false , the alternative expression is evaluated, and its result becomes the result of the operation. Only consequent or alternative is evaluated. Conditional expressions are target-typed. That is, if a target type of a conditional expression is known, the types of consequent and alternative must be implicitly convertible to the target type, as the following example shows:
If a target type of a conditional expression is unknown (for example, when you use the var keyword) or the type of consequent and alternative must be the same or there must be an implicit conversion from one type to the other:
The conditional operator is right-associative, that is, an expression of the form
is evaluated as
You can use the following mnemonic device to remember how the conditional operator is evaluated:
Conditional ref expression
A conditional ref expression conditionally returns a variable reference, as the following example shows:
You can ref assign the result of a conditional ref expression, use it as a reference return or pass it as a ref , out , in , or ref readonly method parameter . You can also assign to the result of a conditional ref expression, as the preceding example shows.
The syntax for a conditional ref expression is as follows:
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative .
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed.
Conditional operator and an if statement
Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following example demonstrates two ways to classify an integer as negative or nonnegative:
Operator overloadability
A user-defined type can't overload the conditional operator.
C# language specification
For more information, see the Conditional operator section of the C# language specification .
Specifications for newer features are:
- Target-typed conditional expression
- Simplify conditional expression (style rule IDE0075)
- C# operators and expressions
- if statement
- ?. and ?[] operators
- ?? and ??= operators
- ref keyword
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Previous: Immediately Expanded Variable Assignment , Up: The Two Flavors of Variables [ Contents ][ Index ]
6.2.4 Conditional Variable Assignment
There is another assignment operator for variables, ‘ ?= ’. This is called a conditional variable assignment operator, because it only has an effect if the variable is not yet defined. This statement:
is exactly equivalent to this (see The origin Function ):
Note that a variable set to an empty value is still defined, so ‘ ?= ’ will not set that variable.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
C Data Types
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
C Operators
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- C Functions
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessors
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- Whatâs difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
C File Handling
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
C Interview Questions
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.
Syntax of Conditional/Ternary Operator in C
The conditional operator can be in the form
Or the syntax can also be in this form
Or syntax can also be in this form
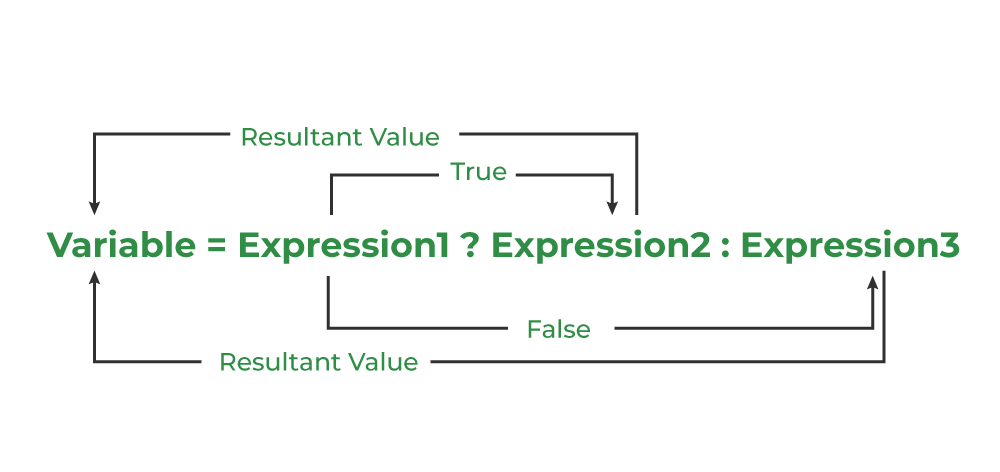
Conditional/Ternary Operator in C
It can be visualized into an if-else statement as:
Since the Conditional Operator ‘?:’ takes three operands to work, hence they are also called ternary operators .
Note: The ternary operator have third most lowest precedence, so we need to use the expressions such that we can avoid errors due to improper operator precedence management.
Working of Conditional/Ternary Operator in C
The working of the conditional operator in C is as follows:
- Step 1: Expression1 is the condition to be evaluated.
- Step 2A: If the condition( Expression1 ) is True then Expression2 will be executed.
- Step 2B : If the condition( Expression1 ) is false then Expression3 will be executed.
- Step 3: Results will be returned.
Flowchart of Conditional/Ternary Operator in C
To understand the working better, we can analyze the flowchart of the conditional operator given below.
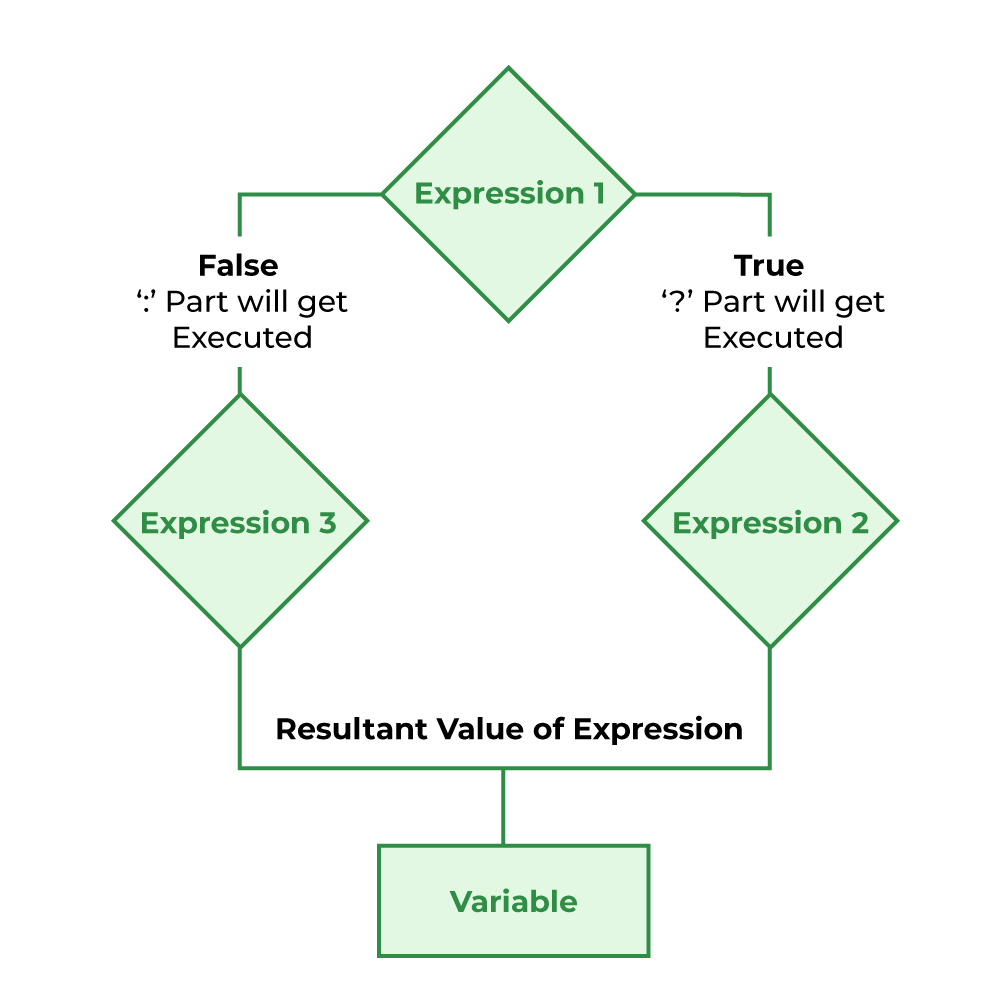
Flowchart of conditional/ternary operator in C
Examples of C Ternary Operator
Example 1: c program to store the greatest of the two numbers using the ternary operator.
Example 2: C Program to check whether a year is a leap year using ternary operator
The conditional operator or ternary operator in C is generally used when we need a short conditional code such as assigning value to a variable based on the condition. It can be used in bigger conditions but it will make the program very complex and unreadable.
FAQs on Conditional/Ternary Operators in C
1. what is the ternary operator in c.
The ternary operator in C is a conditional operator that works on three operands. It works similarly to the if-else statement and executes the code based on the specified condition. It is also called conditional Operator
2. What is the advantage of the conditional operator?
It reduces the line of code when the condition and statements are small.
Please Login to comment...
Similar reads.
- C# Programs
- C/C++ Puzzles
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Verilog Conditional Statements
In Verilog, conditional statements are used to control the flow of execution based on certain conditions. There are several types of conditional statements in Verilog listed below.
Conditional Operator
The conditional operator allows you to assign a value to a variable based on a condition. If the condition is true, expression_1 is assigned to the variable. Otherwise, expression_2 is assigned.
Nested conditional operators
Conditional operators can be nested to any level but it can affect readability of code.
Here are some of the advantages of using conditional operators:
- Concise syntax : The conditional operator allows for a compact and concise representation of conditional assignments. It reduces the amount of code needed compared to using if-else statements or case statements.
- Readability : The conditional operator can enhance code readability, especially for simple conditional assignments. It clearly expresses the intent of assigning different values based on a condition in a single line.
And some disadvantages:
- Limited functionality : The conditional operator is primarily used for simple conditional assignments. It may not be suitable for complex conditions or multiple actions, as it can quickly become unreadable and difficult to maintain.
- Lack of flexibility : The conditional operator only allows for a binary choice based on the condition. It cannot handle multiple cases or multiple actions within a single line of code.
- Potential for reduced readability : While the conditional operator can enhance code readability for simple assignments, it can also make the code more difficult to understand if the condition and assigned values become complex.
if-else statement
The if-else statement allows you to perform different actions based on a condition.
If the condition evaluates to true, statement 1 is executed. Otherwise, statement 2 is executed.
Read more on Verilog if-else-if statements
case statement
The case statement is used when you have multiple conditions and want to perform different actions based on the value of a variable.
The expression is evaluated, and based on its value, the corresponding statement is executed. If none of the values match the expression, the statement under default is executed.
Read more on Verilog case statement
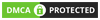
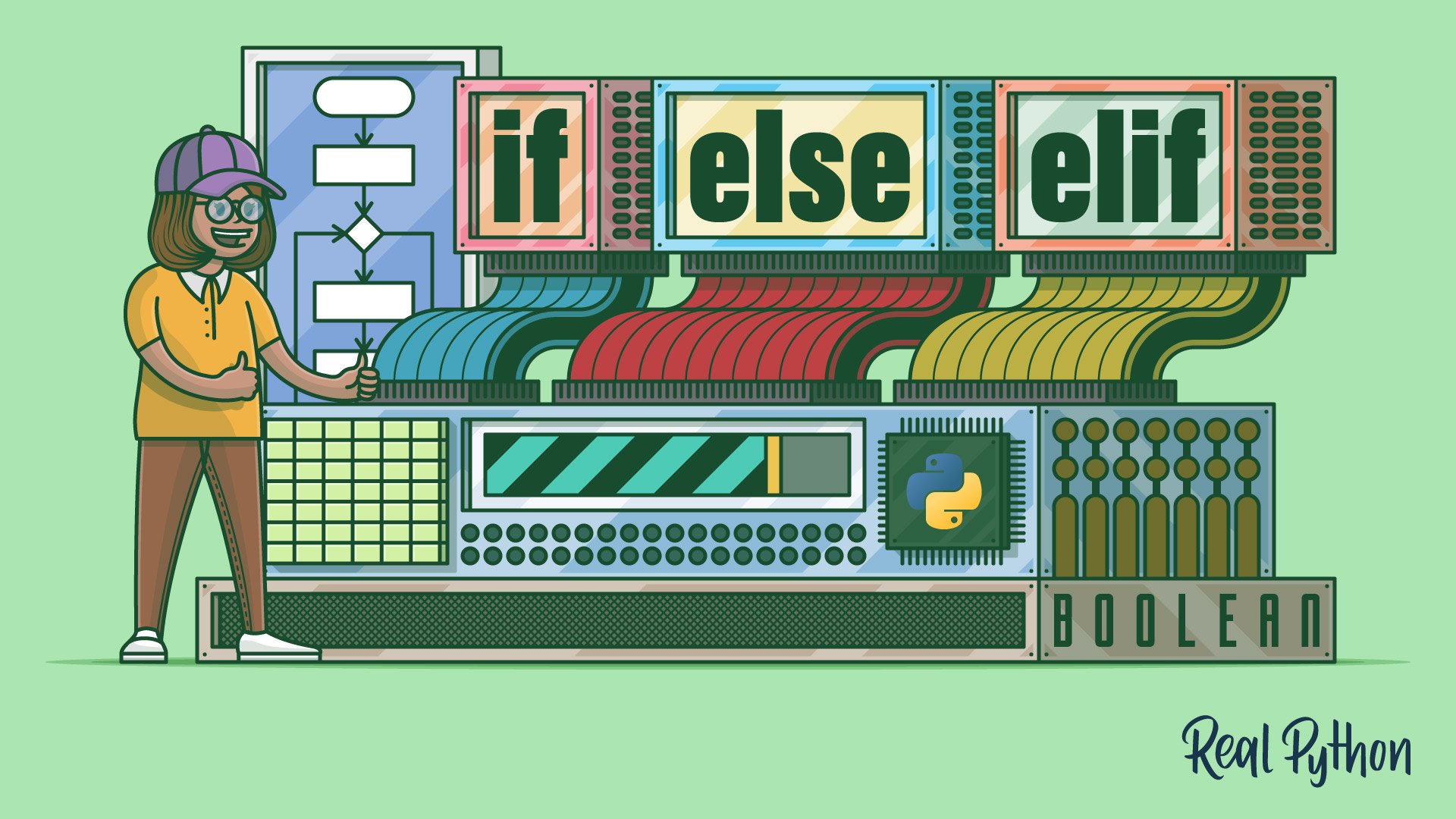
Conditional Statements in Python
Table of Contents
Introduction to the if Statement
Python: it’s all about the indentation, what do other languages do, which is better, the else and elif clauses, one-line if statements, conditional expressions (python’s ternary operator), the python pass statement.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Conditional Statements in Python (if/elif/else)
From the previous tutorials in this series, you now have quite a bit of Python code under your belt. Everything you have seen so far has consisted of sequential execution , in which statements are always performed one after the next, in exactly the order specified.
But the world is often more complicated than that. Frequently, a program needs to skip over some statements, execute a series of statements repetitively, or choose between alternate sets of statements to execute.
That is where control structures come in. A control structure directs the order of execution of the statements in a program (referred to as the program’s control flow ).
Here’s what you’ll learn in this tutorial: You’ll encounter your first Python control structure, the if statement.
In the real world, we commonly must evaluate information around us and then choose one course of action or another based on what we observe:
If the weather is nice, then I’ll mow the lawn. (It’s implied that if the weather isn’t nice, then I won’t mow the lawn.)
In a Python program, the if statement is how you perform this sort of decision-making. It allows for conditional execution of a statement or group of statements based on the value of an expression.
The outline of this tutorial is as follows:
- First, you’ll get a quick overview of the if statement in its simplest form.
- Next, using the if statement as a model, you’ll see why control structures require some mechanism for grouping statements together into compound statements or blocks . You’ll learn how this is done in Python.
- Lastly, you’ll tie it all together and learn how to write complex decision-making code.
Ready? Here we go!
Take the Quiz: Test your knowledge with our interactive âPython Conditional Statementsâ quiz. Youâll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Test your understanding of Python conditional statements
We’ll start by looking at the most basic type of if statement. In its simplest form, it looks like this:
In the form shown above:
- <expr> is an expression evaluated in a Boolean context, as discussed in the section on Logical Operators in the Operators and Expressions in Python tutorial.
- <statement> is a valid Python statement, which must be indented. (You will see why very soon.)
If <expr> is true (evaluates to a value that is “truthy”), then <statement> is executed. If <expr> is false, then <statement> is skipped over and not executed.
Note that the colon ( : ) following <expr> is required. Some programming languages require <expr> to be enclosed in parentheses, but Python does not.
Here are several examples of this type of if statement:
Note: If you are trying these examples interactively in a REPL session, you’ll find that, when you hit Enter after typing in the print('yes') statement, nothing happens.
Because this is a multiline statement, you need to hit Enter a second time to tell the interpreter that you’re finished with it. This extra newline is not necessary in code executed from a script file.
Grouping Statements: Indentation and Blocks
So far, so good.
But let’s say you want to evaluate a condition and then do more than one thing if it is true:
If the weather is nice, then I will: Mow the lawn Weed the garden Take the dog for a walk (If the weather isn’t nice, then I won’t do any of these things.)
In all the examples shown above, each if <expr>: has been followed by only a single <statement> . There needs to be some way to say “If <expr> is true, do all of the following things.”
The usual approach taken by most programming languages is to define a syntactic device that groups multiple statements into one compound statement or block . A block is regarded syntactically as a single entity. When it is the target of an if statement, and <expr> is true, then all the statements in the block are executed. If <expr> is false, then none of them are.
Virtually all programming languages provide the capability to define blocks, but they don’t all provide it in the same way. Let’s see how Python does it.
Python follows a convention known as the off-side rule , a term coined by British computer scientist Peter J. Landin. (The term is taken from the offside law in association football.) Languages that adhere to the off-side rule define blocks by indentation. Python is one of a relatively small set of off-side rule languages .
Recall from the previous tutorial on Python program structure that indentation has special significance in a Python program. Now you know why: indentation is used to define compound statements or blocks. In a Python program, contiguous statements that are indented to the same level are considered to be part of the same block.
Thus, a compound if statement in Python looks like this:
Here, all the statements at the matching indentation level (lines 2 to 5) are considered part of the same block. The entire block is executed if <expr> is true, or skipped over if <expr> is false. Either way, execution proceeds with <following_statement> (line 6) afterward.
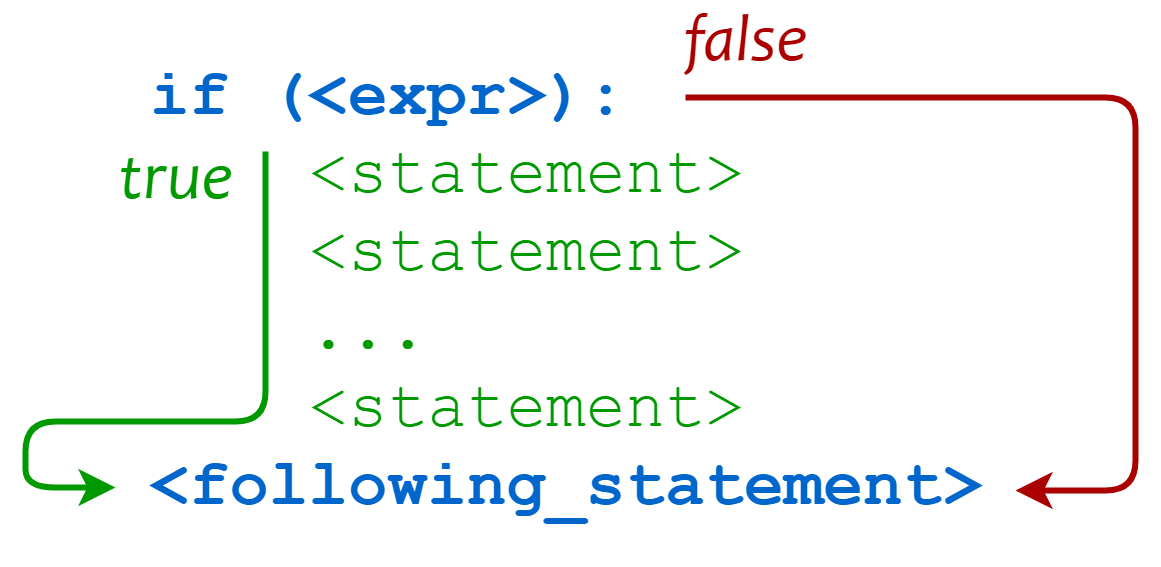
Notice that there is no token that denotes the end of the block. Rather, the end of the block is indicated by a line that is indented less than the lines of the block itself.
Note: In the Python documentation, a group of statements defined by indentation is often referred to as a suite . This tutorial series uses the terms block and suite interchangeably.
Consider this script file foo.py :
Running foo.py produces this output:
The four print() statements on lines 2 to 5 are indented to the same level as one another. They constitute the block that would be executed if the condition were true. But it is false, so all the statements in the block are skipped. After the end of the compound if statement has been reached (whether the statements in the block on lines 2 to 5 are executed or not), execution proceeds to the first statement having a lesser indentation level: the print() statement on line 6.
Blocks can be nested to arbitrary depth. Each indent defines a new block, and each outdent ends the preceding block. The resulting structure is straightforward, consistent, and intuitive.
Here is a more complicated script file called blocks.py :
The output generated when this script is run is shown below:
Note: In case you have been wondering, the off-side rule is the reason for the necessity of the extra newline when entering multiline statements in a REPL session. The interpreter otherwise has no way to know that the last statement of the block has been entered.
Perhaps you’re curious what the alternatives are. How are blocks defined in languages that don’t adhere to the off-side rule?
The tactic used by most programming languages is to designate special tokens that mark the start and end of a block. For example, in Perl blocks are defined with pairs of curly braces ( {} ) like this:
C/C++, Java , and a whole host of other languages use curly braces in this way.
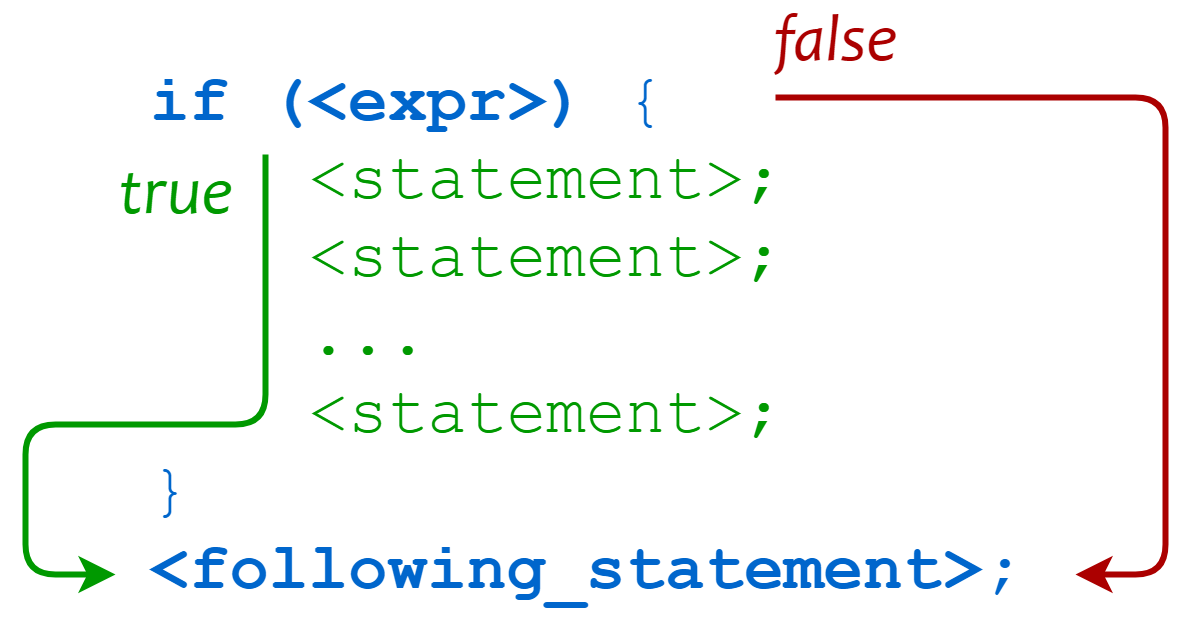
Other languages, such as Algol and Pascal, use keywords begin and end to enclose blocks.
Better is in the eye of the beholder. On the whole, programmers tend to feel rather strongly about how they do things. Debate about the merits of the off-side rule can run pretty hot.
On the plus side:
- Python’s use of indentation is clean, concise, and consistent.
- In programming languages that do not use the off-side rule, indentation of code is completely independent of block definition and code function. It’s possible to write code that is indented in a manner that does not actually match how the code executes, thus creating a mistaken impression when a person just glances at it. This sort of mistake is virtually impossible to make in Python.
- Use of indentation to define blocks forces you to maintain code formatting standards you probably should be using anyway.
On the negative side:
- Many programmers don’t like to be forced to do things a certain way. They tend to have strong opinions about what looks good and what doesn’t, and they don’t like to be shoehorned into a specific choice.
- Some editors insert a mix of space and tab characters to the left of indented lines, which makes it difficult for the Python interpreter to determine indentation levels. On the other hand, it is frequently possible to configure editors not to do this. It generally isn’t considered desirable to have a mix of tabs and spaces in source code anyhow, no matter the language.
Like it or not, if you’re programming in Python, you’re stuck with the off-side rule. All control structures in Python use it, as you will see in several future tutorials.
For what it’s worth, many programmers who have been used to languages with more traditional means of block definition have initially recoiled at Python’s way but have gotten comfortable with it and have even grown to prefer it.
Now you know how to use an if statement to conditionally execute a single statement or a block of several statements. It’s time to find out what else you can do.
Sometimes, you want to evaluate a condition and take one path if it is true but specify an alternative path if it is not. This is accomplished with an else clause:
If <expr> is true, the first suite is executed, and the second is skipped. If <expr> is false, the first suite is skipped and the second is executed. Either way, execution then resumes after the second suite. Both suites are defined by indentation, as described above.
In this example, x is less than 50 , so the first suite (lines 4 to 5) are executed, and the second suite (lines 7 to 8) are skipped:
Here, on the other hand, x is greater than 50 , so the first suite is passed over, and the second suite executed:
There is also syntax for branching execution based on several alternatives. For this, use one or more elif (short for else if ) clauses. Python evaluates each <expr> in turn and executes the suite corresponding to the first that is true. If none of the expressions are true, and an else clause is specified, then its suite is executed:
An arbitrary number of elif clauses can be specified. The else clause is optional. If it is present, there can be only one, and it must be specified last:
At most, one of the code blocks specified will be executed. If an else clause isn’t included, and all the conditions are false, then none of the blocks will be executed.
Note: Using a lengthy if / elif / else series can be a little inelegant, especially when the actions are simple statements like print() . In many cases, there may be a more Pythonic way to accomplish the same thing.
Here’s one possible alternative to the example above using the dict.get() method:
Recall from the tutorial on Python dictionaries that the dict.get() method searches a dictionary for the specified key and returns the associated value if it is found, or the given default value if it isn’t.
An if statement with elif clauses uses short-circuit evaluation, analogous to what you saw with the and and or operators. Once one of the expressions is found to be true and its block is executed, none of the remaining expressions are tested. This is demonstrated below:
The second expression contains a division by zero, and the third references an undefined variable var . Either would raise an error, but neither is evaluated because the first condition specified is true.
It is customary to write if <expr> on one line and <statement> indented on the following line like this:
But it is permissible to write an entire if statement on one line. The following is functionally equivalent to the example above:
There can even be more than one <statement> on the same line, separated by semicolons:
But what does this mean? There are two possible interpretations:
If <expr> is true, execute <statement_1> .
Then, execute <statement_2> ... <statement_n> unconditionally, irrespective of whether <expr> is true or not.
If <expr> is true, execute all of <statement_1> ... <statement_n> . Otherwise, don’t execute any of them.
Python takes the latter interpretation. The semicolon separating the <statements> has higher precedence than the colon following <expr> —in computer lingo, the semicolon is said to bind more tightly than the colon. Thus, the <statements> are treated as a suite, and either all of them are executed, or none of them are:
Multiple statements may be specified on the same line as an elif or else clause as well:
While all of this works, and the interpreter allows it, it is generally discouraged on the grounds that it leads to poor readability, particularly for complex if statements. PEP 8 specifically recommends against it.
As usual, it is somewhat a matter of taste. Most people would find the following more visually appealing and easier to understand at first glance than the example above:
If an if statement is simple enough, though, putting it all on one line may be reasonable. Something like this probably wouldn’t raise anyone’s hackles too much:
Python supports one additional decision-making entity called a conditional expression. (It is also referred to as a conditional operator or ternary operator in various places in the Python documentation.) Conditional expressions were proposed for addition to the language in PEP 308 and green-lighted by Guido in 2005.
In its simplest form, the syntax of the conditional expression is as follows:
This is different from the if statement forms listed above because it is not a control structure that directs the flow of program execution. It acts more like an operator that defines an expression. In the above example, <conditional_expr> is evaluated first. If it is true, the expression evaluates to <expr1> . If it is false, the expression evaluates to <expr2> .
Notice the non-obvious order: the middle expression is evaluated first, and based on that result, one of the expressions on the ends is returned. Here are some examples that will hopefully help clarify:
Note: Python’s conditional expression is similar to the <conditional_expr> ? <expr1> : <expr2> syntax used by many other languages—C, Perl and Java to name a few. In fact, the ?: operator is commonly called the ternary operator in those languages, which is probably the reason Python’s conditional expression is sometimes referred to as the Python ternary operator.
You can see in PEP 308 that the <conditional_expr> ? <expr1> : <expr2> syntax was considered for Python but ultimately rejected in favor of the syntax shown above.
A common use of the conditional expression is to select variable assignment. For example, suppose you want to find the larger of two numbers. Of course, there is a built-in function, max() , that does just this (and more) that you could use. But suppose you want to write your own code from scratch.
You could use a standard if statement with an else clause:
But a conditional expression is shorter and arguably more readable as well:
Remember that the conditional expression behaves like an expression syntactically. It can be used as part of a longer expression. The conditional expression has lower precedence than virtually all the other operators, so parentheses are needed to group it by itself.
In the following example, the + operator binds more tightly than the conditional expression, so 1 + x and y + 2 are evaluated first, followed by the conditional expression. The parentheses in the second case are unnecessary and do not change the result:
If you want the conditional expression to be evaluated first, you need to surround it with grouping parentheses. In the next example, (x if x > y else y) is evaluated first. The result is y , which is 40 , so z is assigned 1 + 40 + 2 = 43 :
If you are using a conditional expression as part of a larger expression, it probably is a good idea to use grouping parentheses for clarification even if they are not needed.
Conditional expressions also use short-circuit evaluation like compound logical expressions. Portions of a conditional expression are not evaluated if they don’t need to be.
In the expression <expr1> if <conditional_expr> else <expr2> :
- If <conditional_expr> is true, <expr1> is returned and <expr2> is not evaluated.
- If <conditional_expr> is false, <expr2> is returned and <expr1> is not evaluated.
As before, you can verify this by using terms that would raise an error:
In both cases, the 1/0 terms are not evaluated, so no exception is raised.
Conditional expressions can also be chained together, as a sort of alternative if / elif / else structure, as shown here:
It’s not clear that this has any significant advantage over the corresponding if / elif / else statement, but it is syntactically correct Python.
Occasionally, you may find that you want to write what is called a code stub: a placeholder for where you will eventually put a block of code that you haven’t implemented yet.
In languages where token delimiters are used to define blocks, like the curly braces in Perl and C, empty delimiters can be used to define a code stub. For example, the following is legitimate Perl or C code:
Here, the empty curly braces define an empty block. Perl or C will evaluate the expression x , and then even if it is true, quietly do nothing.
Because Python uses indentation instead of delimiters, it is not possible to specify an empty block. If you introduce an if statement with if <expr>: , something has to come after it, either on the same line or indented on the following line.
Consider this script foo.py :
If you try to run foo.py , you’ll get this:
The Python pass statement solves this problem. It doesn’t change program behavior at all. It is used as a placeholder to keep the interpreter happy in any situation where a statement is syntactically required, but you don’t really want to do anything:
Now foo.py runs without error:
With the completion of this tutorial, you are beginning to write Python code that goes beyond simple sequential execution:
- You were introduced to the concept of control structures . These are compound statements that alter program control flow —the order of execution of program statements.
- You learned how to group individual statements together into a block or suite .
- You encountered your first control structure, the if statement, which makes it possible to conditionally execute a statement or block based on evaluation of program data.
All of these concepts are crucial to developing more complex Python code.
The next two tutorials will present two new control structures: the while statement and the for statement. These structures facilitate iteration , execution of a statement or block of statements repeatedly.
đ Python Tricks đ
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
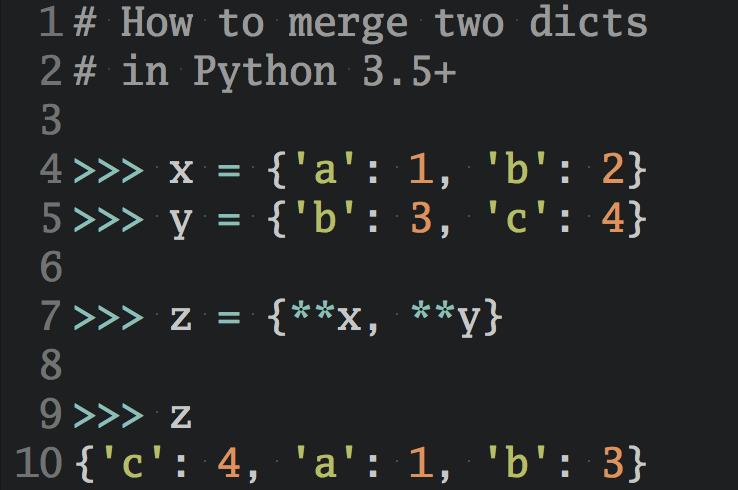
About John Sturtz
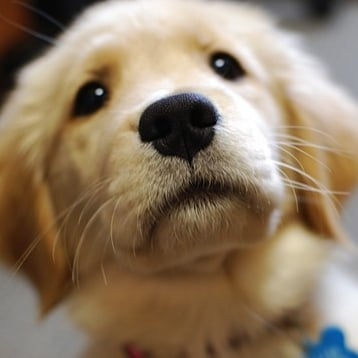
John is an avid Pythonista and a member of the Real Python tutorial team.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
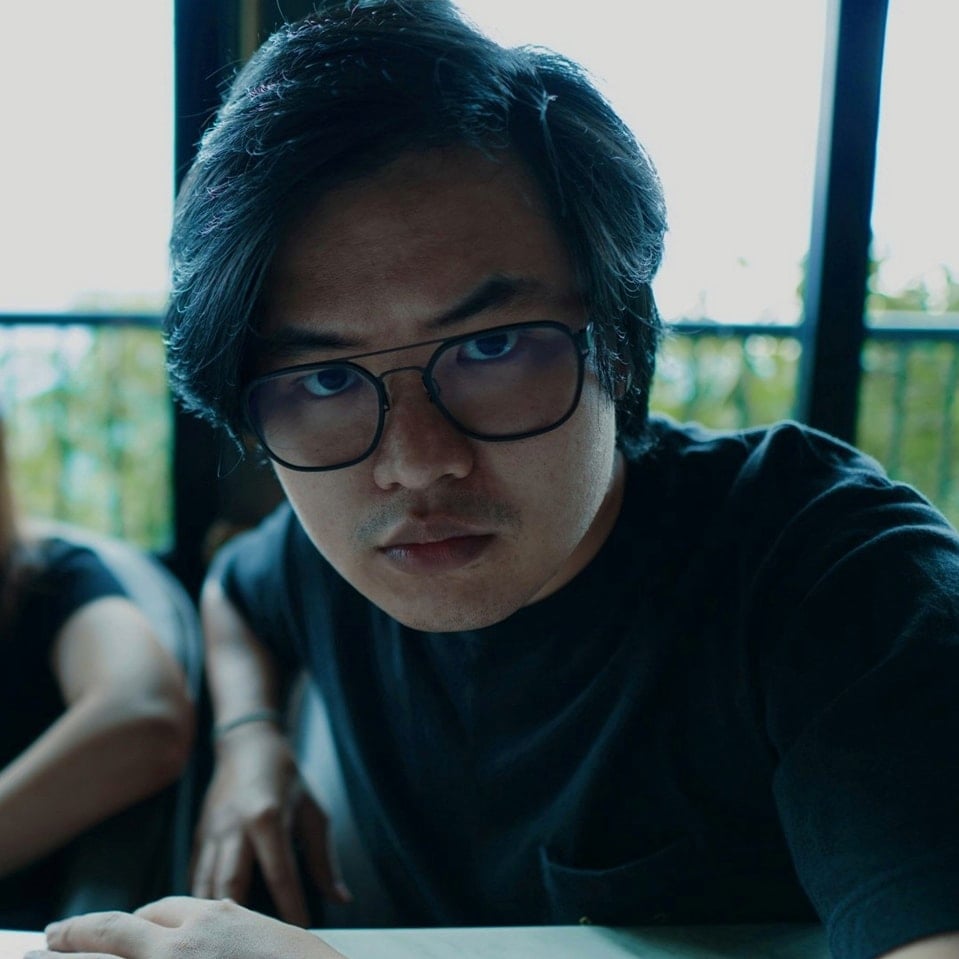
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
Whatâs your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics python
Recommended Video Course: Conditional Statements in Python (if/elif/else)
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In

Verilog Conditional Operator
Just what the heck is that question mark doing.
Have you ever come across a strange looking piece of Verilog code that has a question mark in the middle of it? A question mark in the middle of a line of code looks so bizarre; they’re supposed to go at the end of sentences! However in Verilog the ? operator is a very useful one, but it does take a bit of getting used to.
The question mark is known in Verilog as a conditional operator though in other programming languages it also is referred to as a ternary operator , an inline if , or a ternary if . It is used as a short-hand way to write a conditional expression in Verilog (rather than using if/else statements). Let’s look at how it is used:
Here, condition is the check that the code is performing. This condition might be things like, “Is the value in A greater than the value in B?” or “Is A=1?”. Depending on if this condition evaluates to true, the first expression is chosen. If the condition evaluates to false, the part after the colon is chosen. I wrote an example of this. The code below is really elegant stuff. The way I look at the question mark operator is I say to myself, “Tell me about the value in r_Check. If it’s true, then return “HI THERE” if it’s false, then return “POTATO”. You can also use the conditional operator to assign signals , as shown with the signal w_Test1 in the example below. Assigning signals with the conditional operator is useful!
Nested Conditional Operators
There are examples in which it might be useful to combine two or more conditional operators in a single assignment. Consider the truth table below. The truth table shows a 2-input truth table. You need to know the value of both r_Sel[1] and r_Sel[0] to determine the value of the output w_Out. This could be achieved with a bunch of if-else if-else if combinations, or a case statement, but it’s much cleaner and simpler to use the conditional operator to achieve the same goal.
r_Sel[1] | r_Sel[0] | Output w_Out |
0 | 0 | 1 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Learn Verilog
One Comment
The input “r_Sel” must be reg type? Could it be wire type?
Leave A Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Python One Line Conditional Assignment
Problem : How to perform one-line if conditional assignments in Python?
Example : Say, you start with the following code.
You want to set the value of x to 42 if boo is True , and do nothing otherwise.
Let’s dive into the different ways to accomplish this in Python. We start with an overview:
Exercise : Run the code. Are all outputs the same?
Next, you’ll dive into each of those methods and boost your one-liner superpower !
Method 1: Ternary Operator
The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True . Otherwise, if the expression c evaluates to False , the ternary operator returns the alternative expression y .
Operand | Description |
---|---|
<OnTrue> | The return expression of the operator in case the condition evaluates to |
<Condition> | The condition that determines whether to return the <On True> or the <On False> branch. |
<OnFalse> | The return expression of the operator in case the condition evaluates to |
Let’s go back to our example problem! You want to set the value of x to 42 if boo is True , and do nothing otherwise. Here’s how to do this in a single line:
While using the ternary operator works, you may wonder whether it’s possible to avoid the ...else x part for clarity of the code? In the next method, you’ll learn how!
If you need to improve your understanding of the ternary operator, watch the following video:
You can also read the related article:
- Python One Line Ternary
Method 2: Single-Line If Statement
Like in the previous method, you want to set the value of x to 42 if boo is True , and do nothing otherwise. But you don’t want to have a redundant else branch. How to do this in Python?
The solution to skip the else part of the ternary operator is surprisingly simple— use a standard if statement without else branch and write it into a single line of code :
To learn more about what you can pack into a single line, watch my tutorial video “If-Then-Else in One Line Python” :
Method 3: Ternary Tuple Syntax Hack
A shorthand form of the ternary operator is the following tuple syntax .
Syntax : You can use the tuple syntax (x, y)[c] consisting of a tuple (x, y) and a condition c enclosed in a square bracket. Here’s a more intuitive way to represent this tuple syntax.
In fact, the order of the <OnFalse> and <OnTrue> operands is just flipped when compared to the basic ternary operator. First, you have the branch that’s returned if the condition does NOT hold. Second, you run the branch that’s returned if the condition holds.
Clever! The condition boo holds so the return value passed into the x variable is the <OnTrue> branch 42 .
Don’t worry if this confuses you—you’re not alone. You can clarify the tuple syntax once and for all by studying my detailed blog article.
Related Article : Python Ternary — Tuple Syntax Hack
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
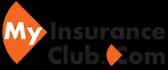
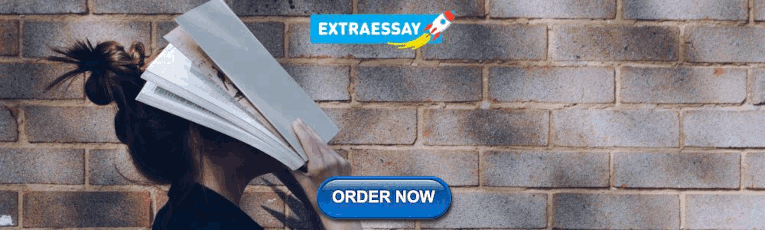
Conditional Assignment of a Life Insurance Policy
Conditional Assignment means that the Transfer of Rights will happen from the Assignor to the Assignee subject to certain terms and conditions. If the conditions are fulfilled then only the Policy will get transferred from the Assignor to the Assignee.
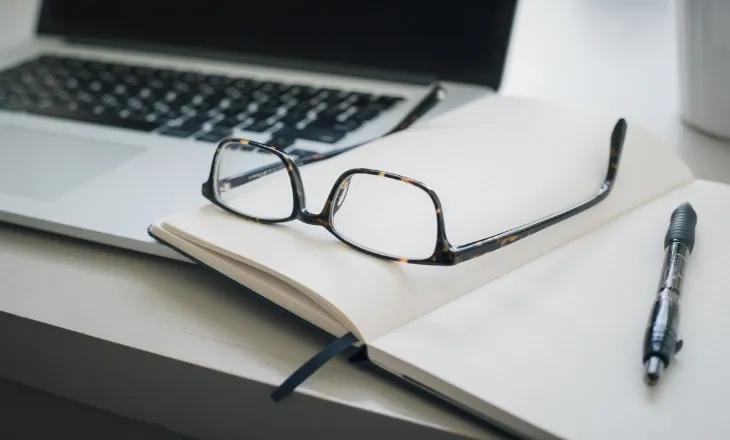
The process of transferring rights of a Life Insurance Policy is called Assignment. There are 2 types of Assignment:
- Absolute Assignment
- Conditional Assignment
Conditional Assignment means that the Transfer of Rights will happen from the Assignor to the Assignee subject to certain terms and conditions. If the conditions are fulfilled then only the Policy will get transferred from the Assignor to the Assignee. Or the policy will get transferred from the Assignor to the Assignee till certain conditions are fulfilled. Once the conditions are fulfilled, the policy automatically gets transferred back to the original owner, i.e. the Assignor.
Letâs take an example:
Rahul owns a Life Insurance Policy of Rs 5 lakhs. He needs to take a loan for his daughterâs school admission. He thought of doing so by taking a loan from the insurer itself or any bank against his Life Insurance Policy of Rs 5 lakhs that he owned.
However, to take a loan from the insurer itself or any bank, he needed to transfer the rights of the Insurance Policy to that entity. Thus he would have had to perform Conditional Assignment of the policy to that Bank. Then the bank would be able to pay out the loan money to him by taking the Insurance Policy as collateral. Thus, if Rahul failed to repay the loan, then the bank would surrender the policy and recover their money.
Once Rahulâs loan is completely repaid, then the policy would automatically come back to Rahul. In case, Rahul died before completely repaying the loan, then also the bank can surrender the policy to get their money back. This type of Assignment is called Conditional Assignment.
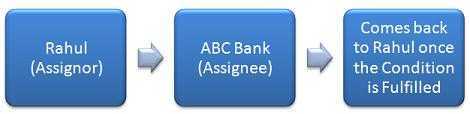
Example in real life of Conditional Assignment happens in case of an Insurance Policy being taken by the employer as a perquisite for the employee and it would be given only if he stays with the company for at least 5 years. Then the policy would be purchased by the employer on the employeeâs name, but it would get transferred to him only when he completes 5 years. Once the employee completes 5 years of service, the policy gets transferred to him. This type of assignment or transfer of rights of a Life Insurance Policy is called Conditional Assignment.

Sachin Telawane is a Content Manager and writes on various aspects of the Insurance industry. His enlightening insights on the insurance industry has guided the readers to make informed decisions in the course of purchasing insurance plans.

Conditional expression (ternary operator) in Python
Python has a conditional expression (sometimes called a "ternary operator"). You can write operations like if statements in one line with conditional expressions.
- 6. Expressions - Conditional expressions â Python 3.11.3 documentation
Basics of the conditional expression (ternary operator)
If ... elif ... else ... by conditional expressions, list comprehensions and conditional expressions, lambda expressions and conditional expressions.
See the following article for if statements in Python.
- Python if statements (if, elif, else)
In Python, the conditional expression is written as follows.
The condition is evaluated first. If condition is True , X is evaluated and its value is returned, and if condition is False , Y is evaluated and its value is returned.
If you want to switch the value based on a condition, simply use the desired values in the conditional expression.
If you want to switch between operations based on a condition, simply describe each corresponding expression in the conditional expression.
An expression that does not return a value (i.e., an expression that returns None ) is also acceptable in a conditional expression. Depending on the condition, either expression will be evaluated and executed.
The above example is equivalent to the following code written with an if statement.
You can also combine multiple conditions using logical operators such as and or or .
- Boolean operators in Python (and, or, not)
By combining conditional expressions, you can write an operation like if ... elif ... else ... in one line.
However, it is difficult to understand, so it may be better not to use it often.
The following two interpretations are possible, but the expression is processed as the first one.
In the sample code below, which includes three expressions, the first expression is interpreted like the second, rather than the third:
By using conditional expressions in list comprehensions, you can apply operations to the elements of the list based on the condition.
See the following article for details on list comprehensions.
- List comprehensions in Python
Conditional expressions are also useful when you want to apply an operation similar to an if statement within lambda expressions.
In the example above, the lambda expression is assigned to a variable for convenience, but this is not recommended by PEP8.
Refer to the following article for more details on lambda expressions.
- Lambda expressions in Python
Related Categories
Related articles.
- Shallow and deep copy in Python: copy(), deepcopy()
- Composite two images according to a mask image with Python, Pillow
- OpenCV, NumPy: Rotate and flip image
- pandas: Check if DataFrame/Series is empty
- Check pandas version: pd.show_versions
- Python if statement (if, elif, else)
- pandas: Find the quantile with quantile()
- Handle date and time with the datetime module in Python
- Get image size (width, height) with Python, OpenCV, Pillow (PIL)
- Convert between Unix time (Epoch time) and datetime in Python
- Convert BGR and RGB with Python, OpenCV (cvtColor)
- Matrix operations with NumPy in Python
- pandas: Replace values in DataFrame and Series with replace()
- Uppercase and lowercase strings in Python (conversion and checking)
- Calculate mean, median, mode, variance, standard deviation in Python
{{ activeMenu.name }}
- Python Courses
- JavaScript Courses
- Artificial Intelligence Courses
- Data Science Courses
- React Courses
- Ethical Hacking Courses
- View All Courses
Fresh Articles
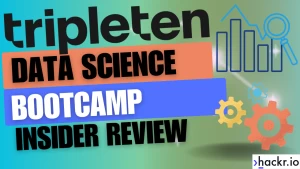
- Python Projects
- JavaScript Projects
- Java Projects
- HTML Projects
- C++ Projects
- PHP Projects
- View All Projects
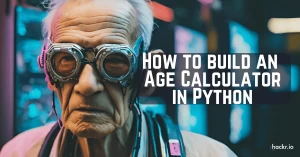
- Python Certifications
- JavaScript Certifications
- Linux Certifications
- Data Science Certifications
- Data Analytics Certifications
- Cybersecurity Certifications
- View All Certifications
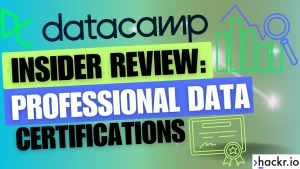
- IDEs & Editors
- Web Development
- Frameworks & Libraries
- View All Programming
- View All Development
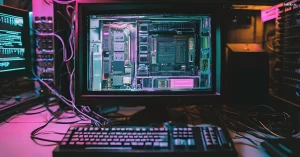
- App Development
- Game Development
- Courses, Books, & Certifications
- Data Science
- Data Analytics
- Artificial Intelligence (AI)
- Machine Learning (ML)
- View All Data, Analysis, & AI
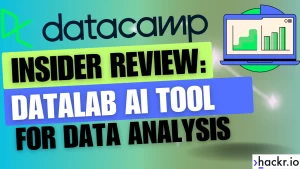
- Networking & Security
- Cloud, DevOps, & Systems
- Recommendations
- Crypto, Web3, & Blockchain
- User-Submitted Tutorials
- View All Blog Content
- Python Online Compiler
- JavaScript Online Compiler
- HTML & CSS Online Compiler
- Certifications
- Programming
- Development
- Data, Analysis, & AI
- Online Python Compiler
- Online JavaScript Compiler
- Online HTML Compiler
Don't have an account? Sign up
Forgot your password?
Already have an account? Login
Have you read our submission guidelines?
Go back to Sign In
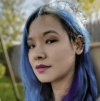
Python Ternary Operator: How and Why You Should Use It
Writing concise, practical, organized, and intelligible code should be a top priority for any Python developer. Enter, the Python ternary operator.
What is this? I hear you ask. Well, you can use the ternary operator to test a condition and then execute a conditional assignment with only one line of code. And why is this so great? Well, this means we can replace those ever-popular if-else statements with a quicker and more practical way to code conditional assignments.
Now donât get me wrong, we still need if-else statements (check out our comprehensive guide and Python cheat sheet for the best way to use conditional statements). But, itâs always good practice to make your code more Pythonic when thereâs a simpler way to get the same result, and thatâs exactly what you get with the ternary operator.
- Why Use the Python Ternary Operator?
Ternary operators (also known as conditional operators) in Python perform evaluations and assignments after checking whether a given condition is true or false. This means that the ternary operator is a bit like a simplified, one-line if-else statement. Cool, right?
When used correctly, this operator reduces code size and improves readability .
Ternary Operator Syntax
A ternary operator takes three operands:
- condition: A Boolean expression that evaluates to true or false
- true_value: A value or expression to be assigned if the condition evaluates to true
- false_value: A value or expression to be assigned if the condition evaluates to false
This is how it should look when we put it all together:
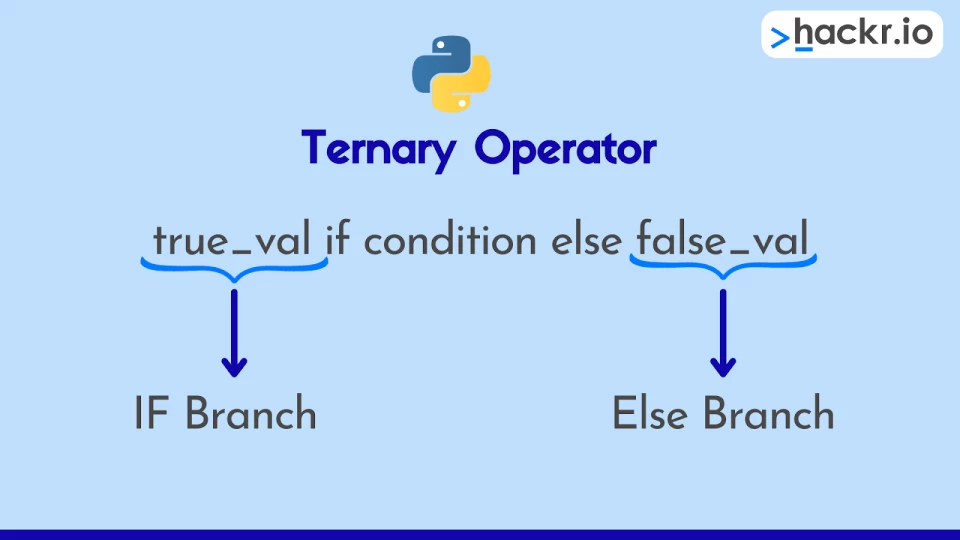
So, this means that the variable "var" on the left side of the assignment operator (=), will be assigned either:
- true_value if the Boolean expression evaluates to true
- false_value if the Boolean expression evaluates to false
- How to Use the Ternary Operator Instead of If-Else
To better understand how we replace an if-else statement with the ternary operator in Python, letâs write a simple program with each approach. In this first example, weâll assume the user enters an integer number when prompted, and weâll start with the familiar if-else.
Program Using an If-Else Statement
Hereâs what we get when the user enters 22 at the input prompt:
In this example, the if-else statement assigns âYes, you are old enough to watch this movie!â to the movie_acess variable if the user enters an age that is greater than or equal to 18, otherwise, it assigns "Sorry, you aren't old enough to watch this movie yet!â. Finally, we use an f-string to print out the result to the screen.
Now, let's use the ternary operator syntax to make the program much more concise.
Program Using a Ternary Operator
In this version of the program, the variable that receives the result of the conditional statement (movie_access) is to the left of the assignment operator (=).
The ternary operator evaluates the condition:
If the condition evaluates to true, the program assigns âYes, you are old enough to watch this movie!â to movie_access, else it assigns âSorry, you aren't old enough to watch this movie yet!â.
Letâs take a look at another example that uses the Python ternary operator and if-else to achieve the same goal. This time, weâll write a simple Python code snippet to check whether a given integer is even or odd.
In this program, weâve used a ternary operator and an if-else statement block to determine whether the given integer produces a zero remainder when using modulo divide by 2 (if youâre unfamiliar with this operator, take a look at our cheat sheet). If the remainder is zero, we have an even number, otherwise, we have an odd number.
Right away, we can tell what the if-else statement will do after evaluating the condition. With the ternary operator snippet, we can see the following:
- "Even" is assigned to msg if the condition (given_int % 2 == 0) is true
- "Odd" is assigned to msg if the condition (given_int % 2 == 0) is false
And, as weâve set the given integer to an even number, the program will print âEvenâ to the screen.
So, we can see again that a ternary conditional statement is a much cleaner and more concise way to achieve the same outcome as an if-else statement. We simply evaluate the ternary expression from left to right, then assign the return value for the true or false condition.
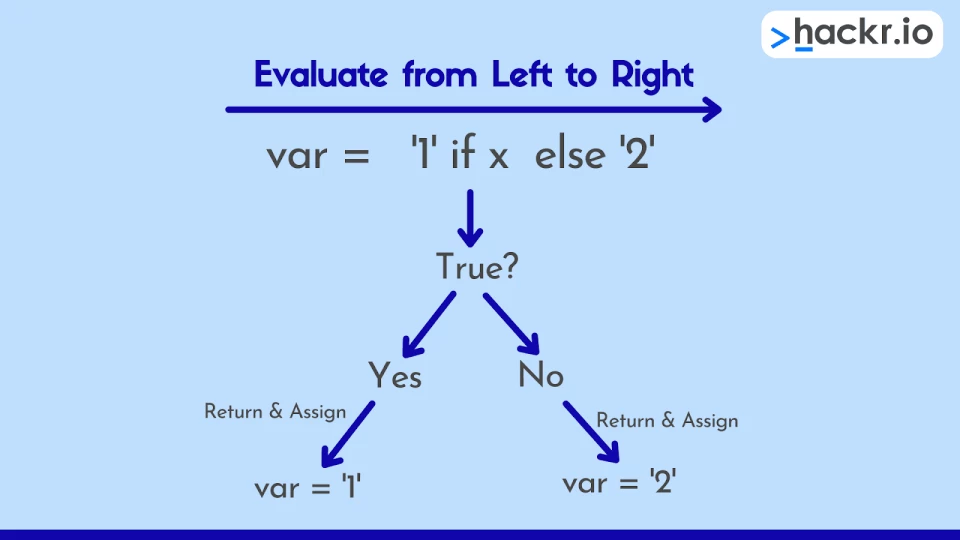
- Key Considerations for Python Ternary Statements
The ternary operator is not always suitable to replace if-else in your code.
If for example, we have more than two conditional branches with an if-elif-else statement, we canât replace this with a single ternary operator. The clue here is in the name, as ternary refers to the number âthreeâ, meaning that it expects three operands. But, if we try to replace an if-elif-else statement with a ternary operator, weâll have four operands to handle (or possibly more if we have many âelifâ branches).
So what do we do here? Well, we need to chain together (or nest) multiple ternary operators (we cover this in the FAQs), but sometimes this can get a little messy, and it may be cleaner to use if-elif-else if the code becomes difficult to read.
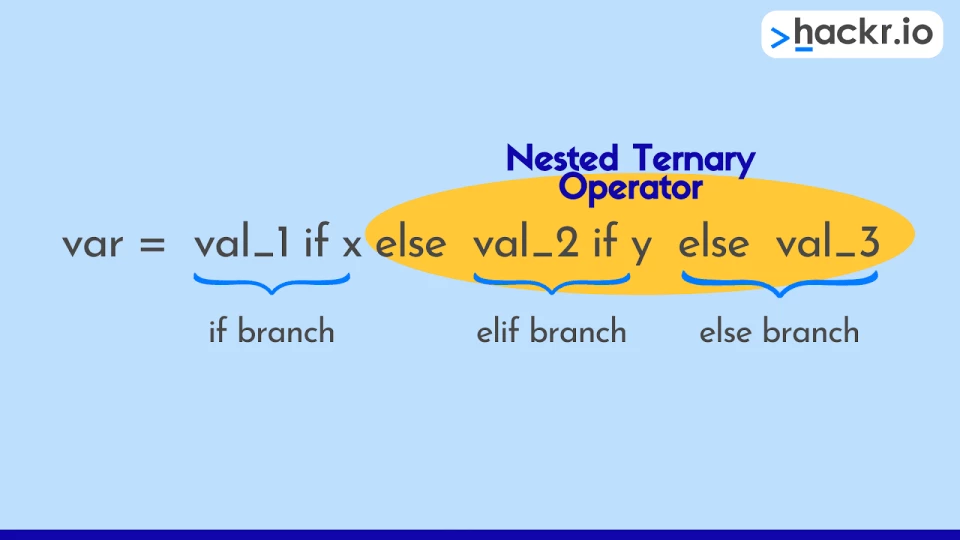
Another thing to keep in mind is that weâre meant to use the ternary operator for conditional assignment. What does this mean? Well, we canât use the ternary approach if we want to execute blocks of code after evaluating a conditional expression: in these situations, itâs best to stick with if-else.
But, we can use the ternary operator if we are assigning something to a variable after checking against a condition. See, itâs easy if we remember that weâre supposed to be assigning something to something else.
Distinct Features of Python Ternary Statements
- Returns A or B depending on the Boolean result of the conditional expression (a < b)
- Compared with C-type languages(C/C++), it uses a different ordering of the provided arguments
- Conditional expressions have the lowest priority of all Python operations
That's all. We did our best to fill you in on everything there is to know about the Python ternary operator. We also covered how to use ternary statements in your Python code, and we compared them to their close relatives, the ever-popular if-else statement.
If youâre a beginner thatâs looking for an easy start to your Python programming career, check out our learning guide along with our up-to-date list of Python courses where you can easily enroll online.
Lastly, donât forget that you can also check out our comprehensive Python cheat sheet which covers various topics, including Python basics, flow control, Modules in Python, functions, exception handling, lists, dictionaries, and data structures, sets, the itertools module, comprehensions, lambda functions, string formatting, the ternary conditional operator, and much more.
- Frequently Asked Questions
1. What are Ternary Operators (including an example)?
Programmers like to use the concise ternary operator for conditional assignments instead of lengthy if-else statements.
The ternary operator takes three arguments:
- Firstly, the comparison argument
- Secondly, the value (or result of an expression) to assign if the comparison is true
- Thirdly, the value (or result of an expression) to assign if the comparison is false
Simple Ternary Operator Example:
If we run this simple program, the "(m < n)" condition evaluates to true, which means that "m" is assigned to "o" and the print statement outputs the integer 10.
The conditional return values of "m" and "n" must not be whole statements, but rather simple values or the result of an expression.
2. How Do You Write a 3-Condition Ternary Operator?
Say you have three conditions to check against and you want to use a ternary conditional statement. What do you do? The answer is actually pretty simple, chain together multiple ternary operators. The syntax below shows the general format for this:
If we break this syntax down, it is saying:
- If condition_1 is true, return value_1 and assign this to var
- Else, check if condition_2 is true. If it is, return value_2 and assign it to var
- If neither conditions are true, return value_3 and assign this to var
Weâve now created a one-line version of an if-elif-else statement using a chain of ternary operators.
The equivalent if-elif-else statement would be:
One thing to consider before chaining together ternary statements is whether it makes the code more difficult to read. If so, then itâs probably not very Pythonic and youâd be better off with an if-elif-else statement.
3. How Do You Code a Ternary Operator Statement?
We can easily write a ternary expression in Python if we follow the general form shown below:
So, we start by choosing a condition to evaluate against. Then, we either return the true_value or the false_value and then we assign this to results_var .
4. Is the Ternary Operator Faster Than If-Else?
If we consider that a ternary operator is a single-line statement and an if-else statement is a block of code, then it makes sense that if-else will take longer to complete, which means that yes, the ternary operator is faster.
But, if we think about speed in terms of Big-O notation (if Iâve lost you here, donât worry, head on over to our Big-O cheat sheet ), then they are in fact equally fast. How can this be? This is because conditional statements are viewed as constant time operations when the size of our problem grows very large.
So, the answer is both yes and no depending on the type of question youâre asking.
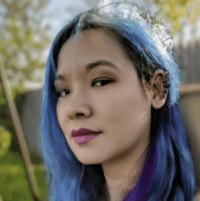
Jenna Inouye currently works at Google and has been a full-stack developer for two decades, specializing in web application design and development. She is a tech expert with a B.S. in Information & Computer Science and MCITP certification. For the last eight years, she has worked as a news and feature writer focusing on technology and finance, with bylines in Udemy, SVG, The Gamer, Productivity Spot, and Spreadsheet Point.
Subscribe to our Newsletter for Articles, News, & Jobs.
Disclosure: Hackr.io is supported by its audience. When you purchase through links on our site, we may earn an affiliate commission.
In this article
- 10 Vital Python Concepts for Data Science
- 10 Common Python Mistakes in 2024 | Are You Making Them? Python Data Science Programming Skills
- GitHub Copilot vs Amazon CodeWhisperer | Who's Best in 2024? Artificial Intelligence (AI) Code Editors IDEs AI Tools
Please login to leave comments
Always be in the loop.
Get news once a week, and don't worry â no spam.
{{ errors }}
{{ message }}
- Help center
- We â€ïž Feedback
- Advertise / Partner
- Write for us
- Privacy Policy
- Cookie Policy
- Change Privacy Settings
- Disclosure Policy
- Terms and Conditions
- Refund Policy
Disclosure: This page may contain affliate links, meaning when you click the links and make a purchase, we receive a commission.
Thank you for visiting nature.com. You are using a browser version with limited support for CSS. To obtain the best experience, we recommend you use a more up to date browser (or turn off compatibility mode in Internet Explorer). In the meantime, to ensure continued support, we are displaying the site without styles and JavaScript.
- View all journals
- Explore content
- About the journal
- Publish with us
- Sign up for alerts
- Open access
- Published: 21 July 2024
UNet-like network fused swin transformer and CNN for semantic image synthesis
- Aihua Ke 1 , 2 ,
- Jian Luo 1 , 2 &
- Bo Cai 1 , 2 Â
Scientific Reports volume  14 , Article number: 16761 ( 2024 ) Cite this article
539 Accesses
Metrics details
- Computer science
- Mathematics and computing
Semantic image synthesis approaches has been dominated by the modelling of Convolutional Neural Networks (CNN). Due to the limitations of local perception, their performance improvement seems to have plateaued in recent years. To tackle this issue, we propose the SC-UNet model, which is a UNet-like network fused Swin Transformer and CNN for semantic image synthesis. Photorealistic image synthesis conditional on the given semantic layout depends on the high-level semantics and the low-level positions. To improve the synthesis performance, we design a novel conditional residual fusion module for the model decoder to efficiently fuse the hierarchical feature maps extracted at different scales. Moreover, this module combines the opposition-based learning mechanism and the weight assignment mechanism for enhancing and attending the semantic information. Compared to pure CNN-based models, our SC-UNet combines the local and global perceptions to better extract high- and low-level features and better fuse multi-scale features. We have conducted an extensive amount of comparison experiments, both in quantitative and qualitative terms, to validate the effectiveness of our proposed SC-UNet model for semantic image synthesis. The outcomes illustrate that SC-UNet distinctively outperforms the state-of-the-art model on three benchmark datasets (Citysacpes, ADE20K, and COCO-Stuff) including numerous real-scene images.
Similar content being viewed by others
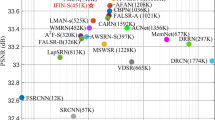
Lightweight interactive feature inference network for single-image super-resolution
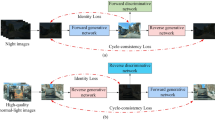
Nighttime road scene image enhancement based on cycle-consistent generative adversarial network

Multi-attention fusion transformer for single-image super-resolution
Introduction.
Semantic layout is a label map obtained using segmentation techniques for the semantic understanding of real-scene images. Following the rapid development of semantic segmentation techniques on the field of computer vision, it has become easier to acquire semantic layout maps, which has led to higher research attention on semantic layout maps. The research tasks based on semantic layout mainly incorporate semantic image retrieval 1 , 2 , semantic image segmentation 3 , 4 , 5 , semantic image synthesis 6 , 7 , 8 , semantic image classification 9 and semantic image annotation 10 , 11 . Semantic image synthesis is a special form of conditional image synthesis task, which aims to synthesize photo-realistic and well-aligned image conditioned on a given semantic layout. Semantic image synthesis has a wide range of practical applications, e.g., it allows ordinary users to control the scene image synthesis by simply modifying the semantic layout. In addition, it can be utilized as a data augmentation tool for deep model convergence by learning to synthesize fresh samples similar to the original image data.

Visual comparison of the synthesized images produced by our method and other baseline approaches. Key differences are positioned with boxes on the synthesized image, and shown magnified below image. âHist-stdâ indicates the histogramâs standard deviation, where lower values indicate more balanced colors in the synthesized image.
Currently, pure CNN-based models have been the mainstream alternative for addressing the task of semantic image synthesis. CNN is proficient at exploiting the nature of local perception and weight sharing, which enables to extract local features of complex scene images at a low level and to reduce the training difficulty. Despite its many advantages, CNN is not fully exploited in semantic image synthesis. Since SwinT 12 introduced the Swin Transformer to computer vision, it has achieved remarkable results in a wide range of downstream vision tasks, outperforming CNN in some cases. SwinT employs hierarchical vision transformers with shift windows to construct a backbone network, which can achieve a substantial improvement in synthesis performance on public datasets based on real scenes. zhang et al. 13 extended the application of SwinT to image synthesis by proposing Styleswin. In order to actualise a broader receptive field, Styleswin utilizes the SwinT architecture to construct a hierarchical feature mapping relation. Despite the excellent success of Styleswin in modelling high-resolution image synthesis,it still suffers from some limitations in image synthesis tasks conditional on semantic layout: (1) The low-level features with the fine-grained information from the semantic layout are insufficiently utilized. (2) Overly weight-light decoder cannot efficiently fuse hierarchical semantic features at different scales.
To tackle the above limitations, this paper proposes the SC-UNet model, which is a UNet-like network fused Swin Transformer and CNN for semantic image synthesis. Our SC-UNet model adopts a UNet-like network structure composed of an encoder and a decoder, which can effectively extract and fuse the fine-grained features at the low level and the coarse-grained features at the high level, so as to achieve the higher synthesis performance. For the encoder part, we utilize a succession of hierarchical Swin Transformers with shift window as the basic unit of the network. The Swin Transformer-based encoder has the capability to efficiently extract low-level feature information at different scales from the input semantic layout map. To fully utilize the low-level features with fine-grained information, we introduce the skip connection before down-sampling with the patch merging layer to fuse the low-level positional information with the high-level semantic information. For the decoder part, the conventional linear projection or \(1\times 1\) convolution can not sufficiently fuse the high- and low-level feature maps at different scales. Accordingly, we design a novel Conditional Residual Fusion (CRF) block, which as the classical structure of CNN can partially improve the synthesis performance. Moreover, the CRF block embeds an opposition-based learning mechanism and a weight assignment mechanism in the normalisation layer and the shortcut connection, respectively. Specifically, the opposition-based learning mechanism can efficiently augment semantic feature information through opposition-based learning, while the weight assignment mechanism can dynamically assign attentional weights over the channel and spatial dimensions.
Since our proposed SC-UNet method employs a supervised learning manner based on Generative Adversarial Networks (GAN), and utilizes the pre-trained Swin Transformer model to initialise the partial weights of the network. Therefore, the GAN-based SC-UNet approach reduces the occurrence probability of gradient disappearance or exploration owing to irrational initialisation. Figure 1 shows the visual comparison between the street scene images synthesised by our method and other baseline methods. From the figure, it can be clearly observed that our proposed SC-UNet method can synthesize more photo-realistic scene images by effectively mitigating common issues encountered in previous synthesis methods, such as local artifacts, color imbalance, and boundary ambiguities. Extensive experiment results, both qualitative and quantitative, conclude that the proposed SC-UNet method remarkably improves the performance of semantic image synthesis on three massive benchmark datasets, namely Cityscapes 14 , ADE20K 15 , and COCO-Stuff 16 . To enhance the robustness of our method, we sequentially resize the resolution of the three benchmark datasets to \(256 \times 512\) , \(256 \times 256\) and \(256 \times 256\) .
The following are the contributions of our paper overall:
We propose a UNet-like network model based on Swin Transformer and CNN for semantic image synthesis, which which overperforms the pure CNN-based model in effectively extracting high- and low-level features at different scales.
We propose a decoder based on the Conditional Residual Fusion (CRF) block, which can produce more accurate feature representations through the hierarchical fusion of multi-scale features to improve the synthesis performance.
We propose two novel mechanisms embedded in the CRF block, the opposition-based learning mechanism can effectively enhance the semantic feature information, while the weight assignment mechanism can dynamically assign attentional weights in channel and spatial dimensions.
Extensive experiments are undertaken on three public datasets: Citysacpes, ADE20K and COCO-Stuff.The results prove the effectiveness of our semantic image synthesis method and the state of the art performance is achieved.

Overview of our approach SC-UNet.
Related work
Generative adversarial networks.
Generative adversarial networks (GANs) 17 , 18 have become the mainstream method for image synthesis tasks. GAN architecture is usually composed of two main networks, namely the generator and the discriminator. The generator is in charge of synthesizing the target images using the given input conditions. Nevertheless, the discriminator aims to distinguish between the synthetic image and the matched natural image. The input conditions used by GAN-based image synthesis methods are various, such as sparse sketches 19 , 20 , 21 , gaussian noise 22 , 23 , text descriptions 24 , 25 , 26 , natural images 27 , 28 , and semantic layout 29 , 30 , 31 , 32 . Considering the great success of GANs in image synthesis, we propose a novel GAN-based approach to tackle image synthesis conditioned only on semantic layout.
Semantic image synthesis
Semantic image synthesis aims at generating high-fidelity image from the given semantic layout map. Such as, Pix2pix 33 proposes a general-purpose solution for image-to-image translation problems by investigating conditional adversarial networks. Then, Pix2pixHD 30 enhances it to achieve higher-resolution image synthesis from semantic label maps. Further, the normalization layer activations in GauGAN 22 are modulated by affine transformations using the input semantic layout. Based on the improvement of this idea, CC-FPSE 34 utilizes a semantic layout map as a condition to dynamically produce convolutional kernels. Similarly, both our approach and CC-FPSE are GAN-based semantic image synthesis models comprising generators and discriminators, utilizing features extracted from the semantic layout map to adaptively control the generation process. OASIS 32 enhances the generator supervision by using feedback from spatial and semantic perceptual discriminators, thus eliminating the limitations of vgg-based perceptual loss on the above GAN model. Besides GAN models, CRN 29 generates images with a photographic appearance that matches well with the input semantic layouts, and SIMS 35 presents a semi-parametric approach to deal with the semantic image synthesis task by integrating the complementing benefits of parametric and non-parametric techniques. However, there is still opportunity for improvement in the quality of images generated using the previous state-of-the-art model. Therefore, we need to propose a novel method for more efficiently extracting the important information contained in a given semantic layout that may promote the development of semantic image synthesis.
Conventional residual block
The conventional residual block, as a classical structure of convolutional neural network, has been extensively studied in prior research 36 . It typically consists of two convolutional layers and a shortcut connection, allowing for efficient transfer of input features to output features, thus facilitating cross-layer feature fusion. Additionally, the residual block helps mitigate the vanishing gradient problem and enhances network training capabilities. Kaiming et al. 36 introduced a residual learning framework to train deeper networks effectively, paving the way for subsequent advancements. Ruofan et al. 37 proposed a deep residual network for end-to-end projection learning, demonstrating its applicability in tasks involving Bayer images and high-resolution images. Despite the achievements of conventional residual blocks, they still exhibit limitations in image synthesis tasks conditioned on semantic layout maps. Recognizing this, we aim to augment traditional residual blocks by incorporating mechanisms inspired by Opposition-based Learning Mechanism (OLM) and Weight Assignment Mechanism (WAM). Where OLM is derived from the concept of opposition 38 , which aims to enhance learning by considering both positive and negative aspects of semantic features. This technique is employed to augment semantic information, thereby enhancing normalization performance. On the other hand, WAM can dynamically allocate attention weights on both channel and spatial dimensions. Despite previous research on similar mechanisms 39 , 40 , 41 such as channel attention and spatial attention, WAM demonstrates uniqueness by integrating feature weighting, thereby showcasing innovation in image synthesis tasks. By integrating these components, we seek to enhance feature fusion and pay closer attention to semantic feature information, ultimately improving fusion performance in image synthesis tasks.
SC-UNet is a semantic image synthesis model based on a UNet-like network structure composed of an encoder and a decoder. The overall architecture of the SC-UNet model is shown in Fig. 2 . In the encoding stage, the input semantic layout map is first semantically augmented by one-hot encoding operation and candy edge extraction operation, and then the augmented semantic features perform a patch embedding layer to obtain a sequence embedding for the input of the Swin Transformer module. Finally, the encoder based on Swin Transformer will extract the low-level features at different scales from the input sequence embedding. In the decoding stage, the decoder combined with the Conditional Residual Fusion (CRF) block and Swin Transformer module will hierarchically fuse the high-level semantic features with the low-level positional features. To recover the photo-realistic synthesized image with abundant details, our model finally employs a tanh activation function on the decoderâs output to maintain the pixel values within a specified range. Since our SC-UNet model utilizes a supervised training strategy based on Generative Adversarial Networks (GANs) and takes advantage of the pre-trained Swin Transformer module to initialize the partial weights of the network. Therefore, the GAN-based SC-UNet approach reduces the occurrence probability of exploding gradients owing to irrational initialisation. During the supervised training process, our model is optimised with the weighted summation of multiple loss functions, thus achieving better synthesis performance.
Model encoder
The encoder of our proposed SC-UNet method aims to extract the low-level position features under different dimensions from the input semantic layout map. Let \(M \in \mathbb {R}^{H \times W \times 1}\) be the input semantic layout map of the model, where H and W dimensions denote the height and width, respectively. To extract more accurate and comprehensive feature representations, the input semantic layout map first needs to be semantically augmented by simultaneously performing a one-hot encoding operation 15 and a candy edge extraction operation. Among them, the one-hot encoding operation can map each object class in the semantic layout map with discrete nature into different channels, thus acquiring a more effective multi-channel feature representation. And the candy edge extraction operation can quickly and accurately extracts the positional information of the object edges from the input semantic layout map M by conducting several steps such as Gaussian blurring, gradient computation, non-maximum value suppression, double threshold detection and edge tracking, thus acquiring a feature representation for further processing by the encoder. Two feature representations resulted by augmenting the semantics are fused into a fresh feature representation \(F_s \in \mathbb {R}^{H \times W \times N_{cls+1}}\) by the channel concatenation way. Where \(N_{cls+1}\) is equal to the total number of object classes in a given dataset plus 1. The new feature representation \(F_s\) is then fed to a patch embedding layer, thus obtaining a sequence embedding \(e_1 \in \mathbb {R}^{\frac{W}{4} \times \frac{H}{4} \times 96}\) as the input of the Swin Transformer module. More specifically, the patch embedding layer exploits non-overlapping convolution to partition the feature map \(F_s\) into a series of patch tokens with size 4, and then each patch token is flattened into a sequence embedding \(e_i\) by linear mapping. Compared to the default \(16\times 16\) patch setting, a smaller patch size facilitates to extract the local features containing more detailed information, but also leads to an extended computational workload. The above mapping process from an input semantic layout map to a one-dimensional sequence embedding is summarized as follows:
where Concat, Encoding and Candy denote the concatenation operation, the one-hot encoding operation and the candy edge extraction operation, respectively. And Conv and Linear are utilized to realize patch partition and linear mapping in the patch embedding layer.
The backbone network of the encoder comprises four combinations of the Swin Transformer module and the patch merging layer, which aims to hierarchically extract the fine-grained features at different scales. Specifically, the patch merging layer mainly focuses on downsampling the feature map to influence the dimensions of width, height and number of channels. And the Swin Transformer module mainly focuses on extracting the low-level position features. Each Swin Transformer module \(T_i\) is made of two consecutive Transformer blocks based on Layer Normalization (LN) 42 layer, Multi-head Self-Attention (MSA) layer, Feed-forward Network (FFN) layer, and skip connections, respectively. The two successive Transformer blocks have the same structure, but the MSA layer can be further subdivided into window-based MSA and shift-window-based MSA based on the window division scheme. In the \(T_i\) module, the detailed computation of the Transformer block is as follows:
here \(e_{i+1}\) represents the output of the Transformer block with a sequence embedding \(e_i\) as input. Multi-head Self-Attention (MSA) has three attention heads with parallel and independent computation, which can effectively shorten the computation workload. The specific computing for the MSA is illustrated as follows:
where \(\text {ESA}(Q,K,V)\) Multiple self-attention ESA represents the output from the integration of QKVâs attention. The symbols Q, K and V denote a query vector, a key vector and a value vector respectively. They are obtained by linear mapping of the same input vector \(\text {LN}(e_i)\) . And, \(d_{K}\) , B and T represent the scaled dot-product attention, bias vector and the transpose operation, respectively.
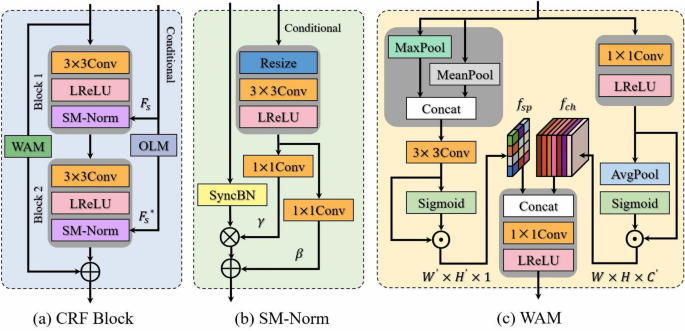
Structure of the CRF block in the SC-UNet method. ( a ) The CRF block represents the conditional residual fusion block. ( b ) SM-Norm denotes the normalization based on semantic modulation. ( c ) WAM stands for the weighting assignment mechanism.
Model decoder
The decoder will fuse the multi-scale features hierarchically extracted by the encoder from the input semantic layout map to recover a realistic synthesized image at the original resolution size. The hierarchical features at the smallest scale are first passed through a Conditional Residual Fusion (CRF) block based on CNN to obtain high-level features with more semantic information. In order to be concatenated with the low-level features output from the encoder in the channel direction, the obtained high-level features need to be up-sampled to \(2 \times\) resolution utilizing a patch expand layer. Subsequently, the fusion results obtained from the high- and low-level features using the concatenation operation performs a CRF block conditional on the feature representation \(F_s \in \mathbb {R}^{H \times W \times N_{cls+1}}\) before being fed to the Swin Transform module. Compared with CNN, a series of combining operations constructed with the Swin Transformer module as the backbone can better capture the contextual feature mapping, which incorporates the comprehensive information of the low-level positions and the high-level semantics. Finally, the feature mapping output by Swin Transformer module will pass through an Image Block to recover a naturalistic synthesized image with the \(H\times W\times 3\) dimensions. The image block, as the final layer of the decoder, is composed of two CRF blocks, a \(3\times 3\) convolution with a padding size of 1, an upsampling function, and a tanh activation function. The following is a detailed description of the CRF block in the decoder network.
Conditional residual fusion block
Earlier approaches for semantic image synthesis primarily specialized in the extraction of low-level features at multiple scales, while neglecting the information fusion among high- and low-level features. These methods exploit the concatenation in the number of channels to form the thicker features, which are then depended to recover the high quality synthesized image. Motivated by ResNet 36 , we design a Conditional Residual Fusion (CRF) block to achieve more effective information fusion between high- and low-level features at multiple scales. As shown in Fig. 3 a, the CRF block is composed of two successive convolutional blocks, a Opposition-based Learning Mechanism (OLM), and a Weight Assignment Mechanism (WAM).
For each convolutional block, our CRF block not only expands the single \(3\times 3\) convolution layer by adding a LReLU 22 activation function and a SM-Norm layer to effectively prevent network overfitting, but it also introduces novel mechanisms to enhance feature extraction. Where SM-Norm stands for the normalization based on semantic modulation, which can effectively improve the convergence speed by shortening the feature differences. Different from the Batch Normalization (BN) 43 , SM-Norm performs the normalization of input activation conditional on \(F_s \in \mathbb {R}^{H \times W \times N_{cls+1}}\) , and its structure is given in Fig. 3 b. The input activation \(h \in \mathbb {R}^{H \times W \times C}\) to the SM-Norm layer is first parameter-free normalized along the batch dimension exploiting the Synchronized Batch Normalization (SyncBN). Then, the condition \(F_s\) as another input of SM-Norm layer will perform a combined block of Resize-Conv-LReLU to extract the semantic features, and utilizes two \(1\times 1\) convolution layers to produce the normalization parameters \(\gamma \in \mathbb {R}^{H \times W \times C}\) and \(\beta \in \mathbb {R}^{H \times W \times C}\) , respectively. Finally, the produced \(\gamma\) and \(\beta\) are multiplied and added to the normalized activation in the element-wise way. Formally, the SM-Norm layer can be defined as:
where \(\mathbb {E}\left[ h \right]\) and \({\text {Var}}\left( h\right)\) represent the mean and standard deviation of the input activation h , respectively. And the symbol \(\varepsilon\) usually denotes a very small positive number. The \(\gamma\) and \(\beta\) learned from condition \(F_s\) are used to modulate the normalized activation in scale and bias.
In addition, the CRF block embeds two novel mechanisms, WAM and OLM, to enhance the hierarchical fusion of high- and low-level features. The WAM added on the constant shortcut connection can adaptively assign different attention weights for the input features, thus obtaining effective feature representations enhanced in both channel and spatial dimensions. Due to the sparse semantic information in condition \(F_s\) , the designed OLM is utilized to actualize the enhancement of semantic feature information.
Opposition-based learning mechanism
The condition \(F_s\) obtained from the input semantic layout map is used to positively influence the normalization layer of CRF block. Accordingly, it is necessary to augment the semantic information of the sparse \(F_s\) for the improvement of normalization performance. Computational intelligence employs opposition-based learning 38 , which has been demonstrated to be an efficient way to improve different optimization methods. To augment semantic information in the condition \(F_s\) , we suggest a fresh Opposition-based Learning Mechanism (OLM) for accomplishing the modulation of the normalization layer.
The condition \(F_s\) is gained by the channel concatenation of one-hot label M and edge map E . Since the semantic information of the condition \(F_s\) is mainly derived from the one-hot label M , which is the output of performing a one-hot encoding operation on the semantic layout map. Thus, the semantic augmentation result of condition \(F_s\) passing through an opposites-based learning mechanism can be expressed as follows:
where \(M^*\) denotes the opposition-based one-hot label. The central idea underlying opposition-based learning is that the opposing side of a solution is possibly closer to the optimal solution. Let \(M = \left\{ m_1 ,m_2 , ... , m_C \right\} ^{H\times W\times C}\) be the one-hot label with multi-channel feature maps. Where the symbols W , H , and C represent the width, height, and number of channels in a semantic condition, respectively. \(m_i \in \left\{ a,b\right\} ^{H\times W\times 1}\) denotes a feature map of the i th channel. Since each pixel value identifies the object class to which it belongs. In \(m_i\) , only the pixel value of the i th object class is 1, and the other pixels are 0. Referring to the description of the opposite point in opposition-based learning, the opposition-based one-hot label \(M^*\) is described as:
where, according to the definition of the one-hot label, the thresholds a and b are set to 0 and 1 respectively. \({m_i}^* \in \left\{ 0,1 \right\} ^{H\times W \times 1 }\) represents a feature map of the i th channel in the opposition-based one-hot label.
Weight assignment mechanism
The distribution of redundant information in input features is usually different. Therefore, we design a Weight Assignment Mechanism (WAM) embedded in the shortcut connections, which can adaptively assign different attention weights to the input features. The detailed structure of WAM is presented in Fig. 3 c. WAM first extracts important semantic and positional features to filter the redundant information in the input features. And then, the extracted important features are efficaciously fused to output a more powerful feature representation.
The extraction of important semantic features relies on the learning of semantic correlations on the channel dimensions. Since the sub-feature maps on each channel dimension contain different amounts of semantic information, assigning an attention weight to them can extract enhanced semantic features. The input feature \(f_{in} \in \mathbb {R}^{H\times W \times C}\) of WAM is first passed through a \(1\times 1\) convolution layer and an LReLU activation to produce the intermediate feature \(t_{ch} \in \mathbb {R}^{H\times W \times {C}'}\) . Then, \(t_{ch}\) utilizes an adaptive average pooling layer and a sigmoid activation function to obtain channel attention weights \(W_{ch} \in \mathbb {R}^{1\times 1\times {C}'}\) , which reflect the importance of the sub-feature maps on each channel dimension. Finally, \(t_{ch}\) and \(W_{ch}\) are fused using element-wise multiplication to extract the attended semantic features \(f_{sc} \in \mathbb {R}^{H\times W \times {C}'}\) . This description of the above process can be defined as:
where \(\odot\) denotes element-wise multiplication to fuse the feature information.
Extracting important positional features depends on learning to correlate positions in space. Similarly, each pixel in the spatial location is assigned an attention weight, which helps to extract the enhanced positional features. To learning the spatial relationship, the input feature \(f_{in} \in \mathbb {R}^{H\times W \times C}\) first combines the outcomes from the max pooling and average pooling layers in the channel concatenation way to produce a higher dimensional feature \(t_{sp} \in \mathbb {R}^{H\times W \times 2}\) . To reduce the number of channels, \(t_{sp}\) exploits a \(3\times 3\) convolution layer with padding size 1, resulting in the intermediate feature \({t^*}_{sp} \in \mathbb {R}^{H\times W \times 1}\) . After that, \({t^*}_{sp}\) utilizes a sigmoid activation function to get the spatial attention weights \(W_{sp} \in \mathbb {R}^{H\times W\times 1}\) , which reflect the importance of the position-wise pixel in the spatial dimension. Finally, we perform a matrix multiplication between \({t^*}_{sp}\) and \(W_{sp}\) to extract the attended positional features \(f_{sp} \in \mathbb {R}^{H\times W \times 1}\) . Mathematically,
The semantic and location features are fused using channel concatenation , and the convolution layer and LReLU activation function will be sequentially performed to generate the final output feature \(f_{out} \in \mathbb {R}^{H\times W \times {C}'}\) of the WAM.

Variation trend of the discriminator and generator loss values with number of iterations during training on the Cityscapes dataset. The black and blue curves indicate the results of total loss and its correlated loss in training, respectively.
Discriminator and loss function
Similar to GauGAN 22 , we use an efficient multi-scale discriminator, which will perform the adversarial training with our SC-UNet network (also regarded as a generator). The multi-scale discriminator utilizes the integration of multiple PatchGAN discriminators with the same structure, and the input image size is different for each PatchGAN 33 discriminator. To distinguish between synthesized and real images, the multi-scale discriminator first scales the input image into different sizes and feeds them into the corresponding PatchGAN discriminator. After that, the output matrices of all PatchGAN discriminators are calculated the mean value. Finally, the summation result of the mean value will be applied as the basis for true or false discrimination. In our experiments, the multi-scale discriminator actually uses only two PatchGAN discriminators, and the size of their input images is the original resolution and half of the original resolution, respectively. Table 1 shows the size change of an original resolution image after being fed to the PatchGAN discriminator. Where each PatchGAN discriminator consists of 6 convolution blocks, which is based on the convolution layer, the instance normalization 44 , and the LReLU activation function.
The multi-scale discriminator is optimized using only the hinge-based adversarial loss \(\mathscr {L}_{hadv}^{D}\) 45 to distinguish between synthesized and real images. However, the generator is optimized with the weighted sum of the multiple loss functions, which include hinge-based adversarial loss \(\mathscr {L}_{hadv}^G\) , feature matching loss \(\mathscr {L}_{fm}\) 30 , and perceptual loss \(\mathscr {L}_{vgg}\) 30 . Finally, all the above losses are integrated to define the overall optimization goal of the discriminator and generator as,
where \(\gamma _{hadv}\) , \(\gamma _{fm}\) , and \(\gamma _{vgg}\) denote the weights corresponding to the losses, and set \(\mathscr {L}_{hadv}=1\) , \(\mathscr {L}_{fm}=10\) and \(\mathscr {L}_{vgg}=10\) in our experiments.
Figure 4 shows the variation trend of the discriminator and generator loss values with number of iterations during training on the Cityscapes dataset. Where the black and blue curves indicate the results of total loss and its correlated loss in training, respectively. We can observe that the correlated losses from the discriminator and generator are smoothly converging as the number of iterations increases. Moreover, the total losses display a positive correlation with its correlated losses. This indicates that our model mitigates the possibility of over-fitting over the training process owing to the reasonable design of the loss function.
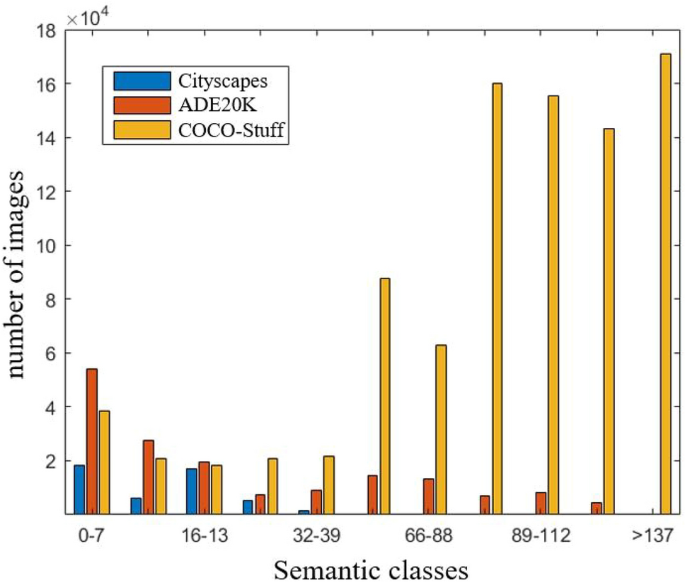
Distribution in the number of images corresponding to each semantic class on the public datasets of Cityscapes, ADE20K and COCO-Stuff.
Experiments
Experimental settings.
In order to validate the superiority of the proposed SC-UNet approach, we have carried out extensive experiments on three public datasets: Cityscapes 14 , ADE20K 15 , and COCO-Stuff 16 . The Cityscape dataset includes 35 semantic classes, while training and validation images are 2975 and 500, respectively. The ADE20K dataset has 150 semantic classes, while 20,210 training and 2000 validation images. The COCO-Stuff dataset comprises 182 semantic classes in addition to 118,287 training and 5000 validation images. The distribution in the number of images for each semantic class on the three datasets is displayed in Fig. 5 . As can be seen, there is an imbalance in the distribution of semantic categories. In addition, we adjusted the resolutions of the images in the cityscape, ADE20K and COCO-Stuf datasets to \(512\times 256\) , \(256\times 256\) and \(256\times 256\) , respectively, so as to verify the robustness of the proposed SC-UNet under different image resolutions.
The baseline models used to implement semantic image synthesis can be broadly classified into unsupervised and supervised. Unsupervised baselines aims to implement a translation of semantic maps to realistic images using unpaired training data. Unsupervised baseline models include CycleGAN 46 , DistanceGAN 47 , MUNIT 48 , DRIT 49 , GCGAN 50 , CUT 51 , USIS 52 and so on. In contrast, supervised baselines can produce higher quality images by utilising input data with labels. In supervised baseline models, the earlier CRN 29 and SIMS 35 are trained without using adversarial training. However, the GAN-based supervised baselines can be further subdivided into other 53 , 54 , 55 , 56 , normalization 22 , 56 , 57 , 58 , 59 , 60 , 61 , attention 7 , 8 , 23 , 31 , 62 , 63 , and discriminator 30 , 32 , 34 , 64 according to the improvement direction.
Evaluation metric
Referring to previous work, we adopts both the Fréchet Inception Distance (FID) 65 as image generation score to assess the perceptual quality and diversity of the synthesized images. Moreover, we also utilize the mean Intersection over Union (mIoU) 29 and the pixel Accuracy (Acc) 22 as semantic segmentation scores to measure the segmentation accuracy. We use the state-of-the-art segmentation networks for each dataset: DRN-D-105 66 for Cityscapes, UperNet101 67 for ADE20K, and DeepLabV2 68 for COCO-Stuff.
Implementation details
We utilize the ADAM optimizer 69 with \(\beta _1=0\) and \(\beta _2=0.999\) to train our models on a single RTX 3090Ti GPU. The learning rates of the generator and the discriminator are defined as lr /2 and \(lr * 2\) , where the initial value of the learning rate lr is set to 0.0002. To more accurately find the global optimal solution, the learning rate is dynamically changed during the training process. Formally, the dynamic learning rate is represented as follows:
where n is the total number of training epochs and \(m=n /2\) . According to the above formula, the learning rate will linearly decay to zero after m epochs. Furthermore,we train 200 epochs on the cityscape and ADE20K datasets to find the optimal solution, and 100 epochs on the COCO-Stuff dataset due to the large number of training images.

Qualitative comparison of our SC-UNet mothod with the competing methods on Cityscapes dataset. Our method generates images with better visual quality and higher-fidelity details.
Quantitative results
Table 2 gives the quantitative comparison results of our method with the supervised baselines in image generation score (FID) and semantic segmentation scores (mIoU and Acc) on the Cityscapes, ADE20K and COCO-Stuff datasets. The results in the table show that our method obtains a lower generation score (FID) than the previous supervised baselines on the validation set for each dataset. The lower the generation score, the higher the fidelity and diversity of the synthesized images produced by the deep learning network. In addition, our proposed method acquires a higher semantic segmentation scores (mIoU and Acc) than previous state-of-the-art models on the Cityscapes dataset, which has a small data amount and a relatively homogeneous distribution of semantic classes. In order to improve the semantic alignment with the input layout map, the latest OASIS 32 and SAFM 64 utilize the idea of semantic segmentation to improve the discriminator network. Although OASIS and SAFM obtain higher Acc and mIoU scores than our approach, this slight improvement only appears in the ADE20K and COCO-Stuff datasets with large data amounts and unbalanced semantic class distributions. Therefore, the quantitative comparison with the baselines confirms the superiority of our proposed network model in semantic image synthesis.
Furthermore, the quantitative comparison of our method with the unsupervised baselines is reported in Table 3 . Compared to the unsupervised baselines, we achieve better image generation score (FID) and semantic segmentation score (mIoU) on three public datasets by constructing a supervised model. Our improvement in the semantic segmentation score is particularly significant, mainly due to the supervised learning under the input semantic layouts. Moreover, the large amount of improvement indicates that the supervised strategy is more beneficial for the semantic image synthesis task.
Human perceptual evaluation
To further validate that our method performs better in the semantic image synthesis, we perform a human perception evaluation 22 , 23 , 56 to compare our approach with the several baseline methods of GauGAN 22 , DAGAN 63 , OASIS 32 , and SAFM 64 on the Cityscapes, ADE20K and COCO-Stuff datasets. Specifically, we first randomly select 200 semantic layout mappings from the validation set of each dataset to synthesis images for our method and the competing method. Then, we also randomly select 100 AMT workers to conduct the evaluation. Where AMT (known as Amazon Mechanical Turk 72 ) is a crowdsourcing marketplace that allows researchers to outsource their tasks to a distributed worker who can volunteer to perform the task for pay. Therefore, this experiment was carried out in accordance with relevant guidelines and regulations, and was obtained the approval of the AMT institutions, and the informed consent from all AMT workers. In each experiment, workers are required to select the perceptually more photo-realistic image from the shown two groups of synthesized images. The two groups of images are synthesized by our method and a competing method, respectively. Finally, we utilize the conventional statistical operations to obtain the average probability that the images synthesized by our method are selected by the workers on each dataset, and the results are shown in Table 5 . The comparison results of the human perception evaluation reaffirm our method, and the images synthesized by it are more acceptable in terms of quality.

Qualitative comparison results on the ADE20K dataset. Despite diverse semantic classes and small textures, our approach still ensures high fidelity.
Traditional statistical evaluation
To further emphasize the efficacy of our method in semantic image synthesis tasks, we employed conventional statistical assessment techniques, including F-statistic 73 , p-value 74 , and Analysis of Variance (ANOVA) 75 . As depicted in Table 6 , our approach yielded a lower F-statistic of 82.629 and a higher p-value of 5.2108. This observation suggests that, compared to existing unsupervised methods such as GauGAN 22 , OASIS 32 , and SAFM 64 , our method ensures minimal disparities among synthesized image samples. Additionally, ANOVA results indicate no discernible differentiation between the synthesized image dataset and the authentic image dataset, further substantiating the robustness of our approach.
Qualitative results
In Figs. 6 , 7 and 8 give the qualitative comparison of our model with the competing methods 22 , 64 on Cityscapes, ADE20K and COCO-Stuff datasets. We found that the images synthesized by our model not only have better perceptual quality, but also are closer to the ground truth images in the overall color and texture distribution. Note that the complex real-world scenes synthesized by our method show significant improvement on Cityscapes datasets. However, SAFM 64 is the current state-of-the-art method, but the images synthesized by it are too bright and even show color distortion. Compared with them, our proposed approach produces photo-realistic images while respecting the input semantic layout map, and can generate challenging scenes with high image fidelity.
Mean power spectrogram
We also calculated the mean power spectrograms of images synthesized by our method with competing methods 22 , 64 on the Cityscapes dataset to compare the qualitative from a signal perspective. The similarity matching result of the average power spectrum is shown in Fig. 9 . It is intuitively obvious that the two power spectrograms drawn separately from the ground-truth images and the synthesized images produced by our method are the most similar from the perspective of color, texture, and shape. Comparatively, the mean power spectrogram drawn from synthesized images produced by the competing methods showed distinct spikes. Some even present pseudo-local maxima, which are not observed in the average power spectrogram of the ground-truth images. Regarding the differences mentioned above, they can be clearly observed in the comparison of the zoomed-in areas. This enhancement allows for a more detailed examination of the discrepancies. Moreover, we utilize the ORB 70 and Histogram 71 algorithm to calculate the similarity between the ground-truth images and images synthesized by our method, and the results are shown in Table 4 . Where the higher the value, the more similarity. The similarity matching results calculated by the mean power spectrograms also can validate that the images synthesized by our method are more photo-realistic in details.
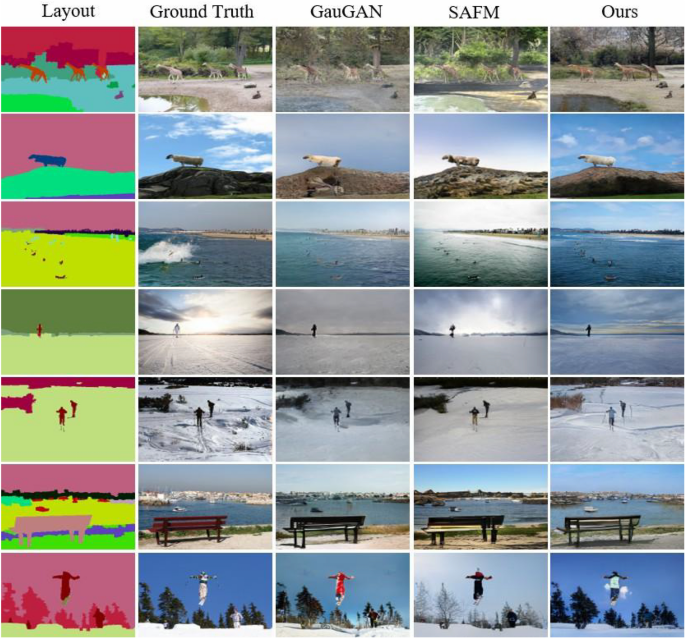
Qualitative comparison results on the COCO-Stuff dataset. The comparison results show that the images synthesized by our model have a higher quality than GauGAN and SAFM.
Ablation studies
Ablation on important components in sc-unet.
To verify the effectiveness of of each component in our SC-UNet method, we compare our SC-UNet method with three variants on the Citysacpes dataset. These three variants are obtained by gradually replacing or eliminating each component in the framework with our method as a benchmark. Specifically include: (i) âOursâ denotes our proposed SC-UNet model , which is used as a benchmark for the ablation experiments. (ii) âw/o SwinTâ denotes that the Swin Transformer (SwinT) module is replaced by the traditional convolutional block to construct a pure CNN-based UNet-like network. (iii) âw/o CRFâ represents that the designed Conditional Residual Fusion (CRF) block is replaced by the conventional residual block to fuse the high- and low-level feature information.(iv) âw/o OLMâ does not use the designed Opposition-based Learning Mechanism (OLM) to enhance the semantic feature information. (v) âw/o WAMâ does not use the Weight Assignment Mechanism (WAM) to allocate attention weights in channel and spatial dimensions. The results of the ablation study are shown in Table 7 . By the pair-wise comparison between our SC-UNet method and other variants, we can observe that the SwinT is used as the backbone network to achieve better synthetic performance than pure CNN. Furthermore, it also validates the effectiveness of the CRF block, OLM and WAM components in SC-UNet for high-squality image synthesis based on semantic layout maps. Although the âw/o OLMâ method is slightly lower than ours in terms of image synthesis score (FID) , the synthesized images from our SC-UNet approach have better performance in terms of two semantic segmentation scores, mIoU and Acc.
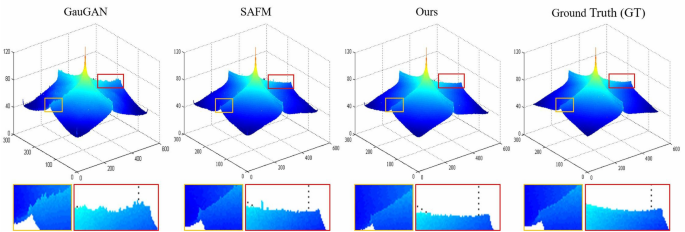
Mean power spectra over the Cityscapes dataset. Key differences are positioned with boxes on the mean power spectra, and shown magnified below image. Magnitude is on a linear scale.
Ablation on discriminator and loss function
Our SC-UNet approach employs adversarial training based on the multi-scale discriminator, which improves the synthesis performance. To highlight the superiority of multi-scale discriminator, we utilize two discriminators available for replacement: a single-scale Markov discriminator 23 (denoted as âPatchGANâ) and a feature pyramid semantic embedding discriminator 16 (denoted as âFPSE-Dâ). As shown in Table 8 , our SC-UNet method with aid of multi-scale discriminator not only performs well in terms of semantic segmentation scores (mIoU and Acc), but also excels in terms of image generation scores (FID).
To explore the effect of each loss function on semantic image synthesis, we use the combination of three loss functions as a baseline, and randomly replace or eliminate one of them for each comparison. Specifically, âw/o \(\mathscr {L}_{hadv}\) â denotes that the hinge-based adversarial loss is replaced by the conditional adversarial loss. âw/o \(\mathscr {L}_{fm}\) â and âw/o \(\mathscr {L}_{vgg}\) â represent constraints without the feature matching loss and the perceptual loss, respectively. As shown in Table 8 , hinge-based adversarial loss have more obvious advantages than conditional adversarial loss in semantic image synthesis. However, the fundamental difference between feature matching loss and perceptual loss is the image feature extraction network, and the two belong to a dynamic and a static relationship. Compared to the constraints of one loss, the combined effect of two losses can improve the quantitative quality of image synthesis.
Ablation on various image sizes
To explore the impact of image size on synthesis performance, we conducted an ablation study on different image sizes in Table 9 . First, images from the Cityscapes dataset were resized to \(1024\times 2048\) , \(512\times 1024\) , and \(256\times 512\) , respectively. Subsequently, we conducted model training by solely controlling the image size as the variable. The results in the table demonstrate that lower resolutions correspond to better synthesis performance. As the image resolution increases, so does the amount of detailed information contained within, necessitating the model to possess a stronger learning capacity for effective processing.

An example application of semantic control synthesis based on our SC-UNet method.
In this paper, we propose a new semantic image synthesis method (SC-UNet) , which can transform a given semantic layout map into the synthesized images with visual fidelity and semantic alignment. Our SC-UNet model is able to decode more photo-realistic images from the hierarchical feature representations encoded from the input semantic layout maps, by building a U-shaped network using the Swin Transformer module as the basic unit. Furthermore, the skip connection is added to a U-shaped network to combine the high- and low-level features of both sides. To compensate for the loss of semantic information resulted from down-sampling, the low-level features are copied to the high-level features by skip connections. An effective Conditional Residual Fusion (CRF) block is designed to obtain the important semantic and location information from the concatenation of high- and low-level features for higher-quality image synthesis and lower memory usage. The performance improvement of CRF blocks is mainly attributed to the embedding of a opposition-based learning mechanism and a weight assignment mechanism. The opposition-based learning mechanism can effectively enhance the semantic feature information, while the weight assignment mechanism can dynamically assign attentional weights in channel and spatial dimensions. Experimental results show that our proposed method outperforms state-of-the-art methods on three baseline datasets, both qualitatively and quantitatively. Moreover, our SC-UNet method can offer widespread applications, such as content generation and image editing, by adding, deleting, or editing objects. Two examples of applications based on the SC-UNet method are as follows.
Semantic control synthesis
Figure 10 displays an example application of semantic control synthesis based on our method. In figure, two semantic layout maps for model testing are selected from âADE_val_00000677.pngâ and âADE_val_00000851.pngâ in ADE20K dataset, respectively. Considering the complexity of manipulating the real scene image, we can change the input semantic layout map from its segmentation to remove or add the objects. Subsequently, the semantic class of a target object is changed, while our model manipulates the real image with the changed semantic layout map. Thus, an ordinary user is also able to interactively manipulate the real image. As can be seen from the results of the semantic control synthesis, our approach can generate realistic and semantically aligned images.
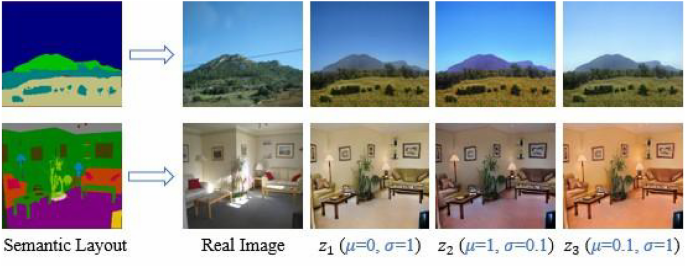
An example application of multi-style image synthesis based on our proposed SC-UNet method. \(z_1\) , \(z_2\) and \(z_3\) denote three different random noise tensors, respectively. The symbols \(\mu\) and \(\delta\) represent the mean and variance of the noise sampling, respectively.
Multi-style image synthesis
Figure 11 displays an example application of multi-style image synthesis based on our method. In figure, two semantic layout maps for model testing are selected from âADE_val_00000574.pngâ and âADE_val_ 00001512.pngâ in ADE20K dataset, respectively. We achieve three different styles of image synthesis from the same semantic layout map by randomly sampling of different 3D noise tensor z . Our method enables to synthesize different styles of high-fidelity images in indoor and outdoor scenes by noise sampling. The colour, luminance and illumination of the synthesised images can be adjusted, but the semantic structure is basically unchanged.
Data availability
Correspondence and requests for data and materials should be addressed to B.C.
Xu, H., Huang, C. & Wang, D. Enhancing semantic image retrieval with limited labeled examples via deep learning. Knowl.-Based Syst. 163 , 252â266 (2019).
Article  Google Scholar Â
Kumar, S., Singh, M. K. & Mishra, M. Efficient deep feature based semantic image retrieval. Neural Process. Lett. 1â24 (2023).
Hua, C.-H., Huynh-The, T., Bae, S.-H. & Lee, S. Cross-attentional bracket-shaped convolutional network for semantic image segmentation. Inf. Sci. 539 , 277â294 (2020).
Article  MathSciNet  Google Scholar Â
Fan, Z. et al. Self-attention neural architecture search for semantic image segmentation. Knowl.-Based Syst. 239 , 107968 (2022).
Ma, Y., Yu, L., Lin, F. & Tian, S. Cross-scale sampling transformer for semantic image segmentation. J. Intell. Fuzzy Syst. 1â13 (2023).
Ke, A., Liu, G., Chen, J. & Wu, X. Trilateral GAN with channel attention residual for semantic image synthesis. In 2021 International Conference on Computer Information Science and Artificial Intelligence (CISAI) . 1123â1129 (IEEE, 2021).
Tang, H., Torr, P. H. & Sebe, N. Multi-channel attention selection GANs for guided image-to-image translation. IEEE Trans. Pattern Anal. Mach. Intell. 45 , 6055â6071 (2022).
Google Scholar Â
Tang, H., Shao, L., Torr, P. H. & Sebe, N. Local and global GANs with semantic-aware upsampling for image generation. In IEEE Transactions on Pattern Analysis and Machine Intelligence (TPAMI) (2022).
Xu, H., He, W., Zhang, L. & Zhang, H. Unsupervised spectral-spatial semantic feature learning for hyperspectral image classification. IEEE Trans. Geosci. Remote Sens. 60 , 1â14 (2022).
Sezen, A., Turhan, C. & Sengul, G. A hybrid approach for semantic image annotation. IEEE Access 9 , 131977â131994 (2021).
Tian, D. & Zhang, Y. Multi-instance learning for semantic image analysis. In International Conference on Intelligent Information Processing . 473â484 (Springer, 2022).
Liu, Z. et al. Swin transformer: Hierarchical vision transformer using shifted windows. In Proceedings of the IEEE/CVF International Conference on Computer Vision . 9992â10002 (2021).
Zhang, B. et al. Styleswin: Transformer-based gan for high-resolution image generation. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 11304â11314 (2022).
Cordts, M. et al. The cityscapes dataset for semantic urban scene understanding. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 3213â3223 (2016).
Zhou, B. et al. Scene parsing through ade20k dataset. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 633â641 (2017).
Lee, C.-H., Liu, Z., Wu, L. & Luo, P. Maskgan: Towards diverse and interactive facial image manipulation. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 5549â5558 (2020).
Goodfellow, I. et al. Generative adversarial networks. Commun. ACM 63 , 139â144 (2020).
Liu, D. et al. View synthesis-based light field image compression using a generative adversarial network. Inf. Sci. 545 , 118â131 (2021).
Liu, R., Yu, Q. & Yu, S. X. Unsupervised sketch to photo synthesis. In Computer VisionâECCV 2020: 16th European Conference, Glasgow, UK, August 23â28, 2020, Proceedings, Part III 16 . 36â52 (Springer, 2020).
Chen, S.-Y., Su, W., Gao, L., Xia, S. & Fu, H. Deepfacedrawing: Deep generation of face images from sketches. ACM Trans. Graph. (TOG) 39 , 72â81 (2020).
Liu, B., Zhu, Y., Song, K. & Elgammal, A. Self-supervised sketch-to-image synthesis. Proc. AAAI Conf. Artif. Intell. 35 , 2073â2081 (2021).
Park, T., Liu, M.-Y., Wang, T.-C. & Zhu, J.-Y. Semantic image synthesis with spatially-adaptive normalization. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 2337â2346 (2019).
Wang, Y., Qi, L., Chen, Y.-C., Zhang, X. & Jia, J. Image synthesis via semantic composition. In Proceedings of the IEEE/CVF International Conference on Computer Vision . 13749â13758 (2021).
Luo, X., Chen, X., He, X., Qing, L. & Tan, X. Cmafgan: A cross-modal attention fusion based generative adversarial network for attribute word-to-face synthesis. Knowl.-Based Syst. 255 , 109750 (2022).
Zhang, Z., Zhou, J., Yu, W. & Jiang, N. Text-to-image synthesis: Starting composite from the foreground content. Inf. Sci. 607 , 1265â1285 (2022).
Zhang, H., Yang, S. & Zhu, H. CJE-TIG: Zero-shot cross-lingual text-to-image generation by corpora-based joint encoding. Knowl.-Based Syst. 239 , 108006 (2022).
Zhan, B. et al. D2fe-GAN: Decoupled dual feature extraction based GAN for MRI image synthesis. Knowl. -Based Syst. 252 , 109362 (2022).
Yang, M., Wang, Z., Chi, Z. & Du, W. Protogan: Towards high diversity and fidelity image synthesis under limited data. Inf. Sci. 632 , 698â714 (2023).
Chen, Q. & Koltun, V. Photographic image synthesis with cascaded refinement networks. In Proceedings of the IEEE International Conference on Computer Vision . 1511â1520 (2017).
Wang, T.-C. et al. High-resolution image synthesis and semantic manipulation with conditional GANs. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 8798â8807 (2018).
Tang, H., Xu, D., Yan, Y., Torr, P. H. & Sebe, N. Local class-specific and global image-level generative adversarial networks for semantic-guided scene generation. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 7870â7879 (2020).
Sushko, V. et al. Oasis: Only adversarial supervision for semantic image synthesis. Int. J. Comput. Vis. 130 , 2903â2923 (2022).
Isola, P., Zhu, J.-Y., Zhou, T. & Efros, A. A. Image-to-image translation with conditional adversarial networks. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 1125â1134 (2017).
Liu, X., Yin, G., Shao, J., Wang, X. et al. Learning to predict layout-to-image conditional convolutions for semantic image synthesis. Adv. Neural Inf. Process. Syst. 32 (2019).
Qi, X., Chen, Q., Jia, J. & Koltun, V. Semi-parametric image synthesis. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 8808â8816 (2018).
He, K., Zhang, X., Ren, S. & Sun, J. Deep residual learning for image recognition. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 770â778 (2016).
Zhou, R., Achanta, R. & SĂŒsstrunk, S. Deep residual network for joint demosaicing and super-resolution. arXiv preprint arXiv:1802.06573 (2018).
Wang, H., Wu, Z. & Rahnamayan, S. Enhanced opposition-based differential evolution for solving high-dimensional continuous optimization problems. Soft Comput. 15 , 2127â2140 (2011).
Gao, C., Cai, Q. & Ming, S. Yolov4 object detection algorithm with efficient channel attention mechanism. In 2020 5th International Conference on Mechanical, Control and Computer Engineering (ICMCCE) . 1764â1770 (IEEE, 2020).
Zhu, X., Cheng, D., Zhang, Z., Lin, S. & Dai, J. An empirical study of spatial attention mechanisms in deep networks. In Proceedings of the IEEE/CVF International Conference on Computer Vision . 6688â6697 (2019).
Li, H. et al. Scattnet: Semantic segmentation network with spatial and channel attention mechanism for high-resolution remote sensing images. IEEE Geosci. Remote Sens. Lett. 18 , 905â909 (2020).
Article  ADS  Google Scholar Â
Ba, J. L., Kiros, J. R. & Hinton, G. E. Layer normalization. arXiv preprint arXiv:1607.06450 (2016).
Ioffe, S. & Szegedy, C. Batch normalization: Accelerating deep network training by reducing internal covariate shift. In International Conference on Machine Learning . 448â456 (PMLR, 2015).
Kim, J., Kim, M., Kang, H. & Lee, K. U-gat-it: Unsupervised generative attentional networks with adaptive layer-instance normalization for image-to-image translation. arXiv preprint arXiv:1907.10830 (2019).
Zhao, J., Mathieu, M. & LeCun, Y. Energy-based generative adversarial network. arXiv preprint arXiv:1609.03126 (2016).
Zhu, J.-Y., Park, T., Isola, P. & Efros, A. A. Unpaired image-to-image translation using cycle-consistent adversarial networks. In Proceedings of the IEEE International Conference on Computer Vision . 2223â2232 (2017).
Benaim, S. & Wolf, L. One-sided unsupervised domain mapping. Adv. Neural Inf. Process. Syst. 30 (2017).
Huang, X., Liu, M.-Y., Belongie, S. & Kautz, J. Multimodal unsupervised image-to-image translation. In Proceedings of the European Conference on Computer Vision (ECCV) . 172â189 (2018).
Lee, H.-Y., Tseng, H.-Y., Huang, J.-B., Singh, M. & Yang, M.-H. Diverse image-to-image translation via disentangled representations. In Proceedings of the European Conference on Computer Vision (ECCV) . 35â51 (2018).
Fu, H. et al. Geometry-consistent generative adversarial networks for one-sided unsupervised domain mapping. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 2427â2436 (2019).
Park, T., Efros, A. A., Zhang, R. & Zhu, J.-Y. Contrastive learning for unpaired image-to-image translation. In Computer VisionâECCV 2020: 16th European Conference, Glasgow, UK, August 23â28, 2020, Proceedings, Part IX 16 . 319â345 (Springer, 2020).
Eskandar, G., Abdelsamad, M. & Armanious, K. & Yang, B. Unsupervised Semantic Image Synthesis. Computers & Graphics (Usis, 2023).
Zhu, J.-Y. et al. Toward multimodal image-to-image translation. Adv. Neural Inf. Process. Syst. 30 (2017).
Dundar, A., Sapra, K., Liu, G., Tao, A. & Catanzaro, B. Panoptic-based image synthesis. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 8070â8079 (2020).
Li, Y. et al. Bachgan: High-resolution image synthesis from salient object layout. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 8365â8374 (2020).
Ntavelis, E., Romero, A., Kastanis, I., Van Gool, L. & Timofte, R. Sesame: Semantic editing of scenes by adding, manipulating or erasing objects. In Computer VisionâECCV 2020: 16th European Conference, Glasgow, UK, August 23â28, 2020, Proceedings, Part XXII 16 . 394â411 (Springer, 2020).
Jiang, L. et al. Tsit: A simple and versatile framework for image-to-image translation. In Computer VisionâECCV 2020: 16th European Conference, Glasgow, UK, August 23â28, 2020, Proceedings, Part III 16 . 206â222 (Springer, 2020).
Yang, D., Hong, S., Jang, Y., Zhao, T. & Lee, H. Diversity-sensitive conditional generative adversarial networks. arXiv preprint arXiv:1901.09024 (2019).
Zhu, Z., Xu, Z., You, A. & Bai, X. Semantically multi-modal image synthesis. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 5467â5476 (2020).
Shi, Y., Liu, X., Wei, Y., Wu, Z. & Zuo, W. Retrieval-based spatially adaptive normalization for semantic image synthesis. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 11224â11233 (2022).
Tan, Z. et al. Efficient semantic image synthesis via class-adaptive normalization. IEEE Trans. Pattern Anal. Mach. Intell. 44 , 4852â4866. https://doi.org/10.1109/TPAMI.2021.3076487 (2022).
Article  PubMed  Google Scholar Â
Tang, H. et al. Multi-channel attention selection GAN with cascaded semantic guidance for cross-view image translation. In Proceedings of the IEEE/CVF conference on computer vision and pattern recognition . 2417â2426 (2019).
Tang, H., Bai, S. & Sebe, N. Dual attention GANs for semantic image synthesis. In Proceedings of the 28th ACM International Conference on Multimedia . 1994â2002 (2020).
Lv, Z., Li, X., Niu, Z., Cao, B. & Zuo, W. Semantic-shape adaptive feature modulation for semantic image synthesis. In Proceedings of the IEEE/CVF Conference on Computer Vision and Pattern Recognition . 11214â11223 (2022).
Seitzer, M. Pytorch-fid: FID Score for PyTorch. Version 0.3.0. . https://github.com/mseitzer/pytorch-fid (2020).
Yu, F., Koltun, V. & Funkhouser, T. Dilated residual networks. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition . 472â480 (2017).
Xiao, T., Liu, Y., Zhou, B., Jiang, Y. & Sun, J. Unified perceptual parsing for scene understanding. In Proceedings of the European Conference on Computer Vision (ECCV) . 418â434 (2018).
Chen, L.-C., Papandreou, G., Kokkinos, I., Murphy, K. & Yuille, A. L. Deeplab: Semantic image segmentation with deep convolutional nets, atrous convolution, and fully connected CRFS. IEEE Trans. Pattern Anal. Mach. Intell. 40 , 834â848 (2017).
Kingma, D. P. & Ba, J. Adam: A method for stochastic optimization. arXiv preprint arXiv:1412.6980 (2014).
Rublee, E., Rabaud, V., Konolige, K. & Bradski, G. Orb: An efficient alternative to sift or surf. In 2011 International Conference on Computer Vision . 2564â2571 (IEEE, 2011).
Wang, Z., Bovik, A. C., Sheikh, H. R. & Simoncelli, E. P. Image quality assessment: From error visibility to structural similarity. IEEE Trans. Image Process. 13 , 600â612 (2004).
Article  ADS  PubMed  Google Scholar Â
Buhrmester, M., Kwang, T. & Gosling, S. D. Amazonâs mechanical turk: A new source of inexpensive, yet high-quality, data?. Perspect. Psychol. 6 , 3â5 (2011).
Fisher, R. A. Statistical methods for research workers. In Breakthroughs in Statistics: Methodology and Distribution . 66â70 (Springer, 1970).
Pearson, K. On the Criterion that a Given System of Deviations from the Probable in the Case of a Correlated System of Variables is Such that it Can be Reasonbly Supposed to have Arisen from Random Sampling (1900).
KPFRS, L. On lines and planes of closest fit to systems of points in space. In Proceedings of the 17th ACM SIGACT-SIGMOD-SIGART Symposium on Principles of Database Systems (SIGMOD) . Vol. 19 (1901).
Download references
Acknowledgements
We gratefully acknowledge funding by National Natural Science Foundation of China grant number 61971316.
Author information
Authors and affiliations.
School of Cyber Science and Engineering, Wuhan University, Wuhan, 430072, China
Aihua Ke, Jian Luo & Bo Cai
Key Laboratory of Aerospace Information Security and Trusted Computing, Ministry of Education, Wuhan, 430072, China
You can also search for this author in PubMed  Google Scholar
Contributions
A.K. and B.C. conceived the experiment(s), A.K. and J.L. conducted the experiment(s), A.K. and J.L. analysed the results, J.L. and A.K. data curation, A.K. and J.L. writing-original draft preparation, J.L. and A.K. formal analysis, J.L. and B.C. validation, All authors reviewed the manuscript.
Corresponding author
Correspondence to Bo Cai .
Ethics declarations
Competing interests.
The authors declare no competing interests.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Rights and permissions
Open Access This article is licensed under a Creative Commons Attribution 4.0 International License, which permits use, sharing, adaptation, distribution and reproduction in any medium or format, as long as you give appropriate credit to the original author(s) and the source, provide a link to the Creative Commons licence, and indicate if changes were made. The images or other third party material in this article are included in the articleâs Creative Commons licence, unless indicated otherwise in a credit line to the material. If material is not included in the articleâs Creative Commons licence and your intended use is not permitted by statutory regulation or exceeds the permitted use, you will need to obtain permission directly from the copyright holder. To view a copy of this licence, visit http://creativecommons.org/licenses/by/4.0/ .
Reprints and permissions
About this article
Cite this article.
Ke, A., Luo, J. & Cai, B. UNet-like network fused swin transformer and CNN for semantic image synthesis. Sci Rep 14 , 16761 (2024). https://doi.org/10.1038/s41598-024-65585-1
Download citation
Received : 07 March 2024
Accepted : 21 June 2024
Published : 21 July 2024
DOI : https://doi.org/10.1038/s41598-024-65585-1
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
By submitting a comment you agree to abide by our Terms and Community Guidelines . If you find something abusive or that does not comply with our terms or guidelines please flag it as inappropriate.
Quick links
- Explore articles by subject
- Guide to authors
- Editorial policies
Sign up for the Nature Briefing: AI and Robotics newsletter â what matters in AI and robotics research, free to your inbox weekly.

- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectivesâą on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What is the purpose of assignment operator used in conditional statements in JavaScript? [closed]
In python, conditional statements(If-else) takes only True/False in its expression field. i.e,
But, in JavaScript, conditional statements can take Assignment operator too. i.e,
My question is:- In Js, while using Assignment operator in Conditional statements, definitely it will become true. So, in that case, there is no need to use Conditional statements right? Instead we can write,
Is there any beneficial reason to use Assignment Operator in Conditional Statements?
- conditional-statements
- assignment-operator
- 1 just because your example is trivial, it doesn't mean the feature is useless. in python you have now the "walrus operator" := which is indeed very useful – folen gateis Commented Jul 25 at 16:24
- 1 "definitely it will become true" - How did you get that idea? – no comment Commented Jul 25 at 16:33
- Conditional statements can take the results of any expression in Python, not just boolean True/False. – notovny Commented Jul 25 at 18:23
- in your second example, try let b = 0; ... the "assignment operator" as you call it is just shorthand for assigning a value AND checking it .. a less trivial (but still trivial) example could be if ((a = b) == c) { ... } - personally, I never use such code, since the days of 33kilobaud internet connections are long gone, so no more need for less readable brevity in your code - besides, code minifiers exist to minify easily readable development code to space saving production code – Jaromanda X Commented Jul 25 at 23:58
Browse other questions tagged javascript python conditional-statements assignment-operator or ask your own question .
- Featured on Meta
- Announcing a change to the data-dump process
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- Looking for a book I read pre-1990. Possibly called "The Wells of Yutan". A group of people go on a journey up a river
- How can I break equation in forest?
- Estimate of a trigonometric product
- Have the results of any U.S. presidential election other than 2000, 2020 been widely disputed and litigated?
- 7x10 floor and a 8x8 and a 6x1 carpet, only one cut allowed
- I found these wild prickly plants on a trip in Cambridge, what are they?
- Identifying SMD Capacitors marked "33 10u" and "47 5v"
- Why is my internet speed slowing by 10x when using this coax cable and splitter setup?
- How to fix "Google Play is linked to another account" in Bomber Friends?
- How do RF broadcast towers switch so much power so quickly?
- Is it true that the letter I is pronounced as a consonant when it's the first letter of a syllable?
- How fast does the Parker solar probe move before/at aphelion?
- Why is much harder to encrypt emails, compared to web pages?
- Has Donald Trump or his campaign explained what his plan is so that "we’ll have it fixed so good you’re not gonna have to vote"?
- How many islands can papa Smurf visit at most?
- What did Rohan and Gondor think Sauron was?
- Does forgetful functor commute with limits?
- What happens after "3.9% APR Financing for 36 Months"?
- Terminology: A "corollary" to a proof?
- Do amateurs still search for supernovae?
- Is copy elision in the form of named return value optimization permitted in C?
- How do I prepare a longer campaign for mixed-experience players?
- How to make sure a payment is actually received and will not bounce, using Paypal, Stripe or online banking respectively?
- Can we simply remove the log term for loss in policy gradient methods?
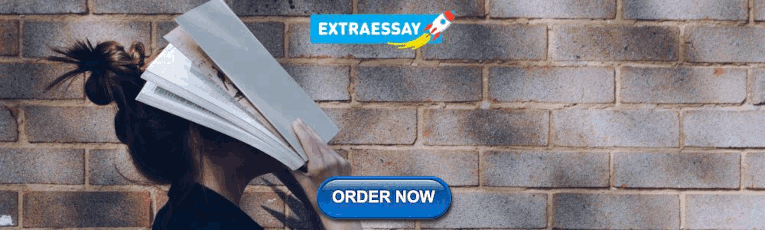
IMAGES
VIDEO
COMMENTS
Therefore, only conditional assignment to a reference through the ?: operator conveys the semantics of Initializing a variable from only one of two choices based on a predicate appropriately. Furthermore, the conditional operator can yield an lvalue, i.e. a value to which another value can be assigned. Consider the following example:
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
Let's see a code snippet to understand it better. a = 10. b = 20 # assigning value to variable c based on condition. c = a if a > b else b. print(c) # output: 20. You can see we have conditionally assigned a value to variable c based on the condition a > b. 2. Using if-else statement.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
Inline conditional assignment in single lines of code. Advantages of Ternary Expression: Concise syntax, reducing the need for multiple lines of code. Suitable for simple conditional assignments. Disadvantages of Ternary Expression: Can reduce code readability, especially for complex conditions or expressions. Limited to simple assignments; not ...
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed. Conditional operator and an if statement. Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following ...
6.2.4 Conditional Variable Assignment. There is another assignment operator for variables, ...
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.. Syntax of Conditional/Ternary Operator in C
Readability: The conditional operator can enhance code readability, especially for simple conditional assignments. It clearly expresses the intent of assigning different values based on a condition in a single line. And some disadvantages: Limited functionality: The conditional operator is primarily used for simple conditional assignments. It ...
It allows for conditional execution of a statement or group of statements based on the value of an expression. The outline of this tutorial is as follows: ... A common use of the conditional expression is to select variable assignment. For example, suppose you want to find the larger of two numbers. Of course, ...
Nested Conditional Operators. There are examples in which it might be useful to combine two or more conditional operators in a single assignment. Consider the truth table below. The truth table shows a 2-input truth table. You need to know the value of both r_Sel[1] and r_Sel[0] to determine the value of the output w_Out.
The concurrent conditional signal assignment statement is a shorthand for a collection of simple signal assignments contained in an if statement, which is in turn contained in a process statement. Let us look at some examples and show how each conditional signal assignment can be transformed into an equivalent process statement.
C and many other languages have a conditional (AKA ternary) operator. This allows you to make very terse choices between two values based on the truth of a condition, which makes expressions, including assignments, very concise. I miss this because I find that my code has lots of conditional assignments that take four lines in Python: var ...
Method 1: Ternary Operator. The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True. Otherwise, if the expression c evaluates to False, the ternary operator returns the alternative expression y. <OnTrue> if <Condition> else <OnFalse>. Operand.
Conditional Assignment means that the Transfer of Rights will happen from the Assignor to the Assignee subject to certain terms and conditions. If the conditions are fulfilled then only the Policy will get transferred from the Assignor to the Assignee. The process of transferring rights of a Life Insurance Policy is called Assignment.
Conditional Assignment. The Design-Builder hereby conditionally assigns to the Owner all of its interest in any subcontracts (including, without limitation, purchase orders) entered into by the Design-Builder for performance of any part of the Work.Such conditional assignment shall become effective only upon the termination of this Contract, whereupon the Owner shall succeed to the rights and ...
Basics of the conditional expression (ternary operator) In Python, the conditional expression is written as follows. The condition is evaluated first. If condition is True, X is evaluated and its value is returned, and if condition is False, Y is evaluated and its value is returned. If you want to switch the value based on a condition, simply ...
Programmers like to use the concise ternary operator for conditional assignments instead of lengthy if-else statements. The ternary operator takes three arguments: Firstly, the comparison argument. Secondly, the value (or result of an expression) to assign if the comparison is true.
Is there a ternary conditional operator in Python? Yes, it was added in version 2.5. The expression syntax is: a if condition else b First condition is evaluated, then exactly one of either a or b is evaluated and returned based on the Boolean value of condition.If condition evaluates to True, then a is evaluated and returned but b is ignored, or else when b is evaluated and returned but a is ...
Create an Assignment; Set a due date; Set Conditional Availability with a Show Date and a Hide Date that surrounds the due date; After the Due Date passes, navigate to the Grade Center > Full Grade Center > View Assignment created in Step 3. Note: Assignment Icon displays as Red (as no submissions are present)
There is conditional assignment in Python 2.5 and later - the syntax is not very obvious hence it's easy to miss. Here's how you do it: x = true_value if condition else false_value For further reference, check out the Python 2.5 docs.
An effective Conditional Residual Fusion (CRF) block is designed to obtain the important semantic and location information from the concatenation of high- and low-level features for higher-quality ...
to continue to Microsoft Entra. No account? Create one! Can't access your account?
What is the need for the conditional operator? Functionally it is redundant, since it implements an if-else construct. If the conditional operator is more efficient than the equivalent if-else assignment, why can't if-else be interpreted more efficiently by the compiler?
My question is:- In Js, while using Assignment operator in Conditional statements, definitely it will become true. So, in that case, there is no need to use Conditional statements right? Instead we can write, let a = 3; let b = 5; a = b; console.log(a, b); Is there any beneficial reason to use Assignment Operator in Conditional Statements?