A Guide To 3-Tier Architecture

A well-structured and organized solution is crucial for maintainability and scalability in software development. The 3-Tier architecture clarifies concerns, allowing developers to manage the application logic, data access, and presentation layers separately. In this article, we will explore the benefits of this architecture and learn how to implement it in a Visual Studio solution using C#.
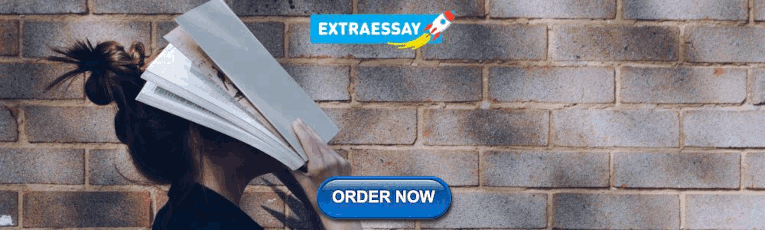
Different Architectures
There are many types of architectures you can use for your applications. You just have to find the one that suits your needs. I like the 3-tier architecture. It’s small, simple, and easy to create. But in some cases, I might change it because of my needs.
There are 9 known architectures we use these days in C#:
- Monolithic architecture
- Microservices architecture
- 3-tier architecture
- Model-View-Controller (MVC) architecture
- Model-View-Presenter (MVP) architecture
- Model-View-ViewModel (MVVM) architecture
- Event-Driven architecture
- Service-Oriented Architecture (SOA)
- Client-Server architecture
I am not going to explain them all. It will become a very large page. But the top 3 popular architectures are:
- Microservices architecture A way to break down a large and complex application into smaller, independent services that are connected. They communicate with each other through APIs. This architecture is becoming popular with bigger projects. You can maintain and deploy the smaller projects more easily.
- 3-tier architecture This type of architecture separates an application into three logical components: the presentation layer, the application layer, and the data layer. This separation of concerns provides better scalability, maintainability, and ease of development.
- Model-View-Controller (MVC) architecture Well-known if you have created web applications and/or APIs with C#. It is a design that separates the application logic from the user interface. The Model component represents the data and the business logic, the View component displays the data, and the Controller component handles user input and updates the Model. This separation of concerns provides a more organized and maintainable codebase.
This tutorial explains the 3-tier architecture mainly because we use this one the most.
The 3-Tier Architecture
This architecture separates the application into three logical components. These three layers help to maintain the code and separation of concern. But for me, it has a different reason I use this architecture: reusability.
Especially at a time when you might want to create a Windows, macOS, Android, Linux, and iOS app that does the same thing. Do you create the same class over and over again? No, you create a class library with the logic and reference that library in all the projects that need the logic. If something changes in the class library, all other projects get updated.
Three Different Layers
Each layer has its own responsibility but only talks to one other layer. The presentation layer only talks to the application layer and the application layer only talks to the data layer. That means that if you want to show a list of movies, for example, you need to ask the application layer which will ask the data layer. The application layer will send the information of the data layer to the presentation layer. The presentation layer never talks to the data layer directly.

The Data Layer
The first layer I am discussing is the data layer. Maybe the name already tells you what this layer is about: Data. It could be data from a database or a file. Generally speaking, we use this layer to transport data from and to a data source.
This layer does not contain any form of logic. It just sends or retrieves data from the data source. Nothing special. Logic is placed in the application layer.
If you have multiple data sources you need to manage, you can create multiple data layers and bring them together in the application by using dependency injection.
The data layer is a layer that is disappearing a bit. Before we had ORMs, like Entity Framework , we had to write a lot of code to retrieve and send data from and to a database. With Entity Framework, we don’t need that anymore. That is the reason the logic for the database is moving to the application layer.
The Application Layer
This layer is the beating heart for all your logic. Here you combine logic with the data from the data layer. This layer is responsible for calculations and decisions before sending the data to your presentation layer.
The application layer is often called the business layer. This is a small heritage from the time we had the DLL (data layer library) and the BLL (business layer library). In my projects, I usually call it the business.
For example, the presentation layer requests a list of movies, ordered by title. The application layer will retrieve all the movies from the data layer and sort them by title.
This layer does nothing with the data source directly. The reason is simple: The application layer should never know what kind of data source you use. If you implement dependency injection, the data source can change. Even the whole data layer can change (except for the interface). But the application layer should always be working without changing it.
You can have multiple application layers. I created a logger once. A simple logger that stores messages in a file. But later I needed a logger that stored the messages in a database. I didn’t want two loggers in the single class library representing my application layer. So I created a second application layer for the logger.
It’s usually this layer we unit test because it contains the most important code of our application.
The Presentation Layer
And last but not least; the presentation layer. This layer is what a user sees. It could be a console application, WinForms, or an API. It’s the one that gives output that a user or a client application can work with. This layer is also referred to as the top-most layer in this architecture.
It is responsible for presenting the data from the application layer in a user-friendly way. Examples of user-friendly are web pages, WinForms, and mobile apps.
A console application or a WinForms application has a clear GUI. You can click on stuff and it works. An API doesn’t have that. But it does expose JSON (or XML if you want). An API is considered a front-end application.
Conclusion On A Guide to 3-Tier Architecture
The 3-tier architecture is the most used because it’s easy to understand and implement. I encourage you to use this if you just start or create a small project. If you have a really, really small project; make your life easier, and don’t use layers.
The idea behind these layers is to separate data, logic, and application. I think the names are a bit confusing and most people think the application layer is the front end, but that’s the presentation layer. And that is why I call the application layer the business layer.
Related Articles

Learn C# Essentials and Frameworks for New Developers

Learn C# – Part 9: Basic Structure And A New Project

- Kens Learning Curve
ChatGPT: Yay or nay?
10 reasons why you should unit test, you may also like, visual studio shortcuts, design patterns and design principles, to savechanges or not to savechanges, top 5 reasons to use c#, how to apply clean code with c#, the c# data types part 2 – int, the c# data types part 1 – string, the use of code comments.
Kens Learning Curve is all about learning C# and all that comes along with it. From short, practical tutorials to learning from A to Z.
All subjects are tried and used by myself and I don’t use ChatGPT to write the texts.
Useful links
- Free Tutorials
- C# Bootcamp
Use the contact form to contact me or send me an e-mail.
The contact form can be found here .
Email: [email protected]
@2023 – All Right Reserved. Designed and Developed by Kens Learning Curve
- Free C# Information Webinar
- Online Courses
- Kens Learning Paths
- Technical Writer
- Career Advise
GET THE LATEST TUTORIALS AND NEWS!
Like what you are seeing.
Make sure you don’t miss our latest tutorials and courses! Subscribe to our updates. We won’t spam you, promised.
- Software design and development
3-tier application architecture
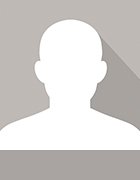
- TechTarget Contributor
What is a 3-tier application?
A 3-tier application architecture is a modular client-server architecture that consists of a presentation tier, an application tier and a data tier. The data tier stores information, the application tier handles logic and the presentation tier is a graphical user interface ( GUI ) that communicates with the other two tiers. The three tiers are logical, not physical, and may or may not run on the same physical server.
The logical tiers of a 3-tier application architecture
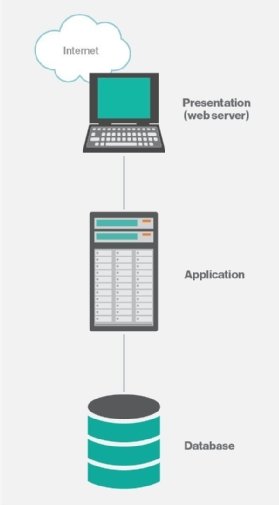
Presentation tier : This tier, which is built with HTML5, cascading style sheets ( CSS ) and JavaScript , is deployed to a computing device through a web browser or a web-based application. The presentation tier communicates with the other tiers through application program interface ( API ) calls.
Application tier : The application tier, which may also be referred to as the logic tier, is written in a programming language such as Java and contains the business logic that supports the application's core functions. The underlying application tier can either be hosted on distributed servers in the cloud or on a dedicated in-house server, depending on how much processing power the application requires.
Data tier : The data tier consists of a database and a program for managing read and write access to a database. This tier may also be referred to as the storage tier and can be hosted on-premises or in the cloud. Popular database systems for managing read/write access include MySQL , PostgreSQL, Microsoft SQL Server and MongoDB .
Benefits of a 3-tier app architecture
The benefits of using a 3-tier architecture include improved horizontal scalability, performance and availability. With three tiers, each part can be developed concurrently by a different team of programmers coding in different languages from the other tier developers. Because the programming for a tier can be changed or relocated without affecting the other tiers, the 3-tier model makes it easier for an enterprise or software packager to continually evolve an application as new needs and opportunities arise. Existing applications or critical parts can be permanently or temporarily retained and encapsulated within the new tier of which it becomes a component.
3-tier application programs may also be referred to as n-tier programs. In this context, the letter n stands for "a number of tiers."
Continue Reading About 3-tier application architecture
- A quick rundown of 3 layered architecture design styles
- Enterprise design: Is three-tiered architecture still useful?
- What is 2nd and 3rd tier Middleware?
- Adopting a 4-tier architecture for mobile solutions
- Web app architectures overview
Related Terms
Dig deeper on software design and development.
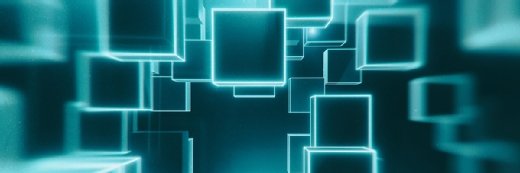
Data center tiers and why they matter for uptime
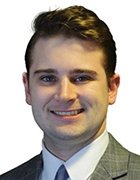
Cloud storage: Key storage specifications
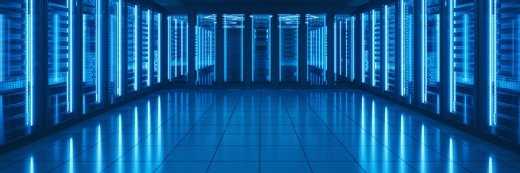
Choose the best Azure Blob Storage tier
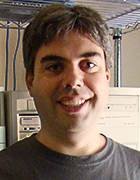
AWS shakes up cloud storage pricing, expands Free Tier
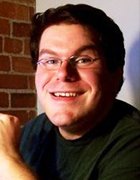
While it's tough to make a clear distinction between cloud-native, cloud-based and cloud-enabled apps, they differ in terms of ...
Receiving a FinOps certification helps individuals develop their cloud financial management skills. Discover training and ...
With Terraform, developers can lean on familiar coding practices to provision the underlying resources for their applications. ...
Authorization is a critical security component of a microservices architecture. Follow these five guiding principles to deploy ...
Managing microservices without API gateways might be uncommon, but not unheard of. Consider the benefits, downsides and available...
The switch from microservices to monolith could save costs and improve performance. Explore key considerations and questions to ...
Terraform can save you time and headaches when deploying servers with cloud providers. Get up to speed on Terraform basics in ...
The k-means clustering algorithm groups similar objects or data points into clusters. This approach is popular for multivariate ...
Datadog is reportedly a suitor for GitLab; existing users understand the rationale for such a deal, but key questions must be ...
Generic variables give the TypeScript language versatility and compile-time type safety that put it on par with Java, C# and C++....
Does the world really need another programming language? Yes, say developers behind Zig. Here are five of the top features Zig ...
Virtual threads in Java currently lack integration with the stream API, particularly for parallel streams. Here's how a JDK 22 ...
Compare Datadog vs. New Relic capabilities including alerts, log management, incident management and more. Learn which tool is ...
Many organizations struggle to manage their vast collection of AWS accounts, but Control Tower can help. The service automates ...
There are several important variables within the Amazon EKS pricing model. Dig into the numbers to ensure you deploy the service ...

DEV Community

Posted on Apr 3
Understanding Layers, Tiers, and N-Tier Architecture in Application Development
In the realm of application development, the architecture of an application significantly impacts its performance, scalability, and ease of maintenance. Developers often talk about "layers" and "tiers" within an application's architecture, terms that are crucial but sometimes misunderstood.
In this article, we will clarify these concepts and delve into the N-tier architecture, focusing on its 3-tier and 4-tier models.
Layers vs. Tiers : What's the Difference?
Although "layers" and "tiers" are often used interchangeably, they refer to different aspects of application architecture:
- Layers are virtual separations within an application that organize its different parts, such as the presentation layer (UI) , the business logic layer , and the data access layer .
These layers exist within the application's code structure and are a logical way to separate responsibilities and functionalities.
- Tiers , on the other hand, refer to the physical distribution of an application's components across different servers or platforms. This physical separation is crucial for an application's scalability, security, and performance. When components are deployed on separate servers and still communicate effectively, they form what is known as a tiered architecture.
Introducing N-Tier Architecture:
N-tier architecture is a scalable and flexible way to structure applications, where "N" represents the number of tiers involved. This architecture divides the application into multiple tiers, each responsible for specific aspects of the application's functionality.

3-Tier Architecture (The Most Common Model):
The 3-tier architecture is a widely adopted model in application development, consisting of the following tiers:
Presentation Tier Tier: This is the user interface (UI) layer, where users interact with the application. It's built using technologies like HTML , CSS , JavaScript , and frameworks like React or Angular .
Application Tier : The core of the application, this layer contains business rules and logic. It acts as an intermediary between the presentation and data access layers, ensuring that user inputs are processed and validated. Technologies used include server-side languages like Java , C# , Python , and frameworks like .NET , Spring , or Django .
Data Tier : This layer is responsible for managing the application's data. This layer handles communication with databases or other storage mechanisms, providing CRUD (Create, Read, Update, Delete) operations. It uses technologies like JDBC, Entity Framework, or ORM.
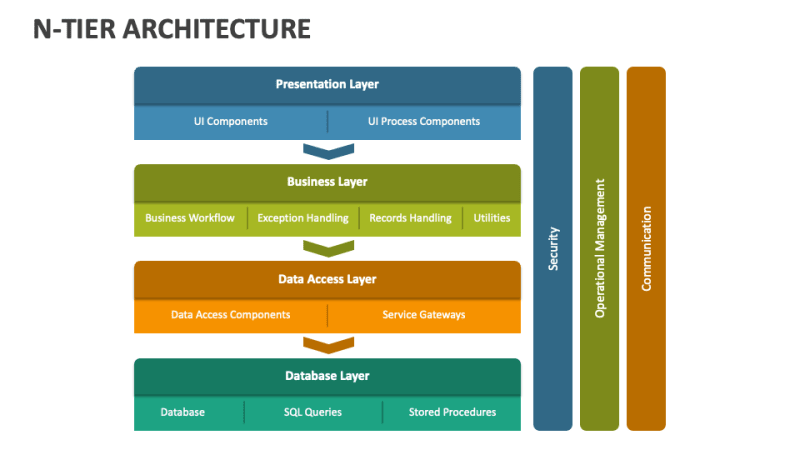
4-Tier Architecture:
This additional layer abstracts the business logic's external communication, making the system more modular and enabling services to be reused across different parts of the application or even across different applications. It's particularly useful in microservices and service-oriented architectures.
In more complex applications, a 4-tier architecture may be employed, which adds an additional tier:
Delivery Tier : This tier focuses on caching and delivering front-end assets to the client, often through a Content Delivery Network ( CDN ).
The CDN enhances the application's performance and user experience by storing and serving static content from locations closest to the user.
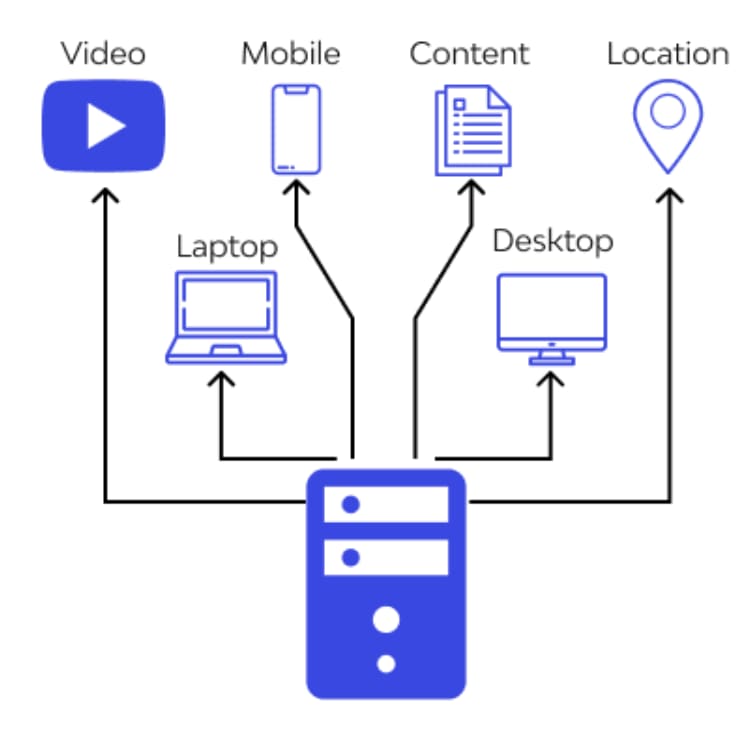
Benefits of N-Tier Architecture:
Scalability : Separating an application into tiers allows for easier scaling of each component independently.
Security : It becomes easier to implement security measures, as each tier can have its own security protocols.
Maintainability : Updates and bug fixes can be carried out more efficiently since changes in one tier do not necessarily affect others.
Efficiency : The development process is streamlined, as developers can focus on specific aspects of the application within each tier.
Top comments (0)
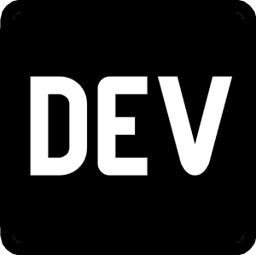
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Are Midjourney Images Free To Use?
Devops Den - Jul 5

How To Host A Static Website In Azure Blob Storage
Chidera Enyelu - Jul 5

Hybrid Rendering Architecture using Astro and Go Fiber
YURIIDE - Jul 6
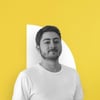
OAuth vs. JWT: Ultimate Comparison
Ege Aytin - Jul 25
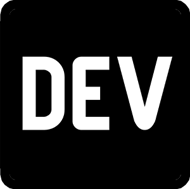
We're a place where coders share, stay up-to-date and grow their careers.
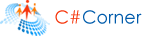
- TECHNOLOGIES
- An Interview Question
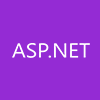
Understand 3-Tier Architecture in C#

- Sourav Kayal
- Jan 18, 2021
- Other Artcile
In this article we will learn to implement 3- Tier architecture in C#.NET application.
Why 3-Tier Architecture?
Difference between tier and layer, what are the layers, presentation layer/ ui layer.
- Business Layer
- Data Access Layer
Code for Data Access Layer
- using System;
- using System.Collections.Generic;
- using System.Data;
- using System.Data.SqlClient;
- namespace WindowsFormsApplication1.DAL
- {
- public class PersonDAL
- {
- public string ConString = "Data Source=SOURAV-PC\\SQL_INSTANCE;Initial Catalog=test;Integrated Security=True" ;
- SqlConnection con = new SqlConnection();
- DataTable dt = new DataTable();
- public DataTable Read()
- {
- con.ConnectionString = ConString;
- if (ConnectionState.Closed == con.State)
- con.Open();
- SqlCommand cmd = new SqlCommand( "select * from Person" ,con);
- try
- {
- SqlDataReader rd = cmd.ExecuteReader();
- dt.Load(rd);
- return dt;
- }
- catch
- throw ;
- }
- public DataTable Read(Int16 Id)
- SqlCommand cmd = new SqlCommand( "select * from Person where ID= " + Id + "" , con);
- }
Create a Business Logic Layer
- using WindowsFormsApplication1.DAL;
- namespace WindowsFormsApplication1.BLL
- public class PersonBLL
- public DataTable GetPersons()
- PersonDAL objdal = new PersonDAL();
- return objdal.Read();
- public DataTable GetPersons(Int16 ID)
- return objdal.Read(ID);
Create a Presentation Layer
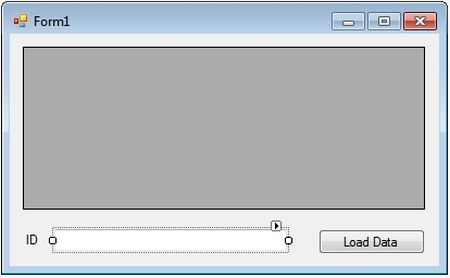
- using System.ComponentModel;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- using WindowsFormsApplication1.BLL;
- namespace WindowsFormsApplication1
- public partial class Form1 : Form
- public Form1()
- InitializeComponent();
- private void button1_Click( object sender, EventArgs e)
- PersonBLL p = new PersonBLL();
- this .dataGridView1.DataSource = p.GetPersons(Convert.ToInt16( this .txtID.Text));
- MessageBox.Show( "Error Occurred" );
- private void Form1_Load( object sender, EventArgs e)
- this .dataGridView1.DataSource = p.GetPersons();
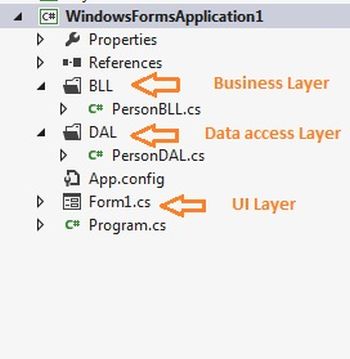
- 3- Tier architecture
- Presentation Layer
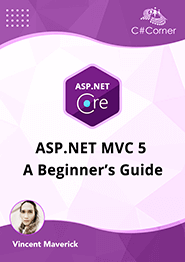
ASP.NET MVC 5: A Beginner’s Guide
Java Guides
Search this blog, three tier (three layer) architecture in spring mvc web application.
Learn Spring MVC at https://www.javaguides.net/p/spring-mvc-tutorial.html
1. Three Tier (Three Layer) Architecture
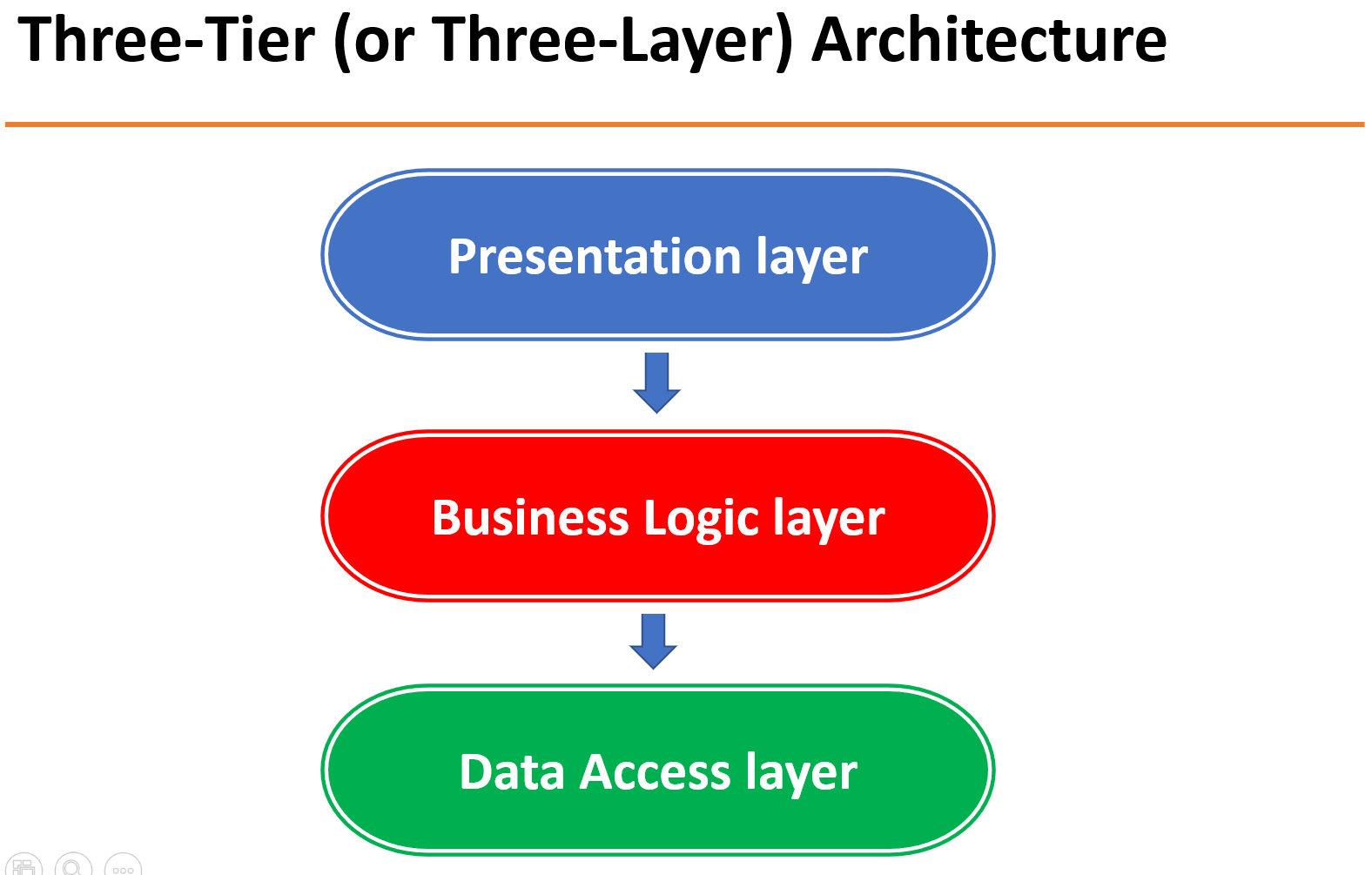
2. Three Tier (Three Layer) Architecture VS MVC Pattern
- The Model Layer - This is the data layer which contains the business logic of the system, and also represents the state of the application. It’s independent of the presentation layer, the controller fetches the data from the Model layer and sends it to the View layer.
- The Controller Layer - The controller layer acts as an interface between View and Model . It receives requests from the View layer and processes them, including the necessary validations.
- The View Layer - This layer represents the output of the application, usually some form of UI. The presentation layer is used to display the Model data fetched by the Controller .
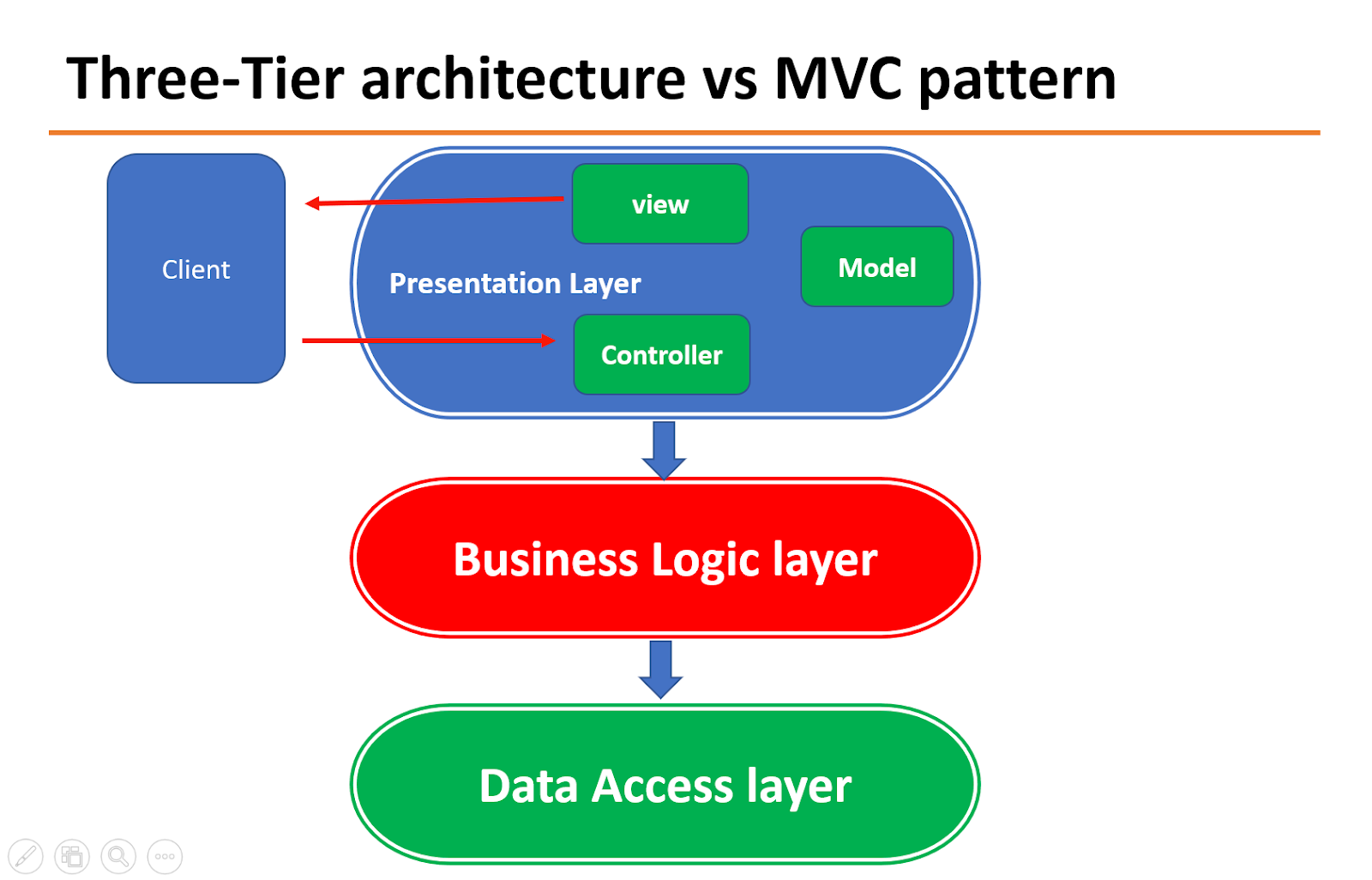
3. How to use Three-layer architecture in Spring MVC web applications.

- Controller classes as the presentation layer. Keep this layer as thin as possible and limited to the mechanics of the MVC operations, e.g., receiving and validating the inputs, manipulating the model object, returning the appropriate ModelAndView object, and so on. All the business-related operations should be done in the service classes. Controller classes are usually put in a controller package.
- Service classes as the business logic layer. Calculations, data transformations, data processes, and cross-record validations (business rules) are usually done at this layer. They get called by the controller classes and might call repositories or other services. Service classes are usually put in a service package.
- Repository classes as data access layer. This layer’s responsibility is limited to Create, Retrieve, Update, and Delete (CRUD) operations on a data source, which is usually a relational or non-relational database. Repository classes are usually put in a repository package.
- Presentation Layer - controller package
- Business Logic Layer - service package
- Data Access Layer - repository package
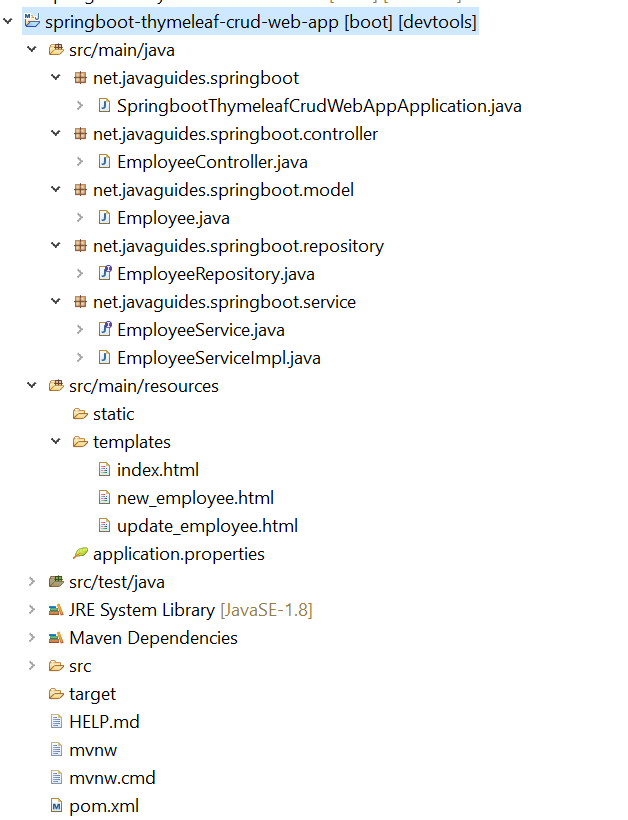
Read more about MVC pattern at Model View Controller (MVC) Design Pattern in Java
Post a Comment
Leave Comment
My Top and Bestseller Udemy Courses
- Spring 6 and Spring Boot 3 for Beginners (Includes Projects)
- Building Real-Time REST APIs with Spring Boot
- Building Microservices with Spring Boot and Spring Cloud
- Full-Stack Java Development with Spring Boot 3 & React
- Testing Spring Boot Application with JUnit and Mockito
- Master Spring Data JPA with Hibernate
- Spring Boot Thymeleaf Real-Time Web Application - Blog App
Check out all my Udemy courses and updates: Udemy Courses - Ramesh Fadatare
Copyright © 2018 - 2025 Java Guides All rights reversed | Privacy Policy | Contact | About Me | YouTube | GitHub

- .NET Framework
- C# Data Types
- C# Keywords
- C# Decision Making
- C# Delegates
- C# Constructors
- C# ArrayList
- C# Indexers
- C# Interface
- C# Multithreading
- C# Exception
What is .NET 3-Tier Architecture?
Three-layer architecture is dividing the project into three layers that are User interface layer , business layer and data(database) layer where we separate UI, logic, and data in three divisions. Suppose the user wants to change the UI from windows to the phone than he has to only make change in UI layer, other layers are not affected by this change. Similarly, if the user wants to change the database then he has to only make a change in the data layer, rest everything remains the same.
Why to use 3-Tier Architecture in Projects?
We use 3 Tier architecture to manage large projects. We can take an example: Suppose you have created 2000 entity code and you have coded all your code in only one layer. Now suppose you want to change from windows application to mobile or web application then again you have to write code for all 2000 entities or you want to change database provider than again you have to change database connection in all 2000 entities. Hence writing such a large amount of code is a time and money wasting. Also writing a large amount of code in one layer only makes new people difficult to understand that code. So, to provide maintenance, flexibility, updation flexibility without affecting other parts, clean and understandable code we should use 3-Tier Architecture in Projects. This architecture basically contains three layers :
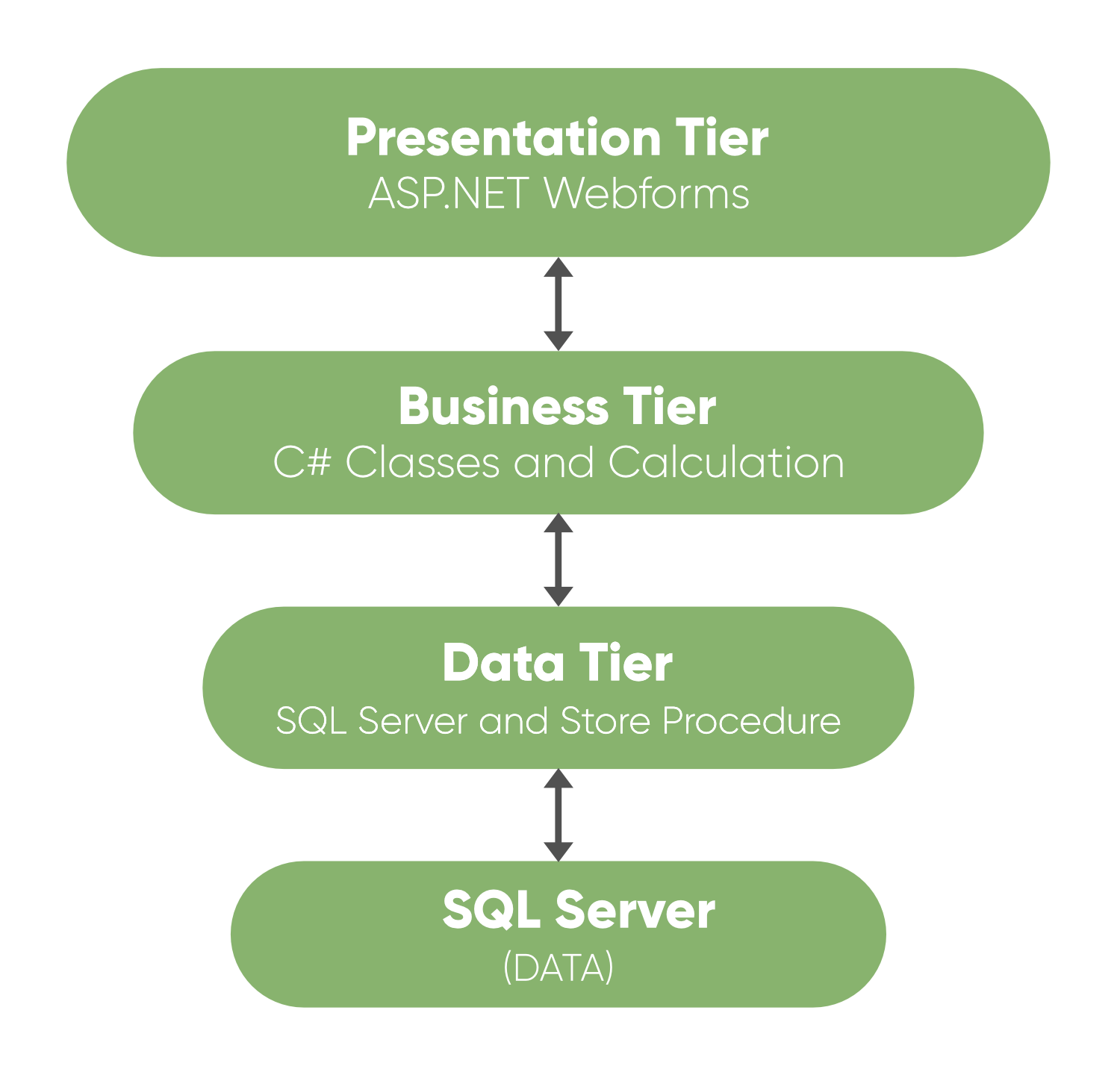
1. Presentation Layer
This is the top layer of architecture. The topmost level of application is the user interface. It is related to the user interface that is what the user sees. The main function of this layer is to translate tasks and results in something which the user can understand. It contains pages like web forms, windows form where data is presented to the user and use to take input from the user. The presentation layer is the most important layer because it is the one that the user sees and good UI attracts the user and this layer should be designed properly.
2. Business Layer
This is the middle layer of architecture. This layer involves C# classes and logical calculations and operations are performed under this layer. It processes the command, makes logical decisions and perform calculations. It also acts as a middleware between two surrounded layers that is presentation and data layer. It processes data between these two layers. This layer implements business logic and calculations. This layer also validates the input conditions before calling a method from the data layer. This ensures the data input is correct before proceeding, and can often ensure that the outputs are correct as well. This validation of input is called business rules.
3. Data Layer
This layer is used to connect the business layer to the database or data source. It contains methods which are used to perform operations on database like insert, delete, update, etc. This layer contains stored procedures which are used to query database. Hence this layer establishes a connection with the database and performs functions on the database.
Advantages:
- Updation to new graphical environments is easier and faster.
- Easy to maintain large and complex projects.
- It provides logical separation between presentation layer, business layer and data layer.
- By introducing application layer between data and presentation layer, it provides more security to database layer hence data will be more secure.
- You can hide unnecessary methods from the business layer in the presentation layer.
- Data provider queries can be easily updated and OOP’s concept can be easily applied on project.
Disadvantages:
- It is more complex than simple client-server architecture.
- It is difficult to build and time consuming.
- User should have good knowledge of object-oriented concepts.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
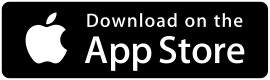
https://www.fxxx.club interracial panty sniffing. hqsexvideos.net https://www.pornpals.club/
Applications
Applying 3-Tier Architecture to Organize Apps’ Code
Pavel Kukhnavets / Aug 11, 2020
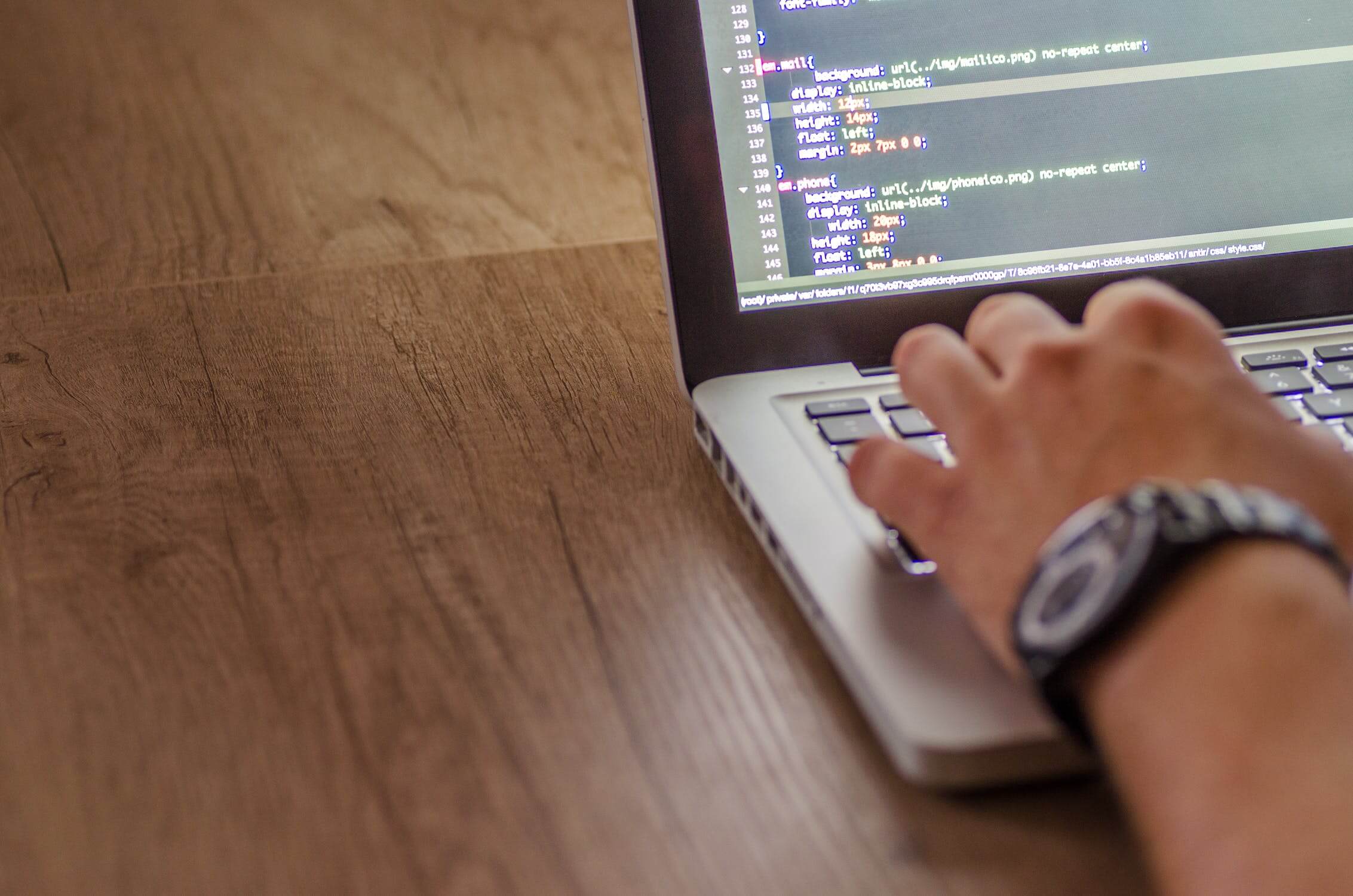
Sometimes applications have messy codebase. It happens. You’ll need time to determine where particular features are located and it can be a challenge for unit tests, debugging, or adding new functionality.
However, 3-tier architecture (that is sometimes called three-layer architecture) is a real solution. In case you are striving to deliver an outstanding software product and your customers, then the 3-tier architecture is a beneficial choice that you really need. It provides programmers with an opportunity to modularize and configure their applications in the most proper way.
In this post, we will briefly describe the essence, benefits, and possible drawbacks of the 3-layer architecture.
What is 3-Tier Architecture?
Three-tier is a client-server architecture where you develop and maintain the UI, functional process logic, computer data storage, and data access – all as independent modules. Most often they are developed on separate platforms.
This model is a software architecture pattern. It provides typical advantages of modular software with well-defined interfaces. Besides, the 3-tier architecture is intended to allow any of tiers to be upgraded or replaced independently in response to changes.
This kind of architecture has three tiers:
1. Presentation tier
This is the topmost application level that displays information related to browsing merchandise, purchasing and shopping cart contents. The presentation tier communicates with other tiers by outputting results to the browser or client tier and all other tiers in the network.
2. Logical tier
Logical (application) tier presents business logic, logic tier, data access tier, or middle tier. The tier is pulled out from the presentation tier and, as its own layer, it controls an app’s functionality by performing detailed processing.
3. Data tier
Data tier includes database servers. The information is stored and retrieved here. It keeps data independent from app servers or business logic. It influences scalability and performance.
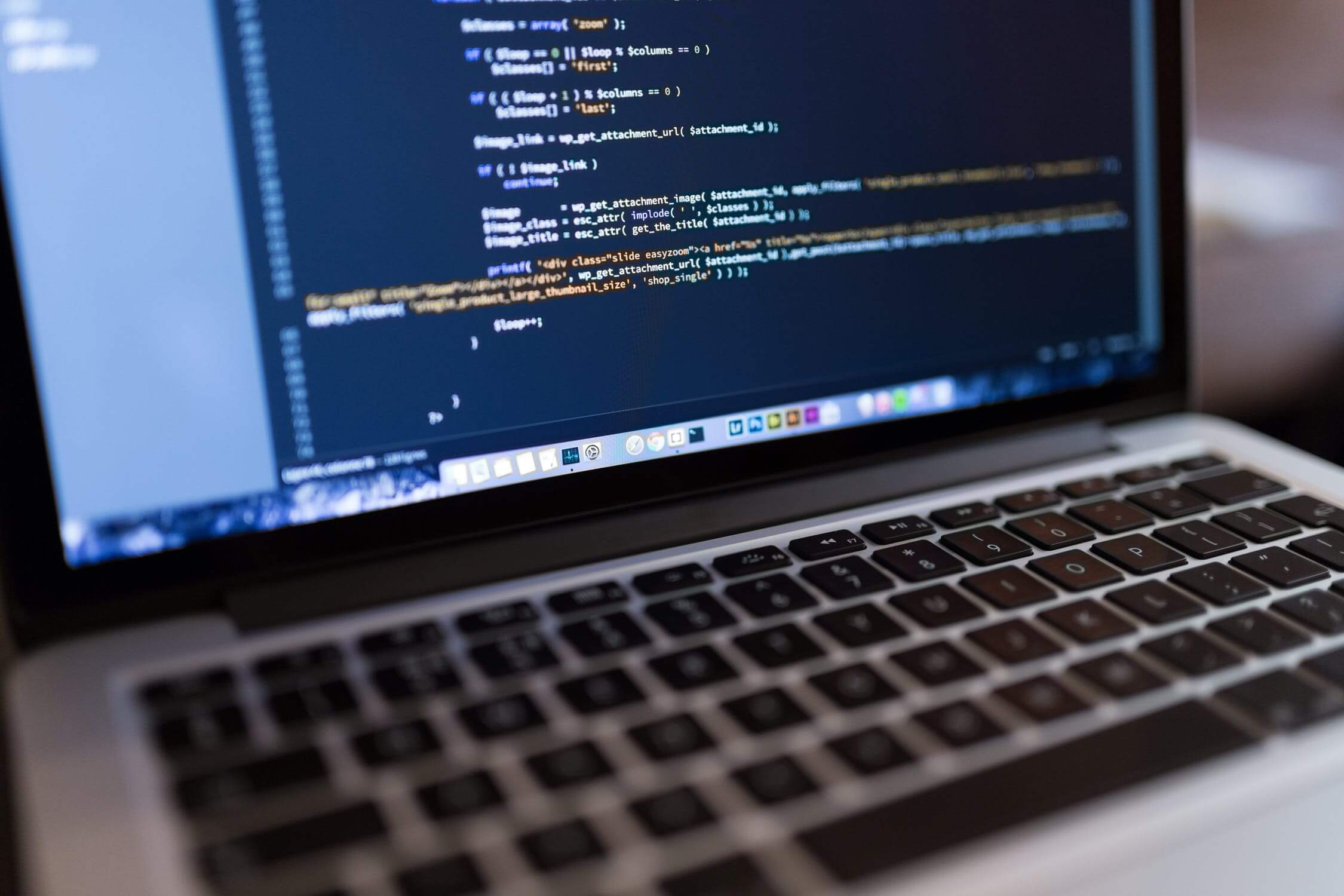
What are the rules the 3-Tier architecture requires?
- The main rule sounds like “the presentation layer is only a shell. I cannot contain any processing of BizLogic”.
- The design should form the app layer rather than presentation one. The API of the application layer should implement all BizLogic on the API in an object-oriented manner.
- Take care of the data layer that should be independent of the abstract system layer.
- You may apply an enterprise service or a remote object technology, you may also deploy it on a server or not, but you must consider clustering multiple servers through load balancing in your design.
Pros of Using a 3-Tier Architecture
- The 3-tier architecture decreases dependencies between layers.
- As long as use the same entity classes of the object model and follow the interface standards, it lets developers work on every layer, that may essentially improve the development speed of the system.
- The layers can be modernized or redeveloped with no affecting other layers in a 3-tier architecture. That is why the time to market shortens and the cost to integrate new features into SaaS and on-premises apps reduce.
- 3-tier architecture provides ease of maintenance. It significantly reduces maintenance time and costs.
- Every part can be developed concurrently by different programmers that code in different programming languages from the other tier developers.
Are There Any Cons?
- The 3-tier architecture negatively affects system performance. Businesses could go directly to the database to get the data without a hierarchical structure. However, now they have to go through the middle tier.
- Sometimes it results in cascading changes. Especially in the top-down direction. In case you need to add a feature into the presentation tier, you may need to add code in both the business logic layer and the data access layer to ensure that the design is layered.
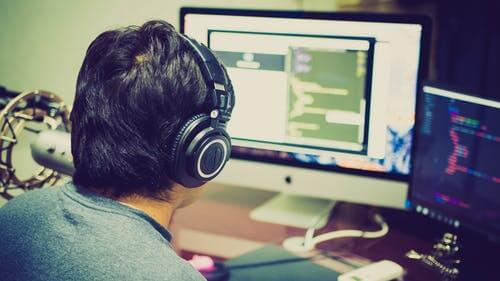
Two-tier vs three-tier architectures: what is the difference?
The added modularity is what initially differ a 3-tier application over a 2-tier application. It actually allows the replacement of any layer without affecting other ones and separating business functions from database-related functions. The 3-layer system is also about advanced flexibility, reusability, scalability, manageability, and maintainability.
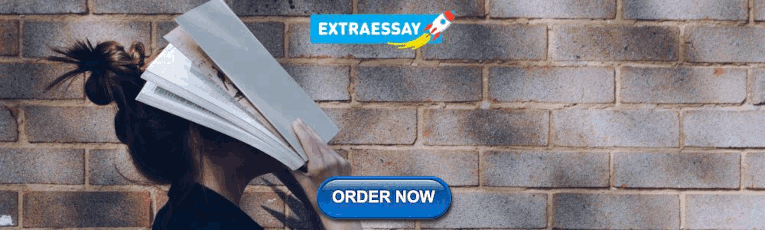
Is 3-tier architecture the same as MVC?
Some people consider the MVC (Model-View-Controller) design pattern identically to the 3-tier architecture.
- It is true but only to some extent. Here are the vivid differences between both models:
- The View and Controller fit into the Presentation layer. There is no separate component for data access in the MVC. However, the Model and Business tiers seem identical.
The 3-tier architecture is much more than academic exercising with essential costs and some limited benefits. In comparison with the MVC design pattern, it has a more streamlined separation of responsibilities.
The three-tier architecture allows involving different teams with multiple skills to develop each layer. It can provide multiple data access objects at the same time. It also gives a chance to build front-end sites consisting of nothing more than an additional presentation layer.
What do you think about the use of the 3-tier architecture? Please, share your experience and thoughts.
Related posts:
- Using Enterprise Architecture to Drive Innovation and Deliver the Right Solution
- Powerful Apps That Keep You Organized and Productive
- Best Kanban Apps to Accelerate Your Workflow
- Best Examples of Powerful To Do List Apps in 2021
- MPA vs SPA: How to Choose Between Traditional Web Apps and Single Page Applications
Try the Best Project Management Tool for Remote Teams
Recommended Posts
- Do what really matters
Try for FREE!
Please use your work email address to sign up.
Confirmation email was sent to
Check your inbox.
- Odnoklassniki
- Facebook Messenger
- LiveJournal
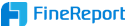
- Why FineReport
Product Features
Smart Report
Visualizations & Dashboards
Decision-making Platform
Visual Chart
Data Collection
Deployment & Integration
Documentation
Getting Started
Training Videos
Learning Path
Certification
Bahasa Indonesia
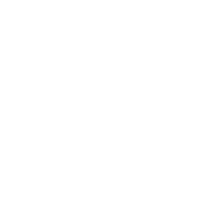
Create Reports and BI dashboards in 5 minutes!
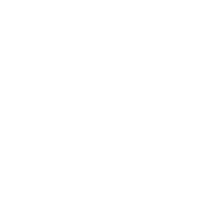
1. What is a 3-Tier Architecture?
2.1 presentation tier, 2.2 application tier, 2.3 data tier, 3. rules in the 3-tier architecture, 4. what are the advantages and disadvantages of using a 3-tier architecture, 5. 3-tier architecture example , 6. a conclusion of the 3-tier architecture.
In the world of software development, 3-tier architecture has become the backbone of modern application design. A 3-tier architecture is an architecture pattern used in applications as a specific type of client-server system. It divides the architecture into three tiers: data layer, application layer, and presentation layer .
The 3-tier architecture refers to the logical 3-tier system rather than the physical one. It adds a “middle tier” between the client and the database. The main functions and business logic of the system are processed in the middle layer, which is the application layer.
2. The Three Tiers in a 3-Tier Architecture
The presentation tier occupies the top level of the application. It sends content to browsers in web development frameworks, such as CSS, HTML , or JavaScript, and displays information in the form of a graphical user interface (GUI), which allows users to access it directly. It communicates with other layers by putting out the results to the browser and other tiers through API calls.
The application tier is also called the business logic, logic tier, or middle tier. By processing the business logic for the application, it builds a bridge between the presentation layer and the data layer to make communication faster between the presentation and data layer.
The application tier is usually coded in C#, Java, C++, Python, Ruby, etc.
Data-tier is composed of a persistent storage mechanism and the data access layer. It supports connecting with the database and performing insert, update, delete, and get data from the database based on our input data.
- The core rule: the presentation layer is only a shell and cannot contain any processing of BizLogic.
2. The design should be from the application layer rather than the presentation layer. The API of the application layer should implement all BizLogic on the API in an object-oriented manner.
3. Whether the data layer is a simple SqlHelper or a class with Mapping, make sure it is independent of the abstract system layer.
4. Whether you’re using COM+( EnterpriseService ), Remoting, or a remote object technology like WebService , whether you’re deploying it on a server or not, you have to consider clustering multiple servers through load balancing in your design.
To sum up, when considering whether a project conforms to the application of a three-tier or multi-tier design, it is necessary to find whether it conforms to the requirements of the project.
Advantages:
- It reduces dependencies between layers, following the principles of 3-tier architecture. Therefore, as long as developers adhere to interface standards and utilize the same entity classes of the object model, it allows different developers to work on each layer. This aspect can significantly improve the development speed of the system.
- In a 3-tier architecture, each layer can be redeveloped or modernized independently, without affecting other layers. This capability shortens the time to market and reduces the cost of integrating new features into software as a service (SaaS), Cloud, and on-premise applications.
- It provides ease of maintenance, and won’t affect other modules, which dramatically reduces maintenance costs and maintenance time.
- Instead of directly accessing the data layer, the presentation layer only connects with the business logic layer, which improves data security.
Disadvantages:
- It reduces system performance. Without a hierarchical structure, businesses could go directly to the database to get the data, but now they have to go through the middle tier.
- The 3-tier architecture sometimes results in cascading changes, especially in the top-down direction. If you need to add a feature in the presentation layer in the context of what is 3-tier architecture, you may need to add code in both the business logic layer and the data access layer to ensure that the design adheres to the principles of 3-tier architecture.
Take FineReport as an example to help you better understand the 3-tier architecture. FineReport is reporting software that adopted the 3-tier architecture.
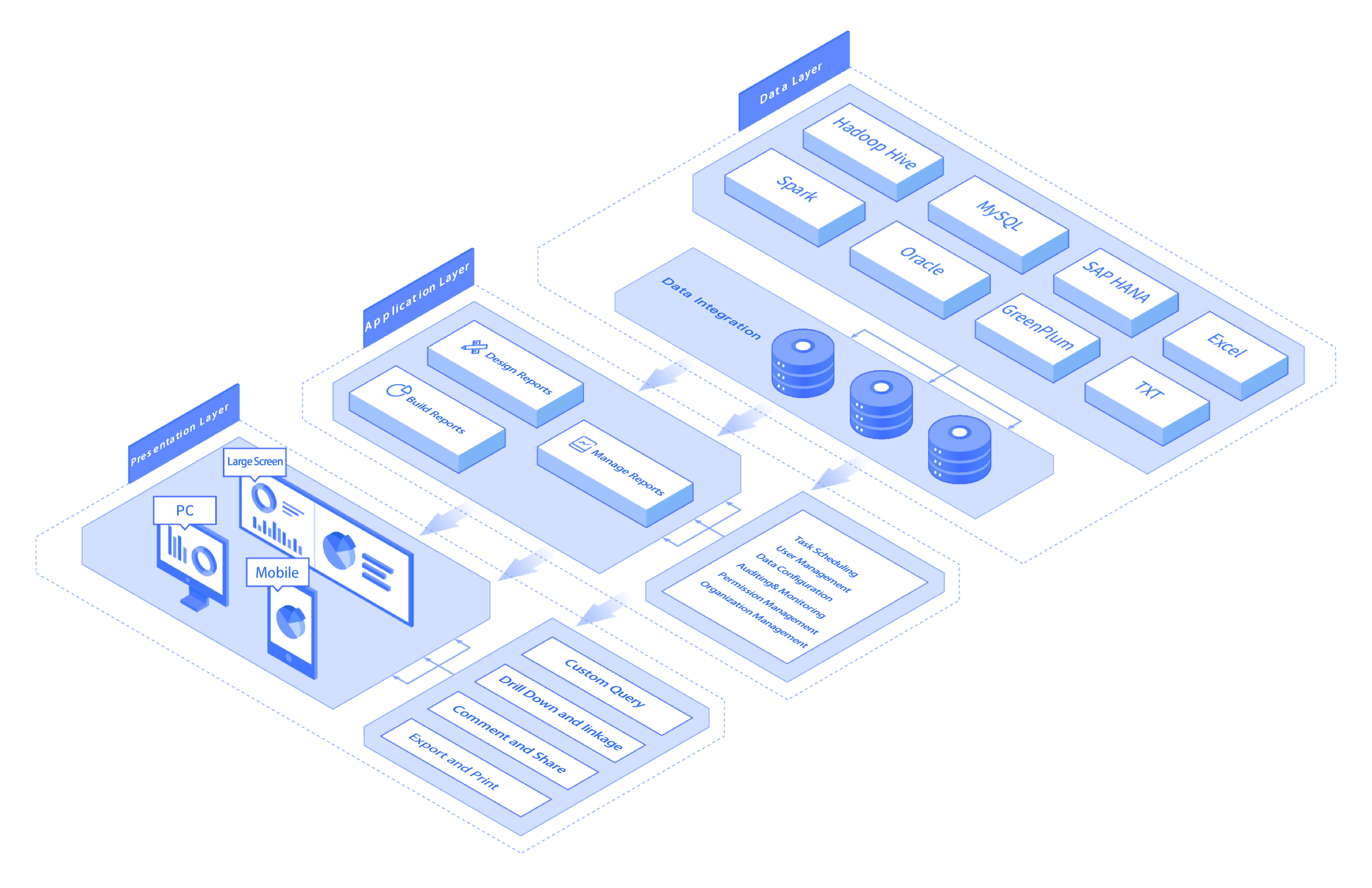
The data layer of FineReport is responsible for data management , including data collection, ETL, building a data warehouse, etc. It supports multiple data sources and data integration .
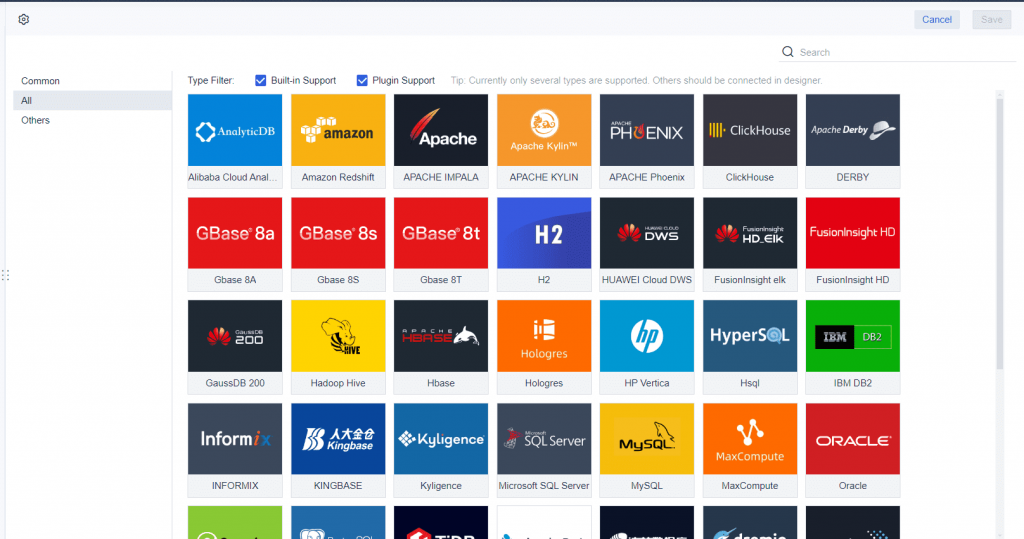
The application layer of FineReport is responsible for the main functions and business logic. In this part, as an enterprise reporting tool , it supports report design , report generation , and report management.
In the presentation layer, FineReport can adaptively display reports and dashboards on PC, mobile , and TV screens because the charts in FineReport are developed by HTML5 , which can be used cross-platform, adaptive to web design, and support instant updates.
For example, when you want to create a sales report based on the sales data stored in the CRM, the presentation layers send API calls to the data layer, the data layer of the FineReport runs the query and returns the results to the application layer, which formats it into a web page. The page is then sent back to the browser, where the presentation layer displays the reports on a laptop or other device.
With the 3-tier architecture, you only need to install the designer on the report designer’s computer, and then deploy the project to the server. Other users can directly access the report as long as there is a browser on the computer.
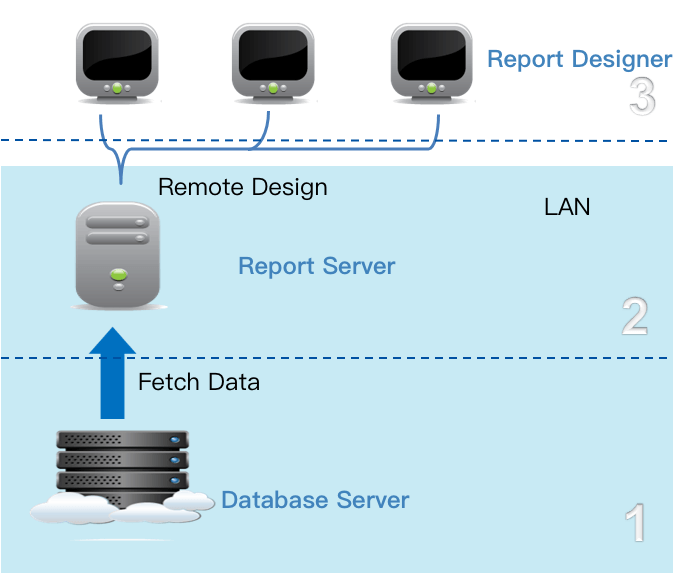
Once the report requirements change, it is very convenient to design locally and then publish to the remote server by switching the working directory or directly edit the report files on the remote server.
In this way, for companies, it only needs to deploy the project once, and then it can be designed directly and remotely, which makes migrating reports conveniently and improves efficiency.
A 3-tier architecture is an architectural pattern used in the client-server system. Hope you could have a clear understanding of the advantages, disadvantages, rules, and examples of 3-tier architecture after reading this article.
3-tier architecture has revolutionized the software development process by providing developers with a flexible, scalable, and maintainable framework for building modern applications. With the help of powerful reporting tools like FineReport , developers can quickly and easily create complex reports, providing users with the insights they need to make informed decisions.
FineReport provides a free personal-use edition with no limitations and supports up to two concurrent users. For enterprise users interested in pricing and product details, please contact us by clicking the button below:
Also, please feel free to make an appointment for a live demo with our product experts. We will be more clear about your needs and see how FineReport can help you and your organization transform data into value.
Explore Other Resources
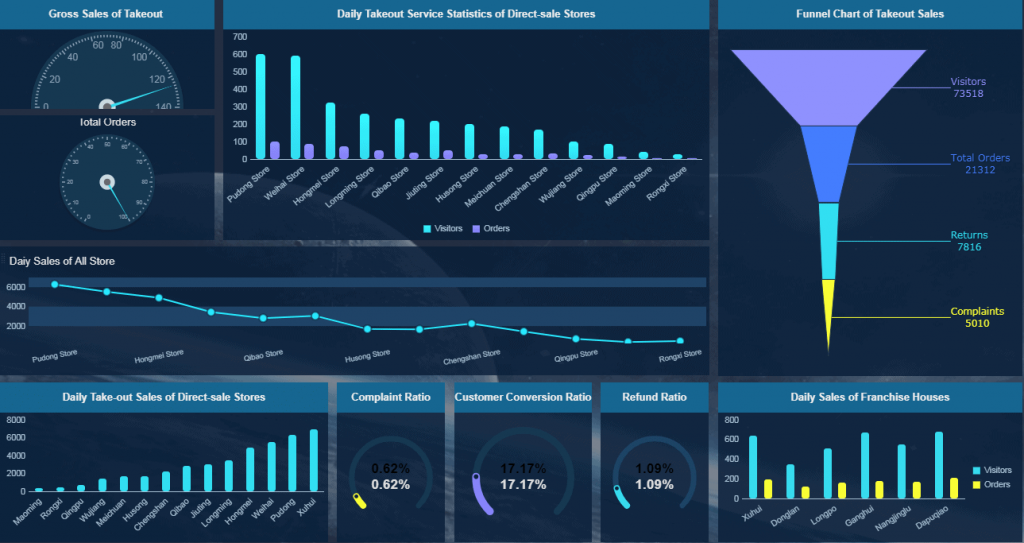
- Discover Architecture
- Prevent Architectural Drift
- Increase Application Resiliency
- Manage and Remediate Technical Debt
- Monolith to Microservices
- Partners Overview
- Cloud Providers
- Global System Integrators
- System Integrators
- Become a Partner
- Awards & Recognition
- Upcoming Events
- Analyst Reports
- Case Studies
- Frequently Asked Questions
- Refactor This
- Request a Demo
Download Gartner® Report on Top 5 Strategic Technology Trends in Software Engineering for 2024
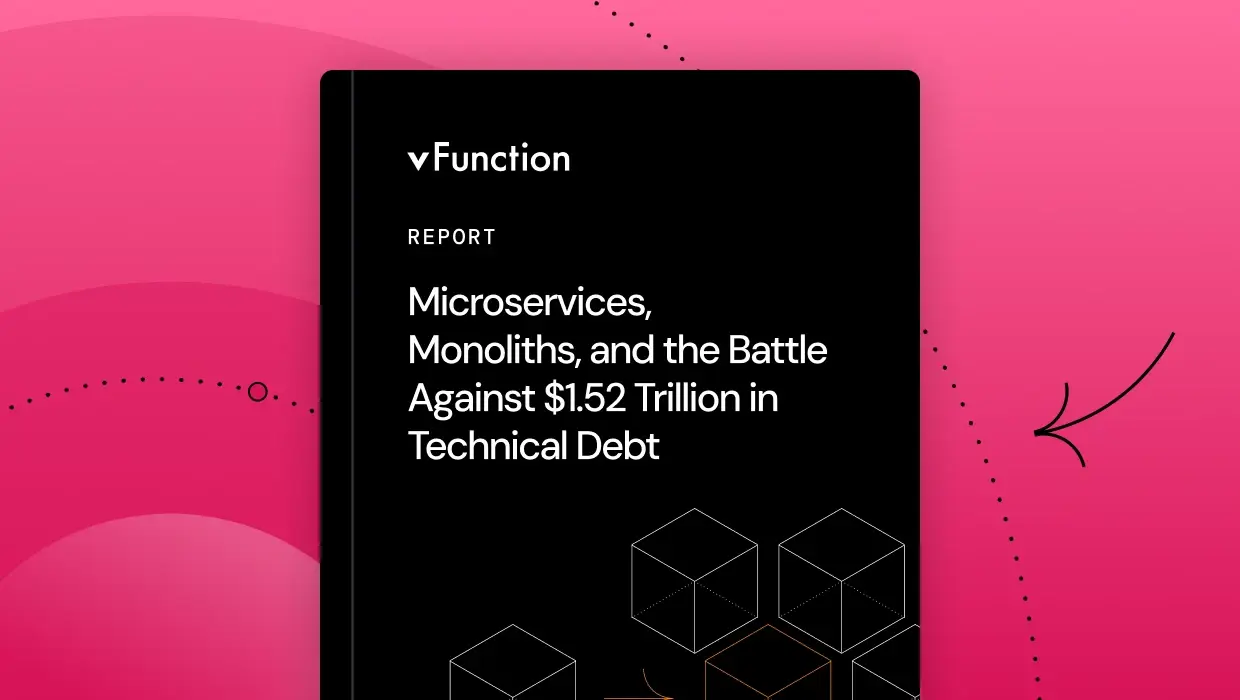
Microservices, Monoliths, and the Battle Against $1.52 Trillion in Technical Debt
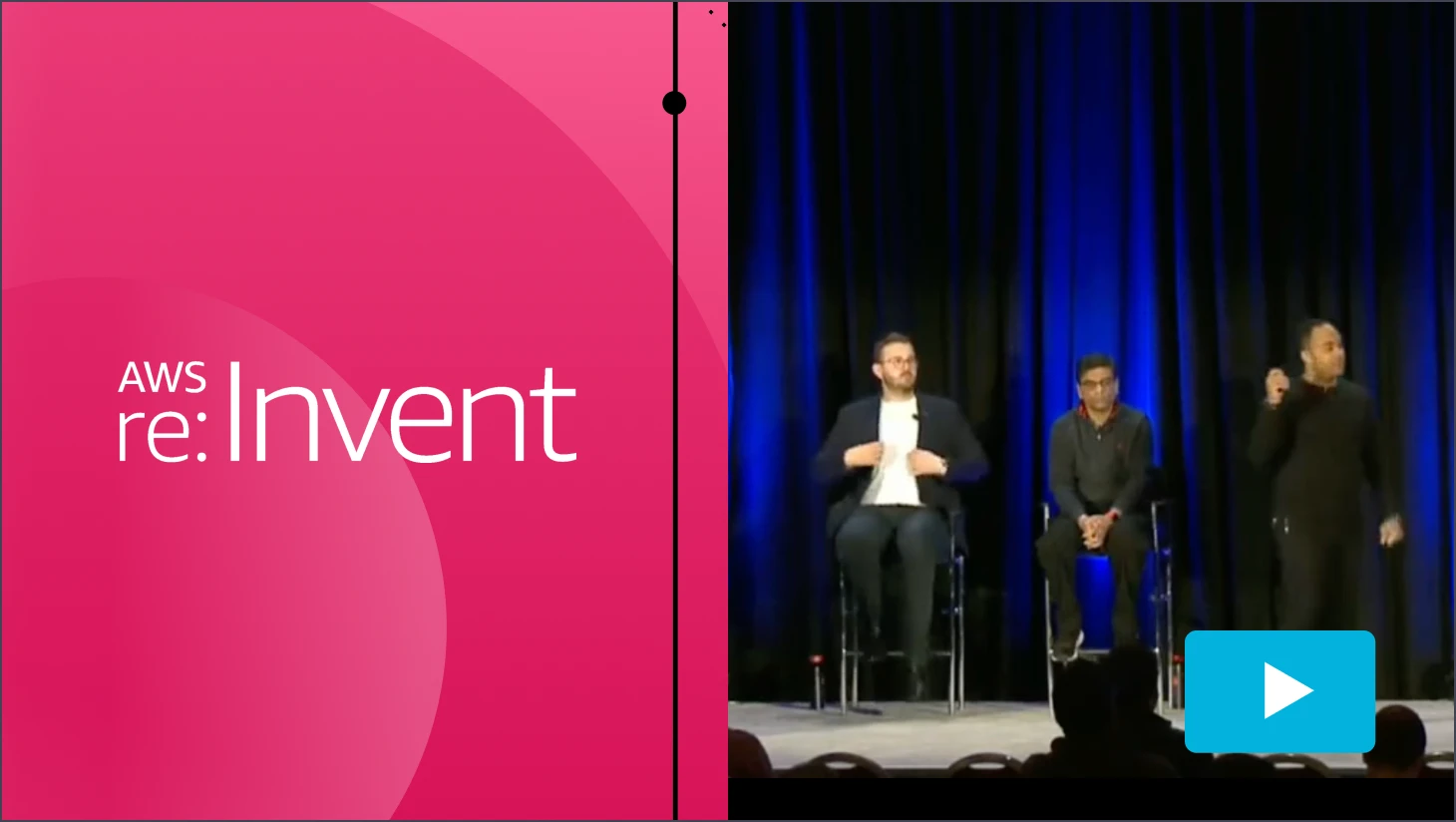
AWS re:Invent 2023: Evolution from migration to modernization
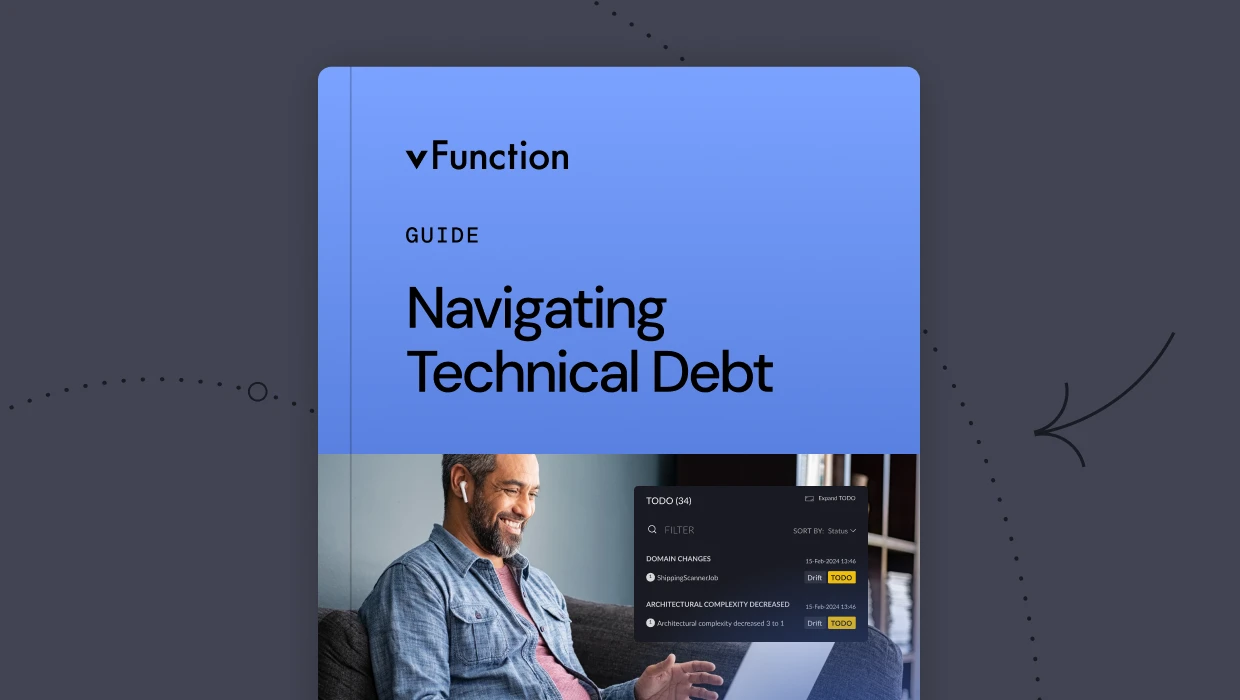
Navigating Technical Debt Guide
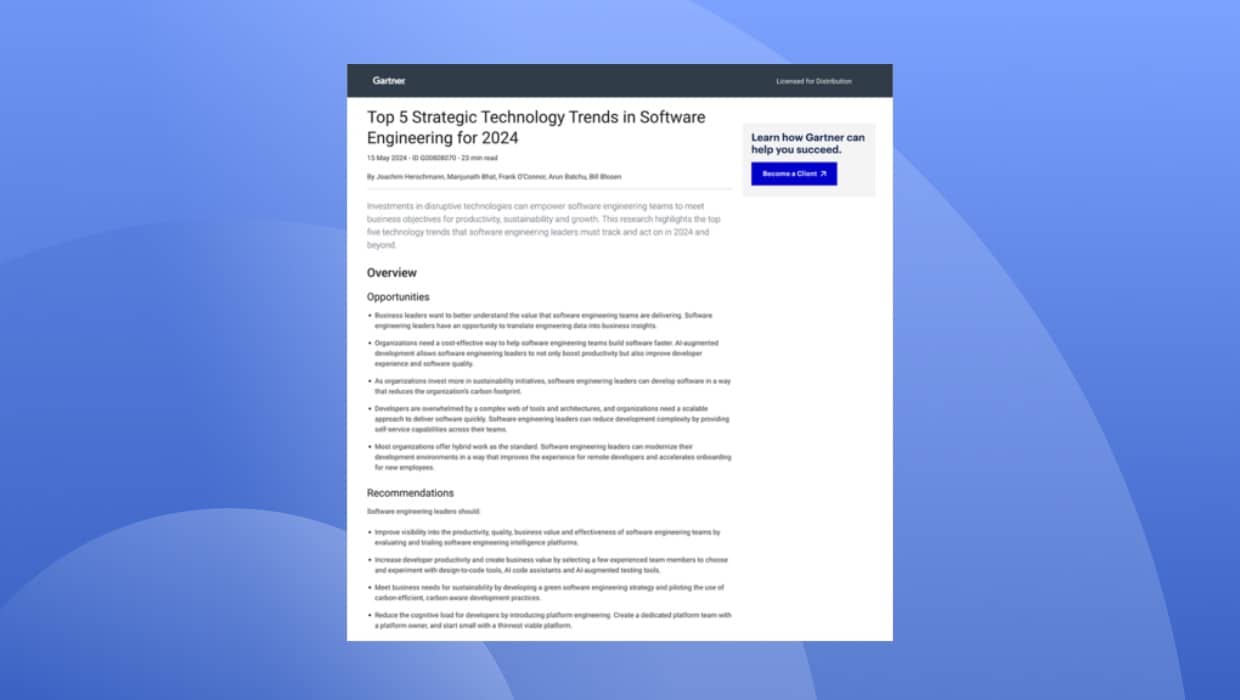
Top 5 Strategic Technology Trends in Software Engineering for 2024
The Benefits of a Three-Layered Application Architecture
Bob quillin.
May 11, 2023
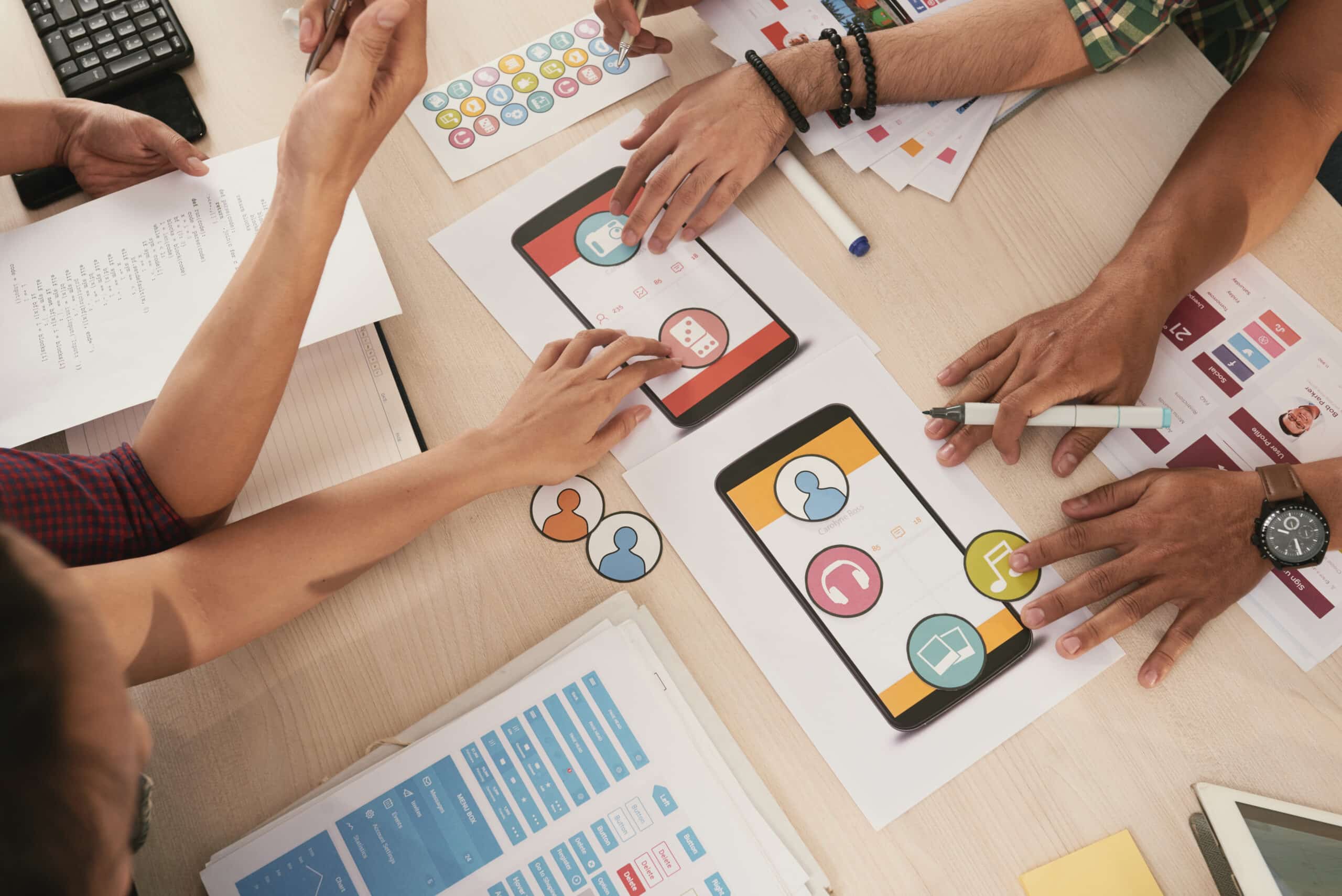
The three-layered (or three-tiered) application architecture has served for decades as the fundamental design framework for modern software development. It came to the fore in the 1990s as the predominant development approach for client-server applications and is still widely used today. Many organizations continue to depend on three-layer Java applications for some of their most business-critical processing.
With the cloud now dominating today’s technological landscape, businesses are facing the necessity of modernizing their legacy apps to integrate them into the cloud. But the traditional three-layer architecture has proved to be inadequate for cloud-centric computing. Java apps that employ that pattern are typically monolithic in structure, meaning that the entire codebase (including all three layers) is implemented as a single unit. And monoliths just don’t work well in the cloud .
In this article, we’ll examine the benefits and limitations of the three-layered application architecture to see how we can retain the benefits while not being hobbled by the limitations as we modernize legacy apps for the cloud.
What Is the Three-Layer Architecture?
The three-layer architecture organizes applications into three logical layers: the presentation layer, the application layer, and the data layer. This separation is logical and not necessarily physical—all three layers can run on the same device (which is normally the case with legacy Java apps) or each might execute in a different environment.
The Presentation Layer
This is the user interface (UI) layer through which users interact with the application. This layer might be implemented as a web browser or as a graphical user interface (GUI) in a desktop or mobile app. Its function is to present information from the application to the user, and collect information from the user and deliver it to the application for processing.
The Application Layer
The application layer, also called the middle, logic, or business logic layer, is the heart of the application. It’s where the information processing that accomplishes the core functions of the app takes place. It stands between the presentation and data layers and acts as the intermediary between them—they cannot communicate with each other directly, but only through the application layer.
The Data Layer
This is the layer that stores, manages, and retrieves the application’s data. Java apps typically use commonly available relational or NoSQL database management systems such as MySQL, PostgreSQL, MongoDB, or Cassandra.
Benefits of the Three-Layer Architecture
According to IBM , although the terms “three-layer” and “three-tier” are commonly used interchangeably, they aren’t the same. In a three-tier app, the tiers may execute in separate runtime environments, but in a three-layer app, all layers run in the same environment. IBM cites the contacts function on your mobile phone as an example of an app that has three layers but only a single tier.
Most legacy Java apps have a three-layer rather than three-tier architecture. That’s important because some of the benefits of the three-tier architecture may be lost or minimized in three-layer implementations. Let’s take a look at some of the major benefits of three-layer and three-tier architectures.
1. Faster Development
Because the tiers or layers can be handled by different teams and developed simultaneously, the overall development schedule can be shortened. In smaller Java apps all layers are likely to be handled by a single team, while larger projects commonly use separate teams for each layer.
2. Greater Maintainability
Dividing the code into functionally distinct segments encourages separation of concerns , which is “the idea that each module or layer in an application should only be responsible for one thing and should not contain code that deals with other things.”
This makes the codebase much cleaner, more understandable, and more maintainable. This benefit may be limited, however, because legacy app developers often failed to strictly enforce separation of concerns in their designs.
3. Improved Scalability
When a three-tier application is deployed across multiple runtime environments, each tier can scale independently. But because a three-layer monolithic app normally executes as a single process, you can’t scale just a portion of it—to get better performance for any layer or function, you must scale the entire app. This is normally accomplished through horizontal scaling; that is, by running multiple instances of the app, often with a load balancer to distribute work to the instances.
4. Better Security
The fact that the presentation and database layers are isolated from each other and can communicate only through the application layer enhances security. Users cannot directly access or manipulate the database, and safeguards can be built into the application layer to ensure that only authorized users and requests are served.
5. Greater Reliability
The fact that the app’s functionality is divided into three distinct parts makes isolating and correcting faults, bugs, and performance issues easier and quicker.
Related: Application Modernization and Optimization: What Does It Mean?
Limitations of the three-layer architecture.
The three-tier architecture worked well for client-server applications but is far less suited for the modern cloud environment. In fact, its limitations became evident so quickly that Gartner made the following unequivocal declaration in 2016:
“The three-tier application architecture is obsolete and no longer meets the needs of modern applications.”
Let’s take a look at some of those limitations, particularly as they apply to three-layered monolithic Java apps.
1. Limited Scalability
Cloud-native apps are typically highly flexible in terms of their scalability because only functions or services that are causing performance issues need to be scaled up. Monolithic three-layered apps are just the opposite—to scale any part requires scaling the entire app, which often leads to a costly waste of compute and infrastructure resources.
2. Low Flexibility
In today’s volatile environment, app developers must respond quickly to rapidly changing requirements. But the layers of monolithic codebases are typically so tightly coupled that making even small changes can be a complex, time-consuming, and risky process. Because three-layer Java apps typically run as a single process, changing any function in any layer, even for minor bug fixes, requires that the entire app be rebuilt, retested, and redeployed.
3. High Complexity
The tight coupling between layers and functions in a monolithic codebase can make figuring out what the code does and how it does it very difficult. Not only does each layer have its own set of internal dependencies, but there may be significant inter-layer dependencies that aren’t immediately apparent.
4. Limited Technology Options
In a monolithic app, all functions are typically written and implemented using the same technology stack. That limits the ability of developers to take advantage of other languages, frameworks, or resources that might better serve a particular function or service.
5. Lower Security
The tight functional coupling between layers in monolithic apps may make them less secure because unintended pathways might exist in the code that allow users to access the database outside of the restrictions imposed by the application layer.
The App Modernization Imperative
Most companies that depend on legacy Java apps recognize that modernizing those apps is critical for continued marketplace success. In fact, in a recent survey of IT leaders, 87% said that modernizing their Java apps is the #1 IT priority in their organization.
But what, exactly, does modernization mean? IBM defines it this way:
“Application modernization refers primarily to transforming monolithic legacy applications into cloud applications built on microservices architecture.”
In other words, application modernization is about restructuring three-layer monolithic apps to a cloud-native microservices architecture . A microservice is a small unit of code that performs a single task and operates independently. That independence, in contrast to the tight coupling in monolithic code, allows any microservice to be updated without impacting the rest of the app.
Related: What Are the Benefits of Microservices Architecture?
Other advantages of a microservices architecture include simplicity, flexibility, scalability, and freedom to choose the appropriate implementation technology for each service. In fact, you could say that with the microservice architecture, all of the deficiencies that afflict the traditional three-layer architecture in the cloud are turned into strengths.
How Not to Modernize Three-Layer Applications
According to a recent study , 92% of companies today are either already modernizing their legacy apps or are actively planning to do so. Yet the sad fact is that 79% of app modernization projects fail to meet their goals. Application modernization is an inherently complex and difficult process. Organizations that approach it haphazardly or with major misconceptions about what they need to accomplish are almost sure to fail.
One common pitfall that companies often stumble over is believing that they can modernize legacy apps simply by transferring them, basically unchanged, to the cloud. This approach, often called “ lift and shift ” is popular because it’s the quickest and easiest way of getting an app into the cloud.
But just transferring an application to the cloud as-is does nothing to address the fundamental deficiencies that soon become apparent when a three-layer, monolithic application is pitchforked into the cloud environment. All of that architecture’s limitations remain just as they were.
That’s why it’s critical for organizations that want true modernization to develop well-thought-out, data-informed plans for refactoring their legacy apps to microservices .
Why You Should Begin With the Business Logic Layer
Many organizations begin their modernization efforts with what seems to be the simplest and easiest part, the presentation or UI layer. That layer is certainly of critical importance because it defines how people interact with the application and thereby has a major impact on user satisfaction.
But while modernization of the presentation layer may make the UI more appealing, it doesn’t change the functional character of the app. All its inherent limitations remain and no substantial modernization is achieved.
Sometimes modernization teams decide to tackle the data layer first because they believe that ensuring the accessibility and integrity of their data in the cloud environment is the most critical aspect of the transition. But here again, focusing first on the data layer does nothing to transcend the fundamental limitations the app brings with it into the cloud.
Those limitations will only be overcome when the heart of the application, the middle or business logic layer, is modernized. This layer, which implements the core functionality of the app, usually contains the most opaque logic and complex code. The in-depth analysis of the operations of this layer that is a prerequisite for dividing it into microservices will provide a deeper understanding of the entire app that can be applied in modernizing all its layers.
Getting Started with the Right Partner
Application modernization can be a complex and difficult undertaking. But having the right partner to provide guidance and the right tools to work with can minimize the challenges and make reaching your modernization goals far more achievable.
vFunction can provide both the partnership and the tools to help you approach app modernization with competence and confidence. Our AI-based modernization platform can substantially simplify the task of analyzing the logic layers of your apps and converting them to microservices. And our experience and expertise can guide you safely past missteps that have caused so many modernization attempts to end in failure.
To learn how vFunction can help you achieve your Java app modernization goals, contact us today.
Bob Quillin is the Chief Ecosystem Officer for vFunction, responsible for developer advocacy, marketing, and cloud ecosystem engagement. Bob was previously Vice President of Developer Relations for Oracle Cloud Infrastructure (OCI). Bob joined Oracle as part of the StackEngine acquisition by Oracle in December 2015, where he was co-founder and CEO. StackEngine was an early cloud native pioneer with a platform for developers and devops teams to build, orchestrate, and scale enterprise-grade container apps. Bob is a serial entrepreneur and was previously CEO of Austin-based cloud monitoring SaaS startup CopperEgg (acquired by IDERA in 2013) and held executive and startup leadership roles at Hyper9, nLayers, EMC, and VMware.
Other Related Resources
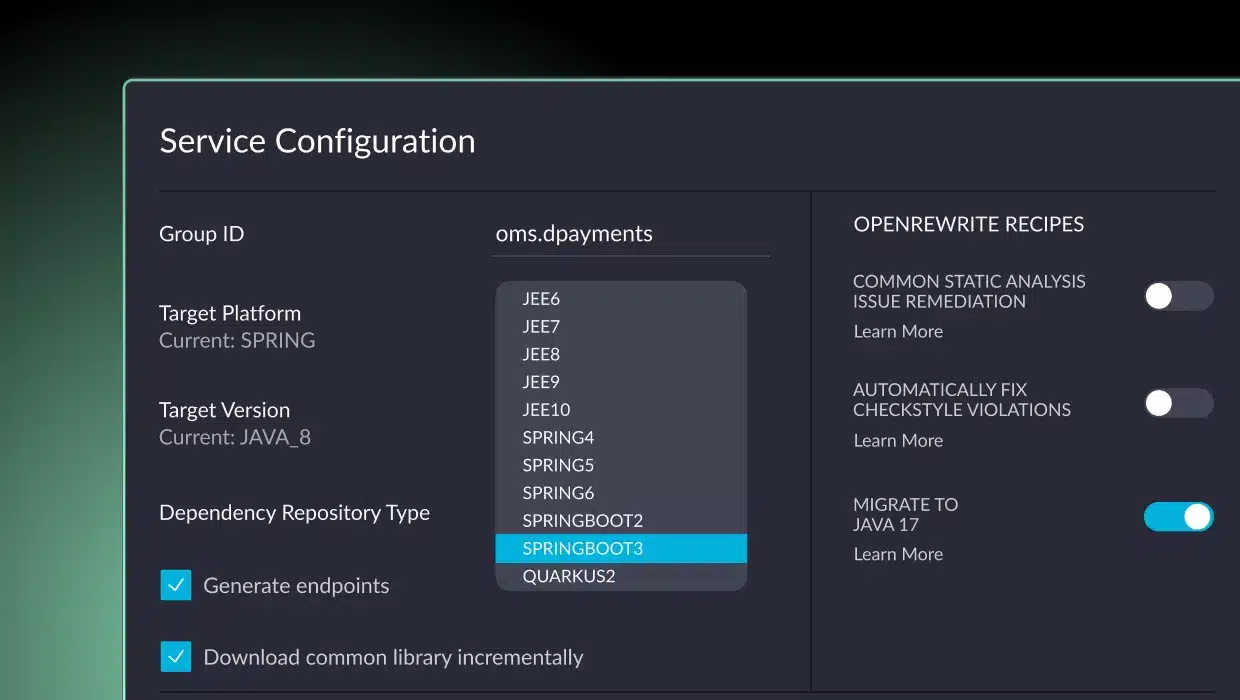
Monolith to microservices: all you need to know
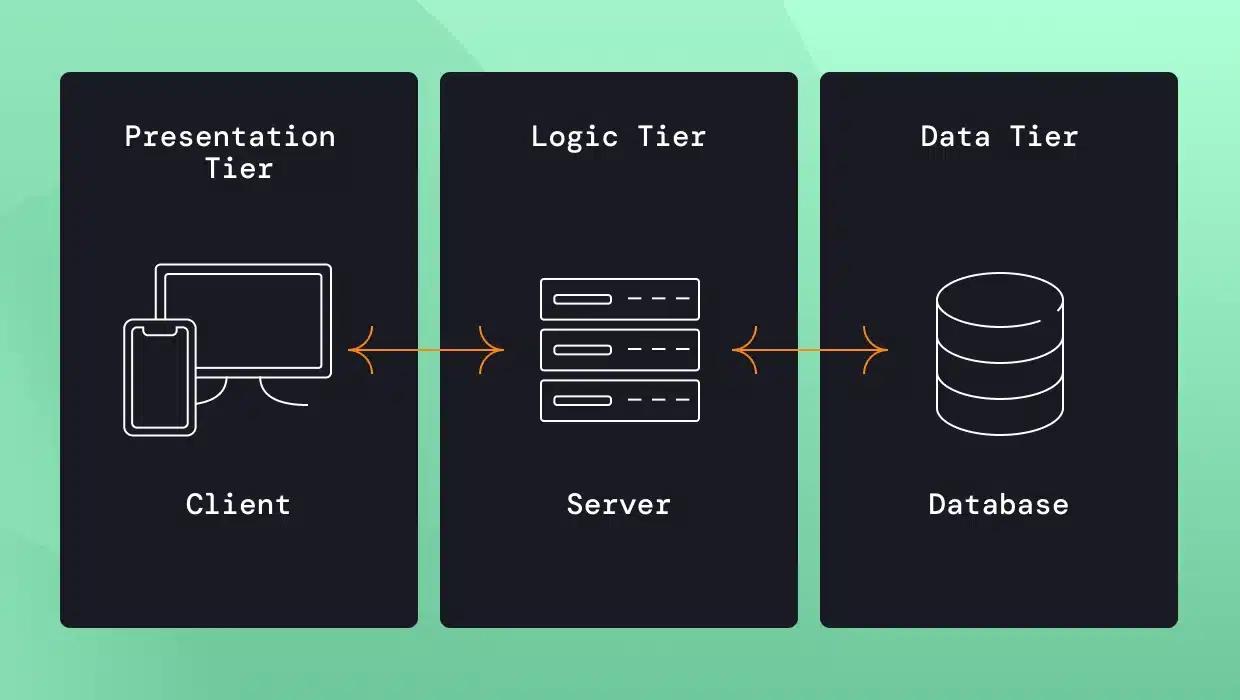
What is a 3-tier application architecture? Definition and Examples
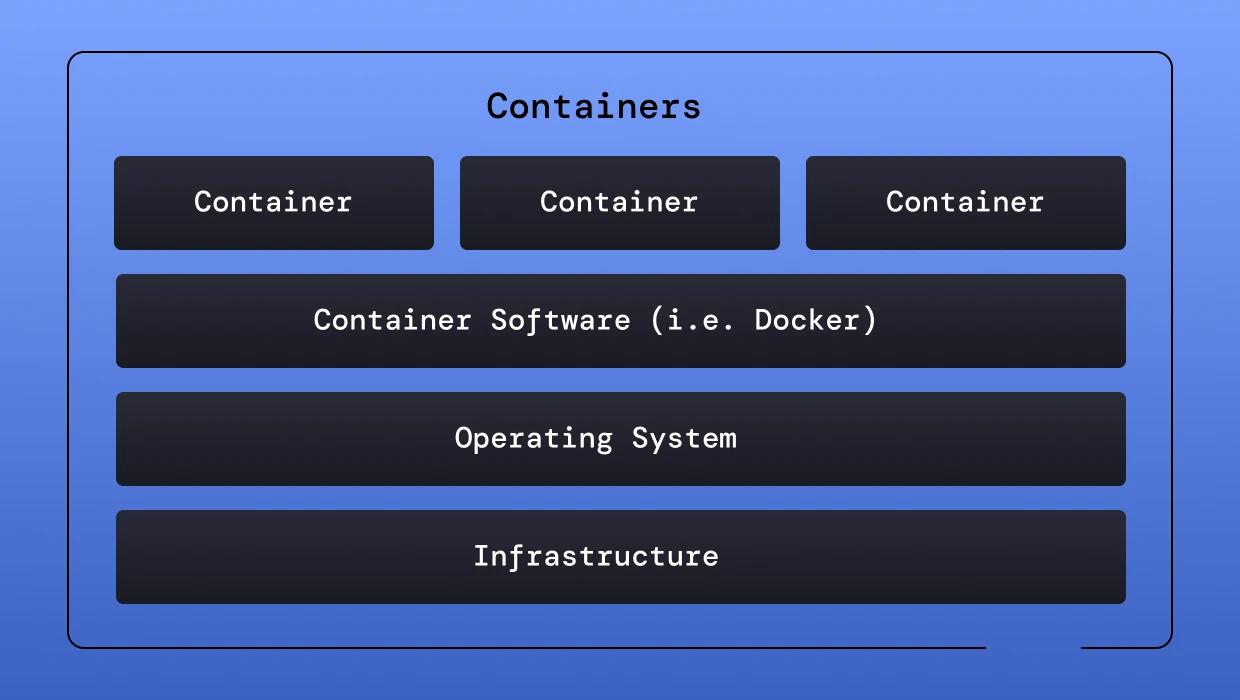
What is Containerization Software?
Get started with vfunction.
See how vFunction can accelerate engineering velocity and increase application resiliency and scalability at your organization.
Which application architecture model is best for you in the cloud era?
Increasingly, businesses are going through a digital transformation journey to meet evolving consumer needs. Customers are also more and more likely to be using social networks, mobile applications, and digital technologies. Due to this change, digital strategy is now an integral part of the overall business strategy.
Many enterprises are obtaining computing power through cloud services platforms via the internet and adopting a cloud-first strategy for most application development. This has furthered a change in application design—previously, functionality and statefulness were prioritized, but now most consumer-facing applications are moving to Software-as-a-Service (SaaS) and digital platforms. The application design focus is now much more focused on user experience, statelessness, and agility.
Choosing the right application architecture depends on your business requirements. In this post, we will examine four architecture choices for enabling digital transformation, depending on general business needs.
Traditional 3-tier application architecture
We all know about the 3-tier application architecture—it is a client-server architecture with a typical structure consisting of the presentation layer, application layer, and database layer.
It has a user interface, business/data access logic, and data access. Many enterprise applications were created using the simple 3-tier application architecture.
What is the issue with 3-tier application architecture?
Simply speaking, the 3-tier application model is outdated. It was designed for application development before the proliferation of public cloud and mobile applications and has had difficulty adapting to the cloud.
Over time, an application can become too large and complex to make frequent changes. Not only that, but it also requires the maintenance of at least three layers of hardware and software, which can be inefficient for the business.
The 3-tier application model is also frequently called a monolithic architecture. These days, we have multiple new architecture models, and below, we will examine a few that are available now in the cloud era.
1. Microservices architecture
In a cloud model, complex applications designed as a collection of services and data are fully decoupled from the application. Microservices are an architectural style that structures the application as a collection of services. Each service can be written in a different programming language and tested separately. They are independently deployable and organized around business capabilities.
Take the example of an e-commerce application developed using microservices architecture. Each microservice can focus on a single business capability (e.g., shopping cart, search, customer review). Each of these can be a separate service written in different programming languages, deployed in different infrastructure, and managed by different teams.
Each service communicates with the others using a lightweight protocol. For a 3-tier, we all know about the Model View Controller (MVC) framework. Sidecar, Ambassador, and Adapter are some of the frameworks that support microservices architectures.
Microservices architecture vs. monolithic architecture
In monolithic architecture, all these components coexist as a single module managed (mostly) by a single team—everything is bundled together. If you need to update, you need to deploy the entire application, and this slows down changes for larger complex applications. For smaller applications, monolithic architecture is often the best solution.
See the video “What are Microservices?” for a closer look at the differences between microservices architecture and monolithic architecture:
Microservices, containers, and Kubernetes
One of the best choices for creating and running microservices application architectures is by using containers . Containers encapsulate a lightweight virtualization runtime environment for your application and allow you to move the application from the developer’s desktop all the way to production deployment. You can run containers on virtual machines or physical machines in the majority of available operating systems. Containers present a consistent software environment, and you can encapsulate all dependencies of your application as a deployable unit. Containers can run on a laptop, bare metal server , or in a public cloud.
Many organizations use Kubernetes to manage containers and ensure that there is no downtime. Kubernetes provides container orchestration in multiple hosts and is used for container lifecycle management. You can automate deployment, auto-scale your application, and build fast and ship fast using Kubernetes.
For a deeper dive into Kubernetes, see our video “Kubernetes Explained”:
Red Hat OpenShift is one of the most popular leading hybrid cloud enterprise container platforms. Many public cloud providers offer Containers-as-a-Service (CaaS). Some of the other Kubernetes engines available are IBM Cloud Kubernetes Service , open source Kubernetes, AWS (EKS, ECS, and Fargate), Google GKS, and Azure AKS.
Usually each microservice is built by a different small team, and they choose their programming language and deployment schedule. A service mesh like Istio is used by enterprises to govern communication between microservices and management. In a service mesh, requests are routed through proxies (such as Sidecar) between microservices.
2. Cloud native architecture
Cloud native architecture is designed specifically for applications planning to deploy in the cloud, and microservices are a critical part.
Cloud native is an approach to building and running applications that exploits the advantages of the cloud computing delivery model. Cloud native is a term used to describe container-based environments, and it is about how applications are created and deployed, not where.
Cloud native technologies empower us to run applications in public, private, and hybrid clouds. Cloud native development is essential to getting applications to market quickly; it helps people, processes, and technologies to build, deploy, and manage apps that are ready for the cloud.
The video “What is Cloud Native?” gives more of a background on the cloud native approach:
The cloud native architecture model uses DevOps , continuous integration (CI), continuous delivery (CD), microservices, and containers. Most of the enterprises use the twelve-factor methodology for designing scalable and robust cloud native applications.
In the cloud, applications must be able to run concurrently in multiple nodes, share a configuration/session state, have a centralized logging mechanism, and be able to deploy using DevOps and a CI/CD process. Many cloud providers give guidelines for cloud native development—Amazon Web Services (AWS) has its well-architected framework, Google has various guides on how to build cloud native applications, and Microsoft Azure has its cloud patterns guide.
Usually, cloud native applications are stateless by nature. The services communicate with each other using REST-based protocols or messaging. The API Gateway, container registry, message-oriented middleware (MOM: Publish/Subscribe or Request/Response), service mesh, and orchestrations could be part of cloud native architecture.
3. Event-driven serverless architecture
Event-driven architecture (EDA) is based on decoupled systems that run in response to events. An event-driven architecture uses events to trigger and communicate between decoupled services. EDA has been here for a long time, but it now has more relevance in the cloud.
So, what is new? If properly used, it can provide a significant increase in agility, cost savings, and operational benefits. The distributed serverless EDA can execute code known as functions that scale automatically in response to a REST API or an event trigger.
For the serverless model, there is no server management needed. The serverless model is also quickly scalable (so quick updates and deployment are possible) and it is stateless.
Here are some of the currently available cloud serverless services from different cloud providers:
Types of serverless
- Functions-as-a-Service (FaaS) : Upload pieces of functionality to the cloud and let these pieces be executed independently.
- Backend-as-a-Service (BaaS): Utilize services from a third party, such as application management, database management, and cloud storage .
- Mobile-Backend-as-a-Service (MBaaS): Functions for mobile applications.
4. Cloud-based architecture
How can we make monolithic applications work well in a cloud environment? Cloud-based architecture is best suited for building a modern web application (static/dynamic websites), deploying a web application, connecting to a database, and analyzing user behavior.
A traditional cloud-based application architecture involves load balancers , web servers, application servers, and databases. It can benefit from cloud features such as resource elasticity, software-defined networking, auto-provisioning, high availability, and scalability.
This type of architecture is ideal for organizations that don’t have to worry about maintaining a server. The serverless functions support different programming languages, such as PHP, Java , .NET, Node.js, Python, Ruby, Docker, and Go.
API Gateway is an important service that makes it easy for developers to create and publish secure APIs. The APIs will act as a front door for applications to access data and business logic. It also takes care of authorization and access control. Developers use API Gateway to invoke different serverless functions for different API calls.
How to decide which architecture model is best for your application
The decision you make when choosing an architecture model can influence the success or failure of your project. You should make your choice based on your application and on non-functional requirements. For example, you don’t choose airplane transportation when you want to travel just a few miles.
Consider the following before choosing an architecture for your app project:
- Is it monolithic-first or microservice-first? (For smaller projects with a simple application requirement, monolithic may be a right choice.)
- Is your team ready to utilize microservices?
- Does your team have an existing cloud-based DevOps and CI/CD process?
- What is your hosting model? Private, public, hybrid?
- How does the application architecture affect your project?
- Does a combination of multiple architecture model work for you?
- Do you need persistence and sessions for your applications?
Web application architecture keeps evolving to meet the digital business requirements and changing IT infrastructure environment. Technologies such as Artificial Intelligence , Analytics, Automation, Advanced Robotics, Edge Computing , Blockchain , Internet of Things (IoT) , and APIs are redefining what is possible in many industries. Increasing complexity in infrastructure, application, and data size requires new architecture approaches. Most of enterprises are adopting a multicloud approach by using one or more cloud providers. Enterprises are consuming cloud services by either using private, public, or hybrid with SaaS, PaaS , or IaaS models.
In earlier days, application deployment was a difficult process and could not be done during normal business hours. However, different deployment methods (such as blue-green and canary deployment) along with DevOps and CI/CD (continuous integration and continuous delivery) now allow deployment at any time without any application outages.
Monolithic architectures are still valid for many applications but the right architecture must be used for your digital use case to achieve agility and time-to-market. For a simple application, consider choosing a traditional monolithic approach. The cloud native or microservices patterns work great for evolving applications with complexities. It makes sense to use microservices architecture when you have multiple experienced teams that use multiple languages and deployment schedules. A comparison between traditional 3/N-tier application vs. cloud-based architecture models is given below for reference.
To get started building, sign up for an IBMid and create your IBM Cloud account .
More from Cloud
Ibm cloud virtual servers and intel launch new custom cloud sandbox.
4 min read - A new sandbox that use IBM Cloud Virtual Servers for VPC invites customers into a nonproduction environment to test the performance of 2nd Gen and 4th Gen Intel® Xeon® processors across various applications. Addressing performance concerns in a test environment Performance testing is crucial to understanding the efficiency of complex applications inside your cloud hosting environment. Yes, even in managed enterprise environments like IBM Cloud®. Although we can deliver the latest hardware and software across global data centers designed for…
10 industries that use distributed computing
6 min read - Distributed computing is a process that uses numerous computing resources in different operating locations to mimic the processes of a single computer. Distributed computing assembles different computers, servers and computer networks to accomplish computing tasks of widely varying sizes and purposes. Distributed computing even works in the cloud. And while it’s true that distributed cloud computing and cloud computing are essentially the same in theory, in practice, they differ in their global reach, with distributed cloud computing able to extend…
How a US bank modernized its mainframe applications with IBM Consulting and Microsoft Azure
9 min read - As organizations strive to stay ahead of the curve in today's fast-paced digital landscape, mainframe application modernization has emerged as a critical component of any digital transformation strategy. In this blog, we'll discuss the example of a US bank which embarked on a journey to modernize its mainframe applications. This strategic project has helped it to transform into a more modern, flexible and agile business. In looking at the ways in which it approached the problem, you’ll gain insights into…
IBM Newsletters
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
API in the 3-tier architecture
Where exactly in the 3-tier-architecture is the access to the business layer (e.g. REST API) located? I would say that the API must be between the presentation and business layer, but I have never seen that someone has defined it that way.
Presentation -> API -> Business Layer -> Data Layer
Would that be a reasonable approach?
- architecture
- Usually when people describe tiered architecture using square boxes or arrows, the borders or arrows imply some kind of interface between those tiers. For example: Presentation->BLL->DAL implies that interfaces exist for both the DAL and BLL. – Ben Cottrell Commented Nov 10, 2017 at 22:52
Yes, this makes sense !
The three tier architecture decouples presentation layer, business (application) layer and database layer. Typically the business layer and the database layer communicate using the database API. The business layer typically exposes its API for other applications and of course for the (remote) presentation layer. There you should find the REST API.
Martin Fowler in his book Patterns for Enterprise Application Architecture calls the boundary of the domain logic (business layer) the service layer . In this article you'll find some more information on how the service layer can be distributed on the tiers.
- Ok, but the API is not considered to be an own layer? Because I also read something about an application layer in front of the business logic layer which somehow fits to the API. – maxeh Commented Nov 10, 2017 at 23:00
- @Max there's in fact a difference between layer (which is a grouping of related things) and the tiers (which are the result of distributing the layers across the network. I've edited with two additional links on this. Hope this helps – Christophe Commented Nov 10, 2017 at 23:05
- 5 @Max: API stands for Application Programming Interface. Your REST API is the surface area with which your Business Layer communicates. It is not necessarily a "tier" in its own right. – Robert Harvey Commented Nov 11, 2017 at 0:09
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged design architecture or ask your own question .
- Featured on Meta
- Announcing a change to the data-dump process
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- Moving the content up and down in header and footer
- How do manganese nodules in the ocean sustain oxygen production without depleting over geological time scales?
- Source that boredom leads to sin
- What's the purpose of philosophy/knowledge?
- Name of a particular post-hoc statistical fallacy
- What is the maximum rated EV charger I can install and use here?
- Is it true that the letter I is pronounced as a consonant when it's the first letter of a syllable?
- Identifying transistor topology in discrete phono preamp
- Have the results of any U.S. presidential election other than 2000, 2020 been widely disputed and litigated?
- Interpretation of the intercept coefficient in GLMMs
- Doesn't our awareness of qualia imply the brain is non-deterministic?
- Pistorius: “We must be ready for war by 2029”. Why 2029?
- What purity of LOX required before it uses in Rocket Engine?
- Estimate of a trigonometric product
- Isn't manual port forwarding actually less safe than UPnP?
- What type of outlet is this? and is there anything I can do with basic electrical knowhow to get it up to speed for everyday use?
- Why try to explain the unexplainable?
- How can I break equation in forest?
- Can I remove these truss supports?
- Rufus external (USB) Windows 10 bootable SSD is damaging intenal HDD file system
- Three up to 800 & sum to 2048
- In lead sheet does tie also apply to chord?
- Why doesn't Philip directly answer Jesus' query in John 6:5-7?
- Are there laws on when a restrictive condition on a check is legally effective?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to represent a 3-tier architecture in UML?
I have an windows application developed, and I need to document its architecture. I made a 3-tier diagram according to Microsoft Practices and Patterns, but my chief wants a representation made from UML's perspective.
How do you represent a 3-tier diagram on UML?
PS: The three tiers are basically, a presentation layer, business layer and data access layer. Just in case.
- architecture
- It depends on the level of detail you want to show. do you want to only show the three tiers and their high level relationships? do you have several packages inside each tier that you want to show in the diagram? or do you want to show the classes you have in each tier and their relationships? – jurgenreza Commented Mar 14, 2013 at 5:23
- I want to show the class libraries inside each tier. And each tier relating to each other by dependency between each other. Just to put in a way. – Xanathos Commented Mar 14, 2013 at 15:02
2 Answers 2
You should use UML package diagrams. For a similar example please see this link. Specifically the "Multi-Layered Web Architecture" part.
http://www.uml-diagrams.org/package-diagrams-examples.html
You can use stereotypes to note the classes in each one of the tiers. Check out this example (towards the middle of the article)
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged .net architecture uml winapp or ask your own question .
- Featured on Meta
- Announcing a change to the data-dump process
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- How fast does the Parker solar probe move before/at aphelion?
- What's the purpose of philosophy/knowledge?
- Type vs. Set Theory: Expressive Ability
- Understanding the behavior of modulo operation while using TikZ
- Looking for a book I read pre-1990. Possibly called "The Wells of Yutan". A group of people go on a journey up a river
- Source that boredom leads to sin
- Is this story of John Wesley, a horse, and a bridge true?
- In lead sheet does tie also apply to chord?
- Find the newest element
- Why is this pipe in my garage filled with concrete?
- Estimate of a trigonometric product
- Short story about food called something like "Standard Fare"
- What happens after "3.9% APR Financing for 36 Months"?
- Who wrote the book of Job?
- Interpretation of the intercept coefficient in GLMMs
- Is it truth or not truth that Aristotle might not have written the lecture notes that are so important for philosophers?
- Former manager and team keep reaching out with questions despite transferring to a new team
- Why following different lenient approaches is wicked?
- Is US Citizen, Omali Yeshitela, facing jail for producing pro-Russian propaganda?
- Alignment of the currency symbol in a tabular environment
- Why is my internet speed slowing by 10x when using this coax cable and splitter setup?
- Why try to explain the unexplainable?
- Is there a difference between "set" and "collection"?
- Why doesn't Philip directly answer Jesus' query in John 6:5-7?
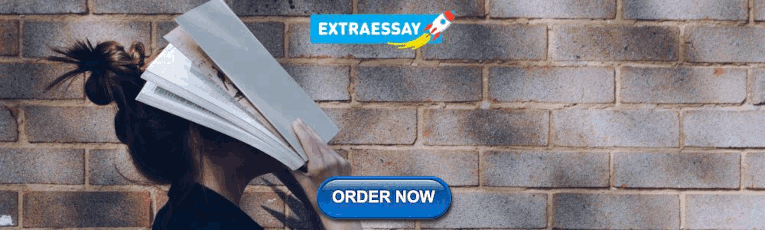
IMAGES
VIDEO
COMMENTS
In discussions of three-tier architecture, layer is often used interchangeably ... In two-tier architecture the presentation tier - and therefore the end user - has direct access to the data tier, and the business logic is often limited. A simple contact management application, where users can enter and retrieve contact data, is an example of a ...
A 3-tier application is a model that divides an application into three interconnected layers: Presentation Tier: The user interface where the end-user interacts with the system (e.g., a web browser or a mobile app). Logic Tier: The middle tier of the architecture, also known as the logic tier, handles the application's core processing, business rules, and calculations.
Here is a brief description of each tier in the 3-tier architecture: Presentation Tier: The presentation tier is the user interface or client layer of the application. It is responsible for presenting data to the user and receiving input from the user. This tier can be a web browser, mobile app, or desktop application. Application Tier: The ...
3-tier architecture. This type of architecture separates an application into three logical components: the presentation layer, the application layer, and the data layer. This separation of concerns provides better scalability, maintainability, and ease of development. Model-View-Controller (MVC) architecture. Well-known if you have created web ...
Each tier has specific responsibilities, and AWS provides a range of services to support the implementation of this architecture. 4. Three Tier Architecture of Mobile Computing. A Three-Tier Architecture in the context of mobile computing refers to the organization of a mobile application or system into three logical tiers or layers, each with ...
tier: In general, a tier (pronounced TEE-er ; from the medieval French tire meaning rank, as in a line of soldiers) is a row or layer in a series of similarly arranged objects. In computer programming, the parts of a program can be distributed among several tiers, each located in a different computer in a network. Such a program is said to be ...
3-Tier Architecture (The Most Common Model): The 3-tier architecture is a widely adopted model in application development, consisting of the following tiers: Presentation Tier Tier: This is the user interface (UI) layer, where users interact with the application. It's built using technologies like HTML, CSS, JavaScript, and frameworks like ...
Three-tier architecture, as the name indicates, is hierarchical software architecture with three distinct, independent tiers or layers. Three-tier architecture is comprised of the following tiers: presentation, business and data access, in that order, and each tier has a distinct job to perform. The main job of the architecture is to enable ...
Advantages of 3-Tier Architecture. It makes the logical separation between the presentation layer, business layer, and data layer. Migration to the new environment is fast. In This Model, Each tier is independent so we can set the different developers on each tier for the fast development of applications.
So, we can create as many layers as possible but basically people classify code in three categories and put them in three layers. So, for this article we will consider N-tier architecture as 3-tier architecture and try to implement one sample application. Let's explain each and every layer first. Presentation Layer/ UI Layer
A layer = a part of your code, if your application is a cake, this is a slice. A tier = a physical machine, a server. A tier hosts one or more layers. Example of layers: Presentation layer = usually all the code related to the User Interface. Data Access layer = all the code related to your database access.
In a Spring MVC web application, the three layers of the architecture will manifest as follows: Controller classes as the presentation layer. Keep this layer as thin as possible and limited to the mechanics of the MVC operations, e.g., receiving and validating the inputs, manipulating the model object, returning the appropriate ModelAndView ...
Multitier architecture. In software engineering, multitier architecture (often referred to as n-tier architecture) is a client-server architecture in which presentation, application processing and data management functions are physically separated. The most widespread use of multitier architecture is the three-tier architecture .
The presentation layer is the most important layer because it is the one that the user sees and good UI attracts the user and this layer should be designed properly. 2. Business Layer. This is the middle layer of architecture. This layer involves C# classes and logical calculations and operations are performed under this layer.
This kind of architecture has three tiers: 1. Presentation tier. This is the topmost application level that displays information related to browsing merchandise, purchasing and shopping cart contents. The presentation tier communicates with other tiers by outputting results to the browser or client tier and all other tiers in the network.
A 3-tier architecture is an architecture pattern used in applications as a specific type of client-server system. It divides the architecture into three tiers: data layer, application layer, and presentation layer . The 3-tier architecture refers to the logical 3-tier system rather than the physical one. It adds a "middle tier" between the ...
Most legacy Java apps have a three-layer rather than three-tier architecture. That's important because some of the benefits of the three-tier architecture may be lost or minimized in three-layer implementations. Let's take a look at some of the major benefits of three-layer and three-tier architectures. 1. Faster Development.
Traditional 3-tier application architecture. We all know about the 3-tier application architecture—it is a client-server architecture with a typical structure consisting of the presentation layer, application layer, and database layer. It has a user interface, business/data access logic, and data access. Many enterprise applications were ...
The three tier architecture decouples presentation layer, business (application) layer and database layer. Typically the business layer and the database layer communicate using the database API. The business layer typically exposes its API for other applications and of course for the (remote) presentation layer. There you should find the REST API.
PS: The three tiers are basically, a presentation layer, business layer and data access layer. Just in case. It depends on the level of detail you want to show. do you want to only show the three tiers and their high level relationships? do you have several packages inside each tier that you want to show in the diagram? or do you want to show ...