Null-Coalescing Assignment Operator in C#
Back to: C#.NET Tutorials For Beginners and Professionals
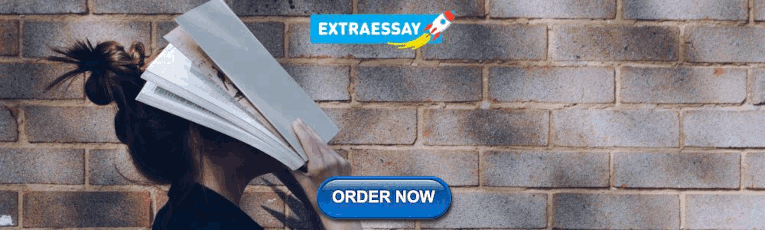
Null-Coalescing Assignment Operator in C# 8
Null-coalescing assignment operator (=) in c# 8.
C# 8.0 introduces the null-coalescing assignment operator ??= . We can use this ??= operator to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null. That means the null-coalescing assignment operator ??= assigns a variable only if it is null. The syntax is given below.
It simplifies a common coding pattern where a variable is assigned with a value if it is null. For a better understanding, please have a look at the below diagram. Here, you can observe before C# 8, how we are checking null and assigning a value if it is null and how we can achieve the same in C# 8 using the null-coalescing assignment (??=) operator.
Points to Remember While working with ??= in C#:
Null-coalescing assignment operator example in c#:, real-time use cases of null-coalescing assignment operator, example using if statements:, same example using null-coalescing assignment = operator:.
In the next article, I am going to discuss Unmanaged Constructed Types in C# 8 with Examples. Here, in this article, I try to explain Null-Coalescing Assignment in C# 8 with Examples. I hope you enjoy this Null-Coalescing Assignment in C# with Examples article.
About the Author: Pranaya Rout
Leave a Reply Cancel reply
- Indices and Ranges
- Interface Default Method Implementation
- Readonly Members
- Switch Expressions
- Using Declaration
- Static Local Functions
- Nullable Reference Types
- Asynchronous Streams
- Null-coalescing Assignment
- Unmanaged Constructed Types
- Stackalloc in Nested Expressions
C# 8 Null-coalescing Assignment
Fastest entity framework extensions.
The null-coalescing operator ?? returns the value of its left-hand operand if it is not a null value. If it is null, then it evaluates the right-hand operand and returns its result.
- In C# 8.0, a new null-coalescing assignment operator ??= assigns the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null.
- The ??= operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null.
- The left-hand operand of the ??= operator must be a variable, a property, or an indexer element.
Before C# 8, the type of the left-hand operand of the ?? operator must be either a reference type or a nullable value type. In C# 8.0, the requirement is changed, and now the type of the left-hand operand of the ?? and ??= operators cannot be a non-nullable value type.
You can use the null-coalescing operators with unconstrained type parameters.
In expressions with the null-conditional operators ? and ?[] , you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null`.
The operators ?? and ??= cannot be overloaded.
Got any C# 8 Question?

- Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks.
- Essential C#
- Announcements
As described in Chapter 3, while null can be a useful value, it also comes with a few challenges—namely, the need to check a value isn’t null before invoking the object’s member or changing the value from null to something more appropriate to the circumstance.
Although you can check for null using the equality operators and even the relational equality operators, there are several other ways to do so, including C# 7.0’s support for is null and C# 10.0’s support for is not null . In addition, several operators are designed exclusively for working with the potential of a null value. These include the null-coalescing operator (and C# 8.0’s null-coalescing assignment) and the null-conditional operator. There is even an operator to tell the compiler when you believe a value isn’t null even if it isn’t obvious to the compiler—the null-forgiving operator. Let’s start by simply checking whether a value is null or not.
It turns out there are multiple ways to check for null , as shown in Table 4.4 . For each listing, assume a declaration of string? uriString precedes it:
string ? uriString = null ;
Description | Example |
is null Operator Pattern Matching The is operator provides multiple approaches to check for null. Starting with C# 7.0, you can check whether a value is null using the is null expression. This is a succinct and clear approach to checking if a value is null. | // 1. if (uriString is null) { Console.WriteLine( "Uri is null"); } |
is not null Operator Pattern Matching Similarly, in C# 9.0, is not null support was added. And, when checking for not null, this is the preferred approach. | // 2. if (uriString is not null) { Console.WriteLine( "Uri is not null"); } |
Equality/Inequality Using the equality and inequality operators works with all versions of C#. In addition, checking for null this way is readable. The only disadvantage of this approach is that it is possible to override the equality/inequality operator, potentially introducing minor performance impacts. | // 3. if(uriString == null) { Console.WriteLine( "Uri is null"); }
if(uriString != null) { Console.WriteLine( $"Uri is: { uriString }"); } |
is object Since C# 1.0, is object checks whether the operand is null, although this syntax isn’t as clear as is not null. is object is preferable over the is {} expression described in the next row because it issues a warning when the operand is a non-nullable value type. What is the point in checking for null if the operand can’t be null? | // 4. int number = 0; if( (uriString is object ) // Warning CS0183: The given // expression is always not // null. && (number is object) ) { Console.WriteLine( $"Uri is: { uriString }"); } |
is { } Operator Pattern Matching The property pattern matching expression, <operand> is { }, (which was added in C# 8.0) provides virtually the same functionality as is object. Besides being less readable, the only noticeable disadvantage is that is {} with a value type expression doesn’t issue a warning, while is object does. And, since a value type cannot be null, the warning is preferable because there is no point in checking a non-nullable value for null. Therefore, favor using is object over is { }, | // 5. if (uriString is {}) { Console.WriteLine( $"Uri is: { uriString }"); } |
ReferenceEquals() While object.ReferenceEquals() is a somewhat long syntax for such a simple operation, like equality/inequality it works with all versions of C# and has the advantage of not allowing overriding; thus, it always executes what the name states. | // 6.
if(ReferenceEquals( uriString, null)) { Console.WriteLine( "Uri is null"); } |
Of course, having multiple ways to check whether a value is null raises the question as to which one to use. C# 7.0’s enhanced is null and C# 9.0’s is not null syntax are preferable if you are using a modern version of C#.
Obviously, if you are programming with C# 6.0 or earlier, the equality/inequality operators are the only option aside from using is object to check for not null . The latter has a slight advantage since it is not possible to change the definition of the is object expression and (albeit unlikely) introduce a minor performance hit. This renders is {} effectively obsolete. Using ReferenceEquals() is rare, but it does allow comparison of values that are of unknown data types, which is helpful when implementing a custom version of the equality operator (see “ Operator Overloading ” in Chapter 10).
Several of the rows in Table 4.4 leverage pattern matching, a concept covered in more detail in the “ Pattern Matching ” section of Chapter 7.
The null-coalescing operator is a concise way to express “If this value is null , then use this other value.” It has the following form:
expression1 ?? expression2
The null-coalescing operator also uses a form of short-circuiting. If expression1 is not null , its value is the result of the operation and the other expression is not evaluated. If expression1 does evaluate to null , the value of expression2 is the result of the operator. Unlike the conditional operator, the null-coalescing operator is a binary operator.
Listing 4.37 illustrates the use of the null-coalescing operator.
In this listing, we use the null-coalescing operator to set fileName to "config.json" if GetFileName() is null . If GetFileName() is not null , fileName is simply assigned the value of GetFileName() .
The null-coalescing operator “chains” nicely. For example, an expression of the form x ?? y ?? z results in x if x is not null ; otherwise, it results in y if y is not null ; otherwise, it results in z . That is, it goes from left to right and picks out the first non- null expression, or uses the last expression if all the previous expressions were null . The assignment of directory in Listing 4.37 provides an example.
C# 8.0 provides a combination of the null-coalescing operator and the assignment operator with the addition of the null-coalescing assignment operator . With this operator, you can evaluate if the left-hand side is null and assign the value on the righthand side if it is. Listing 4.37 uses this operator when assigning fullName .
In recognition of the frequency of the pattern of checking for null before invoking a member, you can use the ?. operator, known as the null-conditional operator , 4 as shown in Listing 4.38 . 5
The null-conditional operator example ( int? length = segments?.Length ) checks whether the operand (the segments in Listing 4.38 ) is null prior to invoking the method or property (in this case, Length ). The logically equivalent explicit code would be the following (although in the original syntax, the value of segments is evaluated only once):
int ? length =
(segments != null ) ? (int?)segments.Length : null
An important thing to note about the null-conditional operator is that it always produces a nullable value. In this example, even though the string.Length member produces a non-nullable int , invoking Length with the null-conditional operator produces a nullable int ( int? ).
You can also use the null-conditional operator with the array accessor. For example, uriString = segments?[0] produces the first element of the segments array if the segments array was not null . Using the array accessor version of the null-conditional operator is relatively rare, however, as it is only useful when you don’t know whether the operand is null , but you do know the number of elements, or at least whether a particular element exists.
What makes the null-conditional operator especially convenient is that it can be chained (with and without more null-coalescing operators). For example, in the following code, both ToLower() and StartWith() will be invoked only if both segments and segments[0] are not null :
segments?[0]?.ToLower().StartsWith( "file:" );
In this example, of course, we assume that the elements in segments could potentially be null , so the declaration (assuming C# 8.0) would more accurately have been
string ?[]? segments;
The segments array is nullable, in other words, and each of its elements is a nullable string.
When null-conditional expressions are chained, if the first operand is null , the expression evaluation is short-circuited, and no further invocation within the expression call chain will occur. You can also chain a null-coalescing operator at the end of the expression so that if the operand is null , you can specify which default value to use:
string uriString = segments?[0]?.ToLower().StartsWith(
"file:" ) ?? "intellitect.com" ;
Notice that the data type resulting from the null-coalescing operator is not nullable (assuming the right-hand side of the operator [ "intellitect.com" in this example] is not null —which would make little sense).
Be careful, however, that you don’t unintentionally neglect additional null values. Consider, for example, what would happen if ToLower() (hypothetically, in this case) returned null . In this scenario, a NullReferenceException would occur upon invocation of StartsWith() . This doesn’t mean you must use a chain of null-conditional operators, but rather that you should be intentional about the logic. In this example, because ToLower() can never be null , no additional null-conditional operator is necessary.
Although perhaps a little peculiar (in comparison to other operator behavior), the return of a nullable value type is produced only at the end of the call chain. Consequently, calling the dot ( . ) operator on Length allows invocation of only int (not int? ) members. However, encapsulating segments?.Length in parentheses—thereby forcing the int? result via parentheses operator precedence—will invoke the int? return and make the Nullable<T> specific members ( HasValue and Value ) available. In other words, segments?.Length.Value won’t compile because int (the data type returned by Length ) doesn’t have a member called Value . However, changing the order of precedence using (segments?.Length).Value will resolve the compiler error because the return of (segments?.Length) is int? , so the Value property is available.
Notice that the Join() invocation of Listing 4.38 includes an exclamation point after segments :
uriString = string .Join( '/' , segments ! );
At this point in the code, segments?.Length is assigned to the length variable, and since the if statement verifies that length is not null , we know that segments can’t be null either.
int ? length = segments?.Length;
if (length is not null && length != 0){ }
However, the compiler doesn’t have the capability to make the same determination. And, since Join() requires a non-nullable string array, it issues a warning when passing an unmodified segments variable whose declaration was nullable. To avoid the warning, we can add the null-forgiving operator ( ! ), starting in C# 8.0. It declares to the compiler that we, as the programmer, know better, and that the segments variable is not null . Then, at compile time, the compiler assumes we know better and dismisses the warning (although the runtime still checks that our assertion is not null at execution time).
Note that while the null-conditional operator checks that segments isn’t null, it doesn’t check how many elements there are or check that each element isn’t null.
In Chapter 1 we encountered warning CS8600, “Converting null literal or possible null value to non-nullable type,” when assigning Console.ReadLine() to a string . The occurs because Console.ReadLine() returns a string? rather than a non-nullable string . In reality, Console.ReadLine() returns null only if the input is redirected, which isn’t a use case we are expecting in these intro programs, but the compiler doesn’t know that. To avoid the warning, we could use the null-forgiving operator on the Console.ReadLine() return—stating we know better that the value returned won’t be null (and if it is, we are comfortable throwing a null-reference exception).
string text = Console.ReadLine() ! ;
The null-conditional operator is a great feature on its own. However, using it in combination with a delegate invocation resolves a C# pain point that has existed since C# 1.0. Notice in Listing 4.39 how the PropertyChanged event handler is assigned to a local copy ( propertyChanged ) before we check the value for null and finally fire the event. This is the easiest thread-safe way to invoke events without running the risk that an event unsubscribe will occur between the time when the check for null occurs and the time when the event is fired. Unfortunately, this approach is nonintuitive, and frequently developers neglect to follow this pattern—with the result of throwing inconsistent NullReferenceExceptions . Fortunately, with the introduction of the null-conditional operator, this issue has been resolved.
The check for a delegate value changes from what is shown in Listing 4.39 to simply
PropertyChanged?.Invoke(propertyChanged(
this , new PropertyChangedEventArgs(nameof(Age)));
Because an event is just a delegate, the same pattern of invoking a delegate via the null-conditional operator and an Invoke() is always possible.
________________________________________
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key) && !currentPage.some(p => p.level > item.level), }" :href="item.href"> Introduction
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key), }" :href="item.href"> {{item.title}}
- .NET Framework
- C# Data Types
- C# Keywords
- C# Decision Making
- C# Delegates
- C# Constructors
- C# ArrayList
- C# Indexers
- C# Interface
- C# Multithreading
- C# Exception
Null-Coalescing Operator in C#
In C#, ?? operator is known as Null-coalescing operator. It will return the value of its left-hand operand if it is not null. If it is null, then it will evaluate the right-hand operand and returns its result. Or if the left-hand operand evaluates to non-null, then it does not evaluate its right-hand operand.
Here, p is the left and q is the right operand of ?? operator. The value of p can be nullable type, but the value of q must be non-nullable type. If the value of p is null, then it returns the value of q. Otherwise, it will return the value of p.
Important Points:
- The ?? operator is used to check null values and you can also assign a default value to a variable whose value is null(or nullable type).
- You are not allowed to overload ?? operator.
- It is right-associative.
- In ?? operator, you can use throw expression as a right-hand operand of ?? operator which makes your code more concise.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Null Coalescing Operator in C#: Simplifying Null Checks
The null coalescing operator in C# is a useful tool for checking and assigning default values to variables. It simplifies the process of checking for null values and assigning a default value when necessary. The operator is represented by two question marks (??), and it returns the value of its left-hand operand if it is not null, otherwise it evaluates the right-hand operand and returns its result.
Developers can also use the null coalescing assignment operator (??=) to assign a value to a variable only if it is null. This operator is a shorthand way of writing an if statement that checks for null values and assigns a default value if necessary. Overall, the null coalescing operator is a powerful tool in C# that simplifies the process of checking for null values and assigning default values to variables.
Table of Contents
Understanding Null Coalescing Operator in C#
Here, the operator checks if expression1 is null. If it is, then expression2 is assigned to result . If expression1 is not null, then its value is assigned to result .
When used with reference types, the default value is null . For example, if the type is string , the default value is null . In this case, the operator checks if the string is null and assigns a default value if it is.
The Null Coalescing Operator can also be used in combination with the null-conditional operator (?.). This allows developers to check for null values in a chain of expressions. For example:
In summary, the Null Coalescing Operator is a useful operator in C# that simplifies code and makes it more concise. It allows developers to check for null values in expressions and assign default values if necessary.
Working with Null Coalescing Operator
The null coalescing operator (??) is a useful tool in C# for handling null values. It allows developers to specify a default value to use when a variable is null, without having to write lengthy if-else statements.
In this example, if the value variable is null, the result variable will be assigned the value of 0.
The null coalescing operator can also be used in combination with the null-conditional operator (?.) to simplify code even further. The null-conditional operator allows developers to check if an object is null before accessing one of its properties or methods. When used in combination with the null coalescing operator, it allows for concise and easy-to-read code.
It is important to note that the null coalescing operator only works with nullable value types or reference types. It cannot be used with non-nullable value types like int or double.
Overall, the null coalescing operator is a powerful tool for simplifying code and handling null values in C#. By using it in combination with the null-conditional operator, developers can write concise and easy-to-read code that handles null values gracefully.
Null Coalescing Operator and Types
The null coalescing operator can be used with different types of operands, including nullable types and value types. When using the null coalescing operator with nullable types, the operator returns the value of the left-hand operand if it is not null, otherwise it returns the value of the right-hand operand.
When using the null coalescing operator with value types, the operator returns the value of the left-hand operand if it is not equal to the default value of the value type, otherwise it returns the value of the right-hand operand. For example, if the left-hand operand is an integer with a value of 0, and the right-hand operand is an integer with a value of 1, the operator will return 1.
It is important to note that the null coalescing operator cannot be used with non-nullable value types. If a non-nullable value type is used as the left-hand operand, the operator will not compile. However, the operator can be used with nullable value types, which allow the value type to be assigned a null value.
In summary, the null coalescing operator is a useful C# operator that can be used with different types of operands and variables. It allows for concise and readable code that assigns default values to variables when they are null.
Null Coalescing Operator and Extension Methods
Extension methods allow developers to add functionality to existing classes without having to create a new class or modify the existing one. By using extension methods, developers can extend the functionality of the null coalescing operator to work on more complex objects.
For example, an extension method can be created to handle null values on an IEnumerable collection. This method can be used to return an empty collection instead of a null value, making it easier to work with the collection in subsequent code.
Overall, the combination of the null coalescing operator and extension methods can help developers write more efficient and effective code by handling null values more gracefully.
Null Coalescing Operator in SQL
The SQL Coalesce function returns the first non-null expression among its arguments. It is a useful tool for handling null values in SQL queries. The syntax for the Coalesce function is as follows:
The Null Coalescing Operator (??) in C# is similar to the Coalesce function in SQL. It returns the value of its left-hand operand if it is not null, otherwise, it returns the value of its right-hand operand.
In SQL, the Coalesce function is often used in conjunction with the IsNull function to handle null values. The IsNull function returns the first expression if it is not null, otherwise, it returns the second expression.
The Coalesce function can also be used to concatenate strings in SQL. For example, the following SQL query will concatenate the values of column1 and column2, separated by a space.
The above SQL query will return the concatenated value of column1 and column2, separated by a space. If either column1 or column2 is null, it will be replaced by an empty string.
Null Coalescing Operator and Conditional Operators
The null coalescing operator (??) is a useful operator in C# that can be used to provide a default value for a null value. It returns the left-hand operand if it is not null, otherwise, it returns the right-hand operand. This operator can be combined with conditional operators to make code more concise and readable.
The conditional operator (?:) is a ternary operator that evaluates a Boolean expression and returns one of two possible values depending on the result of the evaluation. It is right-associative, meaning that the right operand is evaluated before the left operand. This operator can be used to provide a default value for a null value, but it requires more code than the null coalescing operator.
The FirstOrDefault method is a LINQ extension method that returns the first element of a sequence or a default value if the sequence is empty. This method can be used in combination with the null coalescing operator to provide a default value for a null value.
The ValueOrDefault method is a method that returns the value of a nullable value type or a default value if the value is null. This method can be used in combination with the null coalescing operator to provide a default value for a null value.
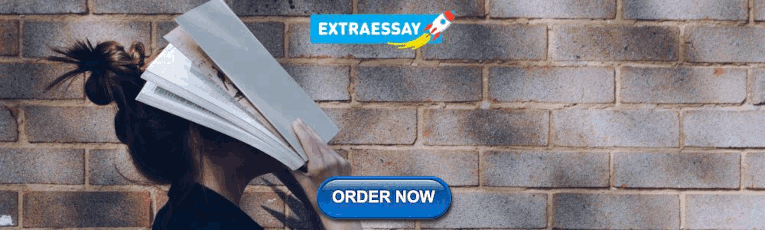
Null Coalescing Operator and Overloading
The null coalescing operator ?? in C# is a shorthand way of checking if a value is null and providing a default value if it is. It is often used to simplify code and make it more concise.
In C#, the null coalescing operator can be overloaded to provide additional functionality. Overloading the null coalescing operator allows the developer to define custom behavior when using the operator with different types of operands.
An example of overloading the null coalescing operator in C# is shown below:
In the example above, the null coalescing operator is overloaded for the int? and int types. The operator returns the value of the left-hand operand if it is not null, otherwise it returns the value of the right-hand operand.
In addition to overloading the null coalescing operator, C# also provides the null-conditional operators ?. and ?[] , which can be used to safely access members of an object or array that may be null.
Overall, the null coalescing operator and overloading can be useful tools for simplifying code and providing custom behavior when working with nullable types in C#.
Related posts:
Leave a comment cancel reply.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Null coalescing assignment
- 6 contributors
This article is a feature specification. The specification serves as the design document for the feature. It includes proposed specification changes, along with information needed during the design and development of the feature. These articles are published until the proposed spec changes are finalized and incorporated in the current ECMA specification.
There may be some discrepancies between the feature specification and the completed implementation. Those differences are captured in the pertinent language design meeting (LDM) notes .
You can learn more about the process for adopting feature speclets into the C# language standard in the article on the specifications .
Simplifies a common coding pattern where a variable is assigned a value if it is null.
As part of this proposal, we will also loosen the type requirements on ?? to allow an expression whose type is an unconstrained type parameter to be used on the left-hand side.
It is common to see code of the form
This proposal adds a non-overloadable binary operator to the language that performs this function.
There have been at least eight separate community requests for this feature.
Detailed design
We add a new form of assignment operator
Which follows the existing semantic rules for compound assignment operators ( §11.18.3 ), except that we elide the assignment if the left-hand side is non-null. The rules for this feature are as follows.
Given a ??= b , where A is the type of a , B is the type of b , and A0 is the underlying type of A if A is a nullable value type:
- If A does not exist or is a non-nullable value type, a compile-time error occurs.
- If B is not implicitly convertible to A or A0 (if A0 exists), a compile-time error occurs.
- If A0 exists and B is implicitly convertible to A0 , and B is not dynamic, then the type of a ??= b is A0 . a ??= b is evaluated at runtime as: var tmp = a.GetValueOrDefault(); if (!a.HasValue) { tmp = b; a = tmp; } tmp Except that a is only evaluated once.
- Otherwise, the type of a ??= b is A . a ??= b is evaluated at runtime as a ?? (a = b) , except that a is only evaluated once.
For the relaxation of the type requirements of ?? , we update the spec where it currently states that, given a ?? b , where A is the type of a :
If A exists and is not a nullable type or a reference type, a compile-time error occurs.
We relax this requirement to:
- If A exists and is a non-nullable value type, a compile-time error occurs.
This allows the null coalescing operator to work on unconstrained type parameters, as the unconstrained type parameter T exists, is not a nullable type, and is not a reference type.
As with any language feature, we must question whether the additional complexity to the language is repaid in the additional clarity offered to the body of C# programs that would benefit from the feature.
Alternatives
The programmer can write (x = x ?? y) , if (x == null) x = y; , or x ?? (x = y) by hand.
Unresolved questions
- [ ] Requires LDM review
- [ ] Should we also support &&= and ||= operators?
Design meetings
C# feature specifications
Additional resources
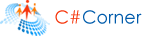
- TECHNOLOGIES
- An Interview Question

Null Coalescing Operator (??) in C#
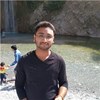
- Shubham Jain
- Jan 11, 2018
Null Coalescing Operator (??)1) A syntax used to check null and assign a default value if null2) This operator can be used with Nullable types as well as reference types.3) This operator can be represented as x ?? y. Here, x and y are left and right operand. Left operand(x) can be nullable type while right operand should have non-nullable value. If the value of x is null then the syntax return the value of y else value of x will be returned. Means value of left operand will be returned if its value is not null else the value of right operand will be returned.Why should we use Null Coalescing Operator (??)?There are numerous of uses/advantages of Null Coalescing Operator:1) It helps in preventing InvalidOperationException. 2) It helps in defining a default value for a nullable item as we did in above program.(assigning a default value 33)3) It removes many redundant "if-else" conditions and makes the code more organized and readable.
- A syntax used to check null and assign a default value if null
- This operator can be used with Nullable types as well as reference types.
- This operator can be represented as x ?? y.
- using System;
- namespace Tutpoint
- {
- class Program
- {
- static void Main( string [] args)
- {
- int ? a = null ;
- int ? b = a.Value;
- Console.ReadKey();
- }
- }
- }
- int ? b = a ?? 33;
- Console.WriteLine( "Value of a= " + a + " and b= " + b);
- int ? b;
- if (a.HasValue)
- {
- b = a;
- }
- else
- b = 33;
- class Program
- {
- // String is reference type
- string str1 = null ;
- string str2 = "Hello" ;
- string str3 = "Buddy" ;
- string str4 = str1 ?? str2;
- str3 = str4 ?? str2;
- // Value type
- bool ? c = null ;
- Program p = new Program();
- bool ? b = c ?? p.IsValue(11, 33);
- Console.WriteLine( "str3= " + str3 + " , str4= " + str4 + " b=" + b);
- public Nullable< bool > IsValue( int ? num1, int ? num2)
- try
- if (num1.HasValue && num2.HasValue)
- {
- return true ;
- }
- else
- return false ;
- catch (Exception)
- return null ;
- int ? num1 = null ;
- int ? num2 = null ;
- int ? num3 = 3;
- int ? num4 = num1 ?? num2 ?? num3;
- Console.WriteLine( "Value of num4 = " + num4);
- Null Coalescing Operator
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
null coalescing operator in return statements- c#
I have used null coalescing operator in return statements like below
But the below code,
I could not understand how it works, since the second parameter to the operator is a assignment statement and i wonder how the return works.
could some one help me understand
- null-coalescing-operator

- The return-value of an assignement is the value being assigned, so Variable = "undefined" returnes "undefined" . This can then be returned by your method. – MakePeaceGreatAgain Commented Oct 23, 2018 at 15:35
- The second line of code is both assigning a value to Variable if it's null, and returning the value of Variable . It's the same as: if (Variable == null) Variable = "undefined"; return Variable; This is different than the first line, which will return "undefined" if Variable is null , but will not assign that value to Variable (it will remain null after the return). – Rufus L Commented Oct 23, 2018 at 15:41
- Possible duplicate of Why do assignment statements return a value? – gunr2171 Commented Oct 23, 2018 at 15:59
- While I do not recommend it, something like A = B = 2; is valid code, and is the same reason why this works. – Ron Beyer Commented Oct 23, 2018 at 16:03
2 Answers 2
From the docs :
The assignment operator (=) stores the value of its right-hand operand in the storage location, property, or indexer denoted by its left-hand operand and returns the value as its result
So the return-value of an assignement is the value being assigned. Variable = "undefined" therefor returns "undefined" . This can then be returned by your method. The ?? on the other hand is just a shorthand for a simple if-statement.
So the following is fairly similar to your code:
- Does that mean i can do assignment to a variable not the same as the variable mentioned in the left hand side of the operator? – Samuel A C Commented Oct 23, 2018 at 15:39
- @SamuelAC Sure, try it out. As assignement is just a statement as any other. – MakePeaceGreatAgain Commented Oct 23, 2018 at 15:39
- I get it, Thanks – Samuel A C Commented Oct 23, 2018 at 15:43
In C# the assign operation also returns the value that was assigned. For example
Is valid code. The Assignment get's evaluated first from right to left. In your case assignment>null coalescing operator. You coudl rewrite your code to
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# null-coalescing-operator or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- Miracle Miracle Octad Generator Generator
- What Christian ideas are found in the New Testament that are not found in the Old Testament?
- how replicate this effect with geometry nodes
- Everyone hates this Key Account Manager, but company won’t act
- How to justify a ban on single-deity religions?
- Is there anything that stops the majority shareholder(s) from destroying company value?
- Efficiently tagging first and last of each object matching condition
- Looking for title of a Ursula K. Le Guin story/novel that features four-way marriage/romantic relationships
- Are automorphisms of matrix algebras necessarily determinant preservers?
- Can a "sharp turn" on a trace with an SMD resistor also present a risk of reflection?
- What does 北渐 mean?
- Print tree of uninstalled dependencies and their filesizes for apt packages
- What's the counterpart to "spheroid" for a circle? There's no "circoid"
- Can objective morality be derived as a corollary from the assumption of God's existence?
- If physics can be reduced to mathematics (and thus to logic), does this mean that (physical) causation is ultimately reducible to implication?
- If you get pulled for secondary inspection at immigration, missing flight, will the airline rebook you?
- Are there any virtues in virtue ethics that cannot be plausibly grounded in more fundamental utilitarian principles?
- Op Amp Feedback Resistors
- Idiomatic alternative to “going to Canossa”
- Do cities usually form at the mouth of rivers or closer to the headwaters?
- grep command fails with out-of-memory error
- Explaining Arithmetic Progression
- How to determine if a set is countable or uncountable?
- Fitting 10 pieces of pizza in a box
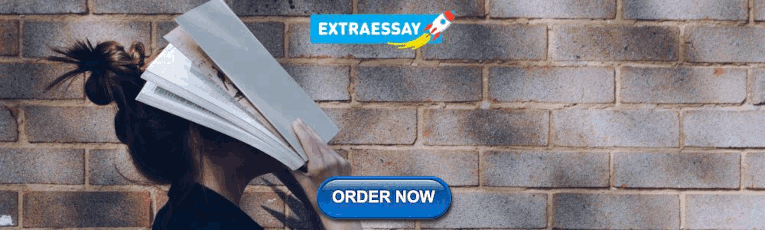
IMAGES
COMMENTS
The null-coalescing operator ?? returns the value of its left-hand operand if it isn't null; otherwise, it evaluates the right-hand operand and returns its result. The ?? operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null.
In C#, ?? operator is known as Null-coalescing operator. It will return the value of its left-hand operand if it is not null. If it is null, then it will evaluate the right-hand operand and returns its result. Or if the left-hand operand evaluates to non-null, then it does not evaluate its right-hand operand. Syntax: p ?? q Here, p is the left and
Nulls in C#. One of the new C# features allows us to get rid of nulls in our code with nullable reference types. We are encouraged to add. to the project file due to problems like described here. Of course, a lot of existing projects don't want to add this. Many, many errors need to be solved when enabling this functionality, so a lot of legacy ...
Here, in this article, I try to explain Null-Coalescing Assignment in C# 8 with Examples. I hope you enjoy this Null-Coalescing Assignment in C# with Examples article. Dot Net Tutorials. About the Author: Pranaya Rout. Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft ...
In this article, we will learn how to use Null-coalescing assignment operator in C# and also check the updated requirements of Null-coalescing operator requirements.
In previous versions of C# like 7.3 we were using other different techniques to do the same task as performed by Null-coalescing assignment operator??. For instance, I'm using Null-coalescing operator to do the same but it seems a little bit cheap.
Else the null-coalescing operator returns its second value. This way the null-coalescing operator has the following logic: "If the first operand is something else than null, return it; otherwise, give the default value specified with the second operand". The null-coalescing operator, however, does not check whether it's second value is ...
Mastering the Null-Coalescing Operator in C#. Handling null values efficiently is a common requirement in software development. C# offers powerful tools to manage nulls, including the null-coalescing operator (??). This article explores the null-coalescing operator, its benefits, and how it can simplify and enhance your code.
csharp-8 documentation: Null-coalescing Assignment. The null-coalescing operator ?? returns the value of its left-hand operand if it is not a null value. If it is null, then it evaluates the right-hand operand and returns its result.
C# 8.0 provides a combination of the null-coalescing operator and the assignment operator with the addition of the null-coalescing assignment operator. With this operator, you can evaluate if the left-hand side is null and assign the value on the righthand side if it is. Listing 4.37 uses this operator when assigning fullName.
In C#, ?? operator is known as Null-coalescing operator. It will return the value of its left-hand operand if it is not null. If it is null, then it will evaluate the right-hand operand and returns its result. Or if the left-hand operand evaluates to non-null, then it does not evaluate its right-hand operand. Syntax: p ?? q. Here, p is the left ...
The null coalescing operator is often used in situations where a default value needs to be assigned to a variable if the original value is null. It is a concise way of writing an if-else statement that checks for null values. The operator is especially useful when working with nullable value types, as it eliminates the need for additional null ...
This allows the null coalescing operator to work on unconstrained type parameters, as the unconstrained type parameter T exists, is not a nullable type, and is not a reference type. Drawbacks As with any language feature, we must question whether the additional complexity to the language is repaid in the additional clarity offered to the body ...
The null coalescing operator (??) in C# is a convenient way to handle null values in expressions. It checks whether its left-hand operand is null and, if so, evaluates and returns the right-hand ...
Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand; OverflowAI GenAI features for Teams; OverflowAPI Train & fine-tune LLMs; Labs The future of collective knowledge sharing; About the company Visit the blog
Introduction. In today's article we will look at two new features introduced with C# 8.0.The first is called the Null-coalescing assignment feature and the second is the enhancement to interpolated verbatim strings. C# 8.0 is supported on .NET Core 3.x and .NET Standard 2.1.
Null Coalescing Assignment in C# 8. Null coalescing assignment is a feature introduced in C# 8 that simplifies the assignment of values when dealing with potentially null variables. It allows you to assign a value to a variable only if it is null, providing a more concise and readable syntax.
The null-coalescing operator results in Callback being evaluated just once. Delegates are immutable: Combining operations, such as Combine and Remove, do not alter existing delegates. Instead, such an operation returns a new delegate that contains the results of the operation, an unchanged delegate, or null.
The null coalescing operator was introduced in C# 7.0. This operator does not even evaluate the right-hand operand if the left-hand operator is not null. Example
Null Coalescing Operator (??)1) A syntax used to check null and assign a default value if null2) This operator can be used with Nullable types as well as reference types.3) This operator can be represented as x ?? y. Here, x and y are left and right operand. Left operand (x) can be nullable type while right operand should have non-nullable value.
3. In C# the assign operation also returns the value that was assigned. For example. Value=Value=Value=Value="Hello World". Is valid code. The Assignment get's evaluated first from right to left. In your case assignment>null coalescing operator. You coudl rewrite your code to. string returnValue="";