21.12 — Overloading the assignment operator
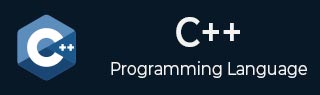
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Decision Making
- C++ Loop Types
- C++ Functions
- C++ Numbers
- C++ Strings
- C++ String Length
- C++ String Concatenation
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ - Advanced Concepts
- C++ Useful Resources
- C++ Questions and Answers
- C++ Quick Guide
- C++ Cheat Sheet
- C++ STL Tutorial
- C++ Standard Library
- C++ Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
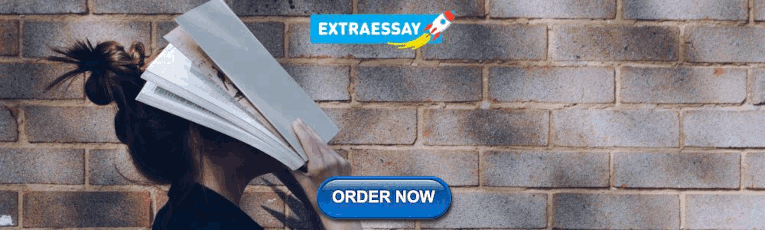
Assignment Operators Overloading in C++
You can overload the assignment operator (=) just as you can other operators and it can be used to create an object just like the copy constructor.
Following example explains how an assignment operator can be overloaded.
When the above code is compiled and executed, it produces the following result −
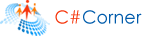
- TECHNOLOGIES
- An Interview Question

Introduction to Python Operators

- Baibhav Kumar
- Aug 30, 2024
- Other Artcile
This article explains Python operators, covering arithmetic, comparison, logical, bitwise, membership, identity, and operator overloading. It includes examples for each, emphasizing their importance in performing operations and comparisons.
In Python, operators are symbols that tell the program to perform specific operations on values or variables. They help you add numbers, compare values, or manipulate data. There are different types of operators, like those for math, comparing things, working with bits, and even creating custom actions. In this guide, we'll break down these operators into simple explanations so you can easily understand how they work.
Python Arithmetic Operators
Arithmetic operators provide a set of operators to perform basic mathematical operations:
- Addition (+): This operator adds two values together.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another, resulting in a floating-point number.
- Floor Division (//): Divides two values and returns the largest integer less than or equal to the result.
- Exponentiation (**): Raises one value to the power of another.
- Modulus (%): Returns the remainder after dividing one value by another.
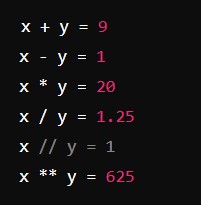
Python Comparison Operators
Comparison operators help compare values and return either True or False. These operators include:
- Greater Than (>): Checks if the value on the left is greater than the value on the right.
- Less Than (<): Check if the value on the left is less than the value on the right.
- Equal To (==): Checks if both values are equal.
- Not Equal To (!=): Checks if both values are not equal.
- Greater Than or Equal To (>=): Check if the value on the left is greater than or equal to the value on the right.
- Less Than or Equal To (<=): Check if the value on the left is less than or equal to the value on the right.
Comparison operators are vital for controlling program flow, particularly in decision-making scenarios like if statements and loops.
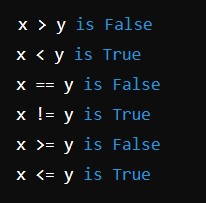
Python Logical Operators
Python logical operators are used to perform logical operations on boolean values, returning either True or False. They are primarily used in conditional statements to combine or negate conditions.
- and: Returns True if both operands are True; otherwise, it returns False.
- or: Returns True if at least one of the operands is True; returns False only if both are False.
- not: Negates the boolean value; if the value is True, it returns False, and vice versa.
Logical operators are used to create complex conditions, enabling more flexible and sophisticated decision-making in programs.
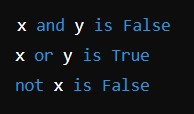
Python Bitwise Operators
Bitwise operators in Python work at the bit level, meaning they perform operations on the binary representation of numbers. These operators manipulate individual bits of data, which can be useful for low-level programming tasks like handling binary data or optimizing performance.
- & (AND): Compares each bit of two numbers. If both bits are 1, the result is 1. Otherwise, it's 0.
- | (OR): Compares each bit of two numbers. If at least one bit is 1, the result is 1. If both are 0, the result is 0.
- ^ (XOR): Compares each bit of two numbers. If the bits are different, the result is 1. If they are the same, the result is 0.
- ~ (NOT): Flips all the bits of a number, turning 1s into 0s and 0s into 1s.
- << (Left Shift): Shifts the bits of a number to the left by a specified number of positions, effectively multiplying the number by powers of two.
- >> (Right Shift): Shifts the bits of a number to the right by a specified number of positions, effectively dividing the number by powers of two.
Bitwise operators are often used in scenarios where performance and memory efficiency are critical, such as in systems programming, cryptography, or data compression.
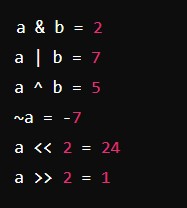
Python Membership Operators
Membership operators in Python are used to check if a value is part of a sequence, such as a list, tuple, string, or set. These operators help determine whether a particular item exists within a collection.
- in: This operator checks if a value exists in a sequence. If the value is found, it returns True; otherwise, it returns False.
- not in: This operator checks if a value does not exist in a sequence. If the value is not found, it returns True; otherwise, it returns False.
Membership operators are commonly used in conditions or loops to verify the presence or absence of elements in data structures like lists, strings, or dictionaries. For example, you might check if a user’s input exists in a predefined list of valid options.
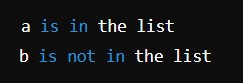
Python Identity Operators
Identity operators in Python are used to compare the memory locations of two objects. They check whether two variables refer to the same object in memory, rather than just having equal values.
- is: This operator returns True if two variables point to the same object in memory, meaning they are identical.
- is not: This operator returns True if two variables do not point to the same object in memory, meaning they are not identical.
Identity operators are helpful when you need to check if two variables reference the same object, particularly when dealing with mutable objects like lists or dictionaries. For instance, in cases where you want to avoid unintentional modifications to a shared object.

Operator overloading in Python allows you to change how operators like +, -, and * work with your custom objects. Normally, these operators work with basic data types like numbers, but with operator overloading, you can define what they do when applied to your objects.
- Customizing Operators: You can change how operators like +, -, and == behave when used with your objects by defining special methods in your class.
- Make Objects Behave Like Built-in Types: This allows your objects to interact with operators in a way that feels natural, just like numbers or strings do.
- Simplifies Complex Data Structures: It is helpful for creating more intuitive code when working with complex objects like matrices, vectors, or custom data types.

To write effective Python code, it's important to understand different operators, like those for math, logic, bitwise operations, checking membership, and comparing identity. Python also lets you extend these operators to work with custom objects, making the language more powerful. Whether you're doing calculations or comparing items, operators are a key part of Python that you'll use often.
- Operator Overloading
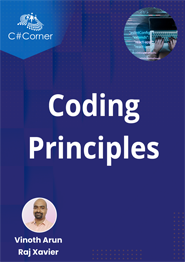
Coding Principles
What is operator overloading?
The mechanism of giving special meaning to an operator is known as operator overloading.
Why is it necessary to overload an operator?
We can almost create a new language of our own by the creative use of the function and operator overloading techniques.
What is an operator function? Describe the syntax of an operator function.
To define an additional task to an operator, we must specify what it means in relation to the class to which the operator is applied. By which function this is done, is called operator function. Syntax of operator function:
How many arguments are required in the definition of an overloaded unary operator?
No arguments are required.
A class alpha has a constructor as follows: alpha(int a, double b); Can we use this constructor to convert types?
No. The constructors used for the type conversion take a single argument whose type is to be converted.
What is a conversion function How is it created Explain its syntax.
C++ allows us to define an overloaded casting operator that could be used to convert a class type data to a basic type. This is referred to conversion function. Syntax:
A friend function cannot be used to overload the assignment operator =. Explain why?
A friend function is a non-member function of the class to which it has been defined as friend. Therefore it just uses the functionality (functions and data) of the class. So it does not consist the implementation for that class. That’s why it cannot be used to overload the assignment operator.
When is a friend function compulsory? Give an example.
When we need to use two different types of operands for a binary operator, then we must use friend function. Example: A = B + 2; or A = B * 2; is valid But A = 2 + B or A = 2 * B will not work. Because the left hand operand is responsible for invoking the member function. In this case friend function allows both approaches.
We have two classes X and Y. If a is an object of X and b is an object of Y and we want to say a = b; What type of conversion routine should be used and where?
We have to use one class to another class type conversion. The type-conversion function to be located in the source class or in the destination class.
State whether the following statements are TRUE or FALSE.
- (a) Using the operator overloading concept, we can change the meaning of an operator.
- (b) Operator overloading works when applied to class objects only.
- (c) Friend functions cannot be used to overload operators.
- (d) When using an overloaded binary operator, the left operand is implicitly passed to the member function.
- (e) The overloaded operator must have at least one operand that is user-defined type.
- (f)Operator functions never return a value.
- (g) Through operator overloading, a class type data can be converted to a basic type data.
- (h) A constructor can be used to convert a basic type to a class type data.
Video Course
Learn on your schedule, stay home, keep learning.
Announcing the Proxy 3 Library for Dynamic Polymorphism

Mingxin Wang
September 2nd, 2024 0 3
We are thrilled to announce that Proxy 3 , our latest and greatest solution for polymorphism in C++, is now feature complete ! Since the library was initially open-sourced , we have heard much positive feedback and received many brilliant feature requests. Big thanks to all of you who have contributed any code or idea that made the library better!
Our Mission
“Proxy” is a modern C++ library that helps you use polymorphism (a way to use different types of objects interchangeably) without needing inheritance.
“Proxy” was created by Microsoft engineers and has been used in the Windows operating system since 2022. For many years, using inheritance was the main way to achieve polymorphism in C++. However, new programming languages like Rust offer better ways to do this. We have improved our understanding of object-oriented programming and decided to use pointers in C++ as the foundation for “Proxy”. Specifically, the “Proxy” library is designed to be:
- Portable : “Proxy” was implemented as a single-header library in standard C++20. It can be used on any platform while the compiler supports C++20. The majority of the library is freestanding , making it feasible for embedded engineering or kernel design of an operating system.
- Non-intrusive : An implementation type is no longer required to inherit from an abstract binding.
- Well-managed : “Proxy” provides a GC -like capability that manages the lifetimes of different objects efficiently without the need for an actual garbage collector.
- Fast : With typical compiler optimizations, “Proxy” produces high-quality code that is as good as or better than hand-written code. In many cases, “Proxy” performs better than traditional inheritance-based approaches, especially in managing the lifetimes of objects.
- Accessible : Learned from user feedback, accessibility has been significantly improved in “Proxy 3” with intuitive syntax, good IDE compatibility, and accurate diagnostics.
- Flexible : Not only member functions, the “abstraction” of “Proxy” allows any expression to be polymorphic, including free functions, operators, conversions, etc. Different abstractions can be freely composed on demand. Performance tuning is supported for experts to balance between extensibility and performance.
The New Look
Comparing to our previous releases, there are some major improvements in the syntax and utilities. Let’s get started with some examples!
Hello World
Here is a step-by-step explanation:
- #include <iostream> : For std::cout .
- #include <string> : For std::string .
- #include "proxy.h" : For the “Proxy” library. Most of the facilities of the library are defined in namespace pro . If the library is consumed via vcpkg or conan , this line should be changed into #include <proxy/proxy.h> .
- pro::facade_builder : Provides capability to build a facade type at compile-time.
- add_convention : Adds a generalized “calling convention”, defined by a “dispatch” and several “overloads”, to the build context.
- pro::operator_dispatch<"<<", true> : Specifies a dispatch for operator << expressions where the primary operand ( proxy ) is on the right-hand side (specified by the second template parameter true ). Note that polymorphism in the “Proxy” library is defined by expressions rather than member functions, which is different from C++ virtual functions or other OOP languages.
- std::ostream&(std::ostream& out) const : The signature of the calling convention, similar with std::move_only_function . const specifies that the primary operand is const .
- build : Builds the context into a facade type.
- pro::proxy<Streamable> p1 = &str : Creates a proxy object from a raw pointer of std::string . p1 behaves like a raw pointer, and does not have ownership of the underlying std::string . If the lifetime of str ends before p1 , p1 becomes dangling.
- std::cout << *p1 : This is how it works. It prints “Hello World” because the calling convention is defined in the facade Streamable , so it works as if by calling std::cout << str .
- pro::proxy<Streamable> p2 = std::make_unique <int>(123) : Creates a std::unique_ptr <int> and converts to a proxy . Different from p1 , p2 has ownership of the underlying int because it is instantiated from a value of std::unique_ptr , and will call the destructor of std::unique_ptr when p2 is destroyed, while p1 does not have ownership of the underlying int because it is instantiated from a raw pointer. p1 and p2 are of the same type pro::proxy<Streamable> , which means you can have a function that returns pro::proxy<Streamable> without exposing any information about the implementation details to its caller.
- std::cout << *p2 : Prints “123” with no surprise.
- Similar with p2 , p3 also has ownership of the underlying double value, but can effectively avoid heap allocation.
- Since the size of the underlying type ( double ) is known to be small (on major 32- or 64-bit platforms), pro::make_proxy realizes the fact at compile-time and guarantees no heap allocation.
- Library “Proxy” explicitly defines when heap allocation occurs or not to avoid users falling into performance hell, which is different from std::function and other existing polymorphic wrappers in the standard.
- std::cout << *p3 : Prints “3.14” with no surprise.
- When main returns, p2 and p3 will destroy the underlying objects, while p1 does nothing because it holds a raw pointer that does not have ownership of the underlying std::string .
More Expressions
In addition to the operator expressions demonstrated in the previous example, the library supports almost all forms of expressions in C++ and can make them polymorphic. Specifically,
- The PRO_DEF_MEM_DISPATCH macro: Defines a dispatch type for member function call expressions.
- The PRO_DEF_FREE_DISPATCH macro: Defines a dispatch type for free function call expressions.
- The pro::operator_dispatch class template: Dispatch type for operator expressions.
- The pro::conversion_dispatch class template: Dispatch type for conversion expressions.
Note that some facilities are provided as macros, because C++ templates today do not support generating a function with an arbitrary name. Here is another example that makes member function call expressions polymorphic:
- #include <sstream> : For std::stringstream .
- #include "proxy.h" : For the “Proxy” library.
- PRO_DEF_MEM_DISPATCH(MemDraw, Draw) : Defines a dispatch type MemDraw for expressions of calling member function Draw .
- PRO_DEF_MEM_DISPATCH(MemArea, Area) : Defines a dispatch type MemArea for expressions of calling member function Area .
- add_convention : Adds calling conventions to the build context.
- support_copy<pro::constraint_level::nontrivial> : Specifies the underlying pointer type shall be copyable, which also makes the resulting proxy type copyable.
- class Rectangle : An implementation of Drawable .
- Function PrintDrawableToString : Converts a Drawable into a std::string . Note that this is a function rather than a function template, which means it can generate ABI in a larger build system.
- pro::proxy<Drawable> p = pro::make_proxy<Drawable, Rectangle>(3, 5) : Creates a proxy<Drawable> object containing a Rectangle .
- std::string str = PrintDrawableToString(p) : Converts p into a std::string , implicitly creates a copy of p .
- std::cout << str : Prints the string.
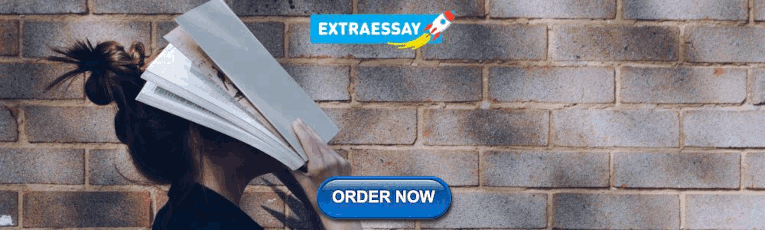
Other Useful Features
In addition to the features mentioned above, here is a curated list of the most popular features based on user feedback:
- Overloading : facade_builder::add_convention is more powerful than demonstrated above. It can take any number of overload types and perform standard overload resolution when invoking a proxy .
- Facade composition : facade_builder::add_facade allows flexible composition of different abstractions.
- Weak dispatch : When an object does not implement a convention, and we do not want it to trigger a hard compile error, it is allowed to define a “weak dispatch” with macro PRO_DEF_WEAK_DISPATCH from an existing dispatch type and a default implementation.
- Allocator awareness : Function template allocate_proxy is able to create a proxy from a value with any custom allocator. In C++11, std::function and std::packaged_task had constructors that accepted custom allocators for performance tuning, but these were removed in C++17 because “the semantics are unclear, and there are technical issues with storing an allocator in a type-erased context and then recovering that allocator later for any allocations needed during copy assignment”. These issues do not apply to allocate_proxy .
- Configurable constraints : facade_builder provides full support for constraints configuration, including memory layout (by restrict_layout ), copyability (by support_copy ), relocatability (by support_relocation ), and destructibility (by support_destruction ).
- Reflection : proxy supports type-based compile-time reflection for runtime queries, specifically with facade_builder::add_reflection and function template proxy_reflect .
We hope this library could empower more C++ users outside Microsoft to write polymorphic code easier. The full documentation of Proxy 3 will be published soon. Please stay tuned for more technical details. We are also actively working on several ISO C++ proposals for further standardization.

Mingxin Wang Senior Software Engineer, Windows Engineering
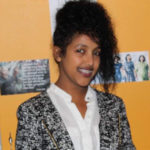
Leave a comment Cancel reply
Log in to start the discussion.

Insert/edit link
Enter the destination URL
Or link to existing content

Snapsolve any problem by taking a picture. Try it in the Numerade app?
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Overloading of function-call operator in C++
In this article, we will discuss the Overloading of the function-call operators in C++ .
- The function call operator is denoted by “()” which is used to call function and pass parameters. It is overloaded by the instance of the class known as a function object .
- When the function call operator is overloaded, an operator function is created that can be used to pass parameters.
- It modifies that how the operator is fetched by the object.
- In Object-Oriented Languages , operator() can be treated as a normal operator, and objects of a class type can call function (named operator() ) like making a function call to any other overloaded operator.
- When the function call operator is overloaded, a new way to call a function is not created rather an operator() function is created that can be passed an arbitrary number of parameters.
Below is the program of taking input in a matrix using friend functions first to overload insertion operator and extraction operator and then overloading operator() for taking input for the i th row and the j th column of the matrix and displaying value at the i th row and the j th column stored in the matrix:
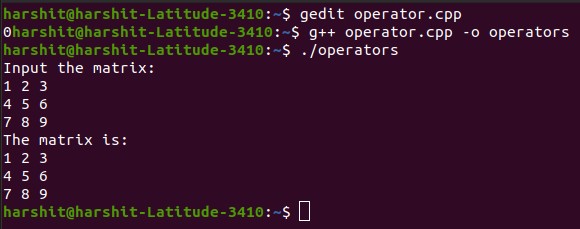
Please Login to comment...
Similar reads.
- C++-Operator Overloading
- cpp-operator
- cpp-operator-overloading
- How to Delete Discord Servers: Step by Step Guide
- Google increases YouTube Premium price in India: Check our the latest plans
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Comparison operator with explicit object parameter of not class type
Can comparison operators for a class in C++23 have explicit object parameter of a type distinct from the class type?
Consider for example
Now the comparison for inequality
is accepted by GCC and Clang, but MSVC complains:
And the comparison for equality
is accepted by GCC only, while Clang already dislikes it:
Online demo: https://gcc.godbolt.org/z/dnKc1fhcT
Which compiler is correct here?
- language-lawyer
- comparison-operators
- explicit-object-parameter
As far as I can tell:
MSVC's error is incorrect. [over.oper.general]/7 places requirements on the types of parameters only for non-member overloads . An explicit object parameter function is a member function. (See also CWG 2931 . The current suggested resolution would make MSVC's behavior correct instead.)
Clang's error message is correct. There is a built-in operator==(int, int) candidate for == , which is not a better or worse match than your operator== overload by any of the overload disambiguations. There is [over.match.oper]/3.3.4 which excludes a built-in candidate if it has a matching parameter type list with one of the non-member candidates, but again, only for non-member candidates and the overload with explicit object parameter is a member candidate.
In case of static_assert( A{0} != A{1} ); there is no problem, because this will prefer the built-in operator!=(int, int) candidate over any rewritten candidate that uses your operator== overload. Both GCC and Clang ignore your overload.
This would mean that such overloads are permitted, but of limited practical use, because they will mostly be ambiguous with the built-in candidate. Maybe [over.match.oper]/3.3.4 should apply to member functions as well, comparing the parameter list with object parameters instead.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ language-lawyer comparison-operators c++23 explicit-object-parameter or ask your own question .
- The Overflow Blog
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- A SF novel where a very poor girl finds a "talking book" meant for daughters of extremely rich people
- Download facebook on ubuntu
- Admissibility of withdrawn confession
- How did Dwight Dixon acquire Charles Charles' watch?
- Is this a 'standard' elliptic integral?
- Conservation of the determinant of density matrix
- Are there probabilistic facts of the matter about the universe?
- Reheating beans makes them mushy and unpeeled
- Is the Oath Formula "By the Life of Pharaoh" Attested Anywhere outside of Biblical Literature?
- Find the radius of a circle given 2 of its coordinates and their angles.
- What makes the spring equinox happen on october instead of fall on the north hemisphere?
- Is consciousness a prerequisite for knowledge?
- How do Trinitarian Christians defend the unfalsifiability of the Trinity?
- decode the pipe
- Replace a string in a script without modifying the file, and then to execute it
- Why is there an "unnamed volcano" in Syria?
- Risks of exposing professional email accounts?
- What happens to entropy during compression?
- A story where SETI finds a signal but it's just a boring philosophical treatise
- Velocity dispersion of stars in galaxies
- How can I align this figure with the page numbering?
- Replicating Econometrics Research
- Should you refactor when there are no tests?
- How to reproduce this equation itemization?
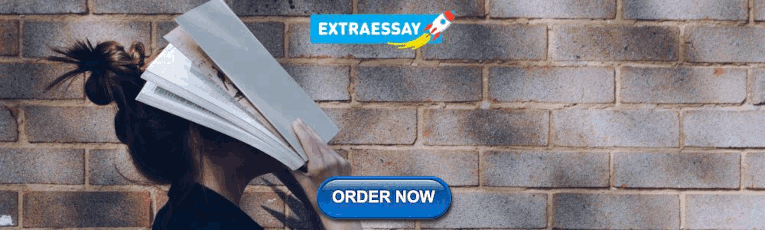
IMAGES
VIDEO
COMMENTS
21.12 — Overloading the assignment operator. Alex July 22, 2024. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Overloading assignment operator in C++ copies all values of one object to another object. Only a non-static member function should be used to overload the assignment operator. In C++, the compiler automatically provides a default assignment operator for classes. This operator performs a shallow copy of each member of the class from one object ...
There is already a special instance of overloading = in place that the designers deemed ok: property setters. Let X be a property of foo. In foo.X = 3, the = symbol is replaced by the compiler by a call to foo.set_X(3). You can already define a public static T op_Assign(ref T assigned, T assignee) method.
1) Do not allow assignment of one object to other object. We can create our own dummy assignment operator and make it private. 2) Write your own assignment operator that does deep copy. Same is true for Copy Constructor. Following is an example of overloading assignment operator for the above class. #include<iostream>.
C++ compiler implicitly provides a copy constructor, if no copy constructor is defined in the class. A bitwise copy gets created, if the Assignment operator is not overloaded. Consider the following C++ program. Explanation: Here, t2 = t1; calls the assignment operator, same as t2.operator= (t1); and Test t3 = t1; calls the copy constructor ...
Assignment Operators Overloading in C++ - You can overload the assignment operator (=) just as you can other operators and it can be used to create an object just like the copy constructor.
The commented assignment operator overloading is my attempt to do what I want, I thought it might provide a better description than the one above the snippet. I want to be able to do the following:
C++ Operator Overloading. C++ has the ability to provide the operators with a special meaning for a data type, this ability is known as operator overloading. Operator overloading is a compile-time polymorphism. For example, we can overload an operator '+' in a class like String so that we can concatenate two strings by just using +.
In this lesson, you will learn. Operator Overloading; Non Overloadable Operator; Types of Operator Overloading in C++ . Operator Overloading. Operator overloading in C++ is a specific case of polymorphism in which the operator is overloaded to provide a special meaning to the objects of user-defined data types.. Basically, It adds some features or redefines the functionality of already ...
Normally, these operators work with basic data types like numbers, but with operator overloading, you can define what they do when applied to your objects. Customizing Operators: You can change how operators like +, -, and == behave when used with your objects by defining special methods in your class.
(a) Using the operator overloading concept, we can change the meaning of an operator. (b) Operator overloading works when applied to class objects only. (c) Friend functions cannot be used to overload operators. (d) When using an overloaded binary operator, the left operand is implicitly passed to the member function. (e) The overloaded ...
0 I have a C++ class template for representing real- and complex valued 2D fields. I'd like to overload the assignment operator to achieve deep-copying the data from one field to another. For now, I've restricted the data to either double or std::complex<double>.
The pro::operator_dispatch class template: Dispatch type for operator expressions. The pro::conversion_dispatch class template: Dispatch type for conversion expressions. Note that some facilities are provided as macros, because C++ templates today do not support generating a function with an arbitrary name.
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other ...
VIDEO ANSWER: The class Stuff has both a copy constructor and an overloaded = operator. Assume blob and clump are both instances of the Stuff class. For each statement below, indicate whether the copy constructor o
The assignment operator can't be overloaded as a stand-alone (non-member) function. If you have control of the class, and can modify it, you can make a conversion operator: operator double() const { return todouble(); } It must still be a member function though. double is not a class and does not have members.
The Subscript or Array Index Operator is denoted by ' []'. This operator is generally used with arrays to retrieve and manipulate the array elements. This is a binary or n-ary operator and is represented in two parts: The postfix expression, also known as the primary expression, is a pointer value such as array or identifiers and the second ...
4. Correct me if I'm wrong: I understand that when having a class with members that are pointers, a copy of a class object will result in that the pointers representing the same memory address. This can result in changes done to one class object to affect all copies of this object. A solution to this can be to overload the = operator.
The function call operator is denoted by " ()" which is used to call function and pass parameters. It is overloaded by the instance of the class known as a function object. When the function call operator is overloaded, an operator function is created that can be used to pass parameters. It modifies that how the operator is fetched by the ...
std::unique_ptr and std::shared_ptr have an optional custom deleter parameter. How is it implemented? And how it is possible to implement it in my own class? P.S not the operator delete overloading but a function that will be called instead of delete, like in this case: std::unique_ptr<int, decltype([](int* p) { free(p);})> ptr So how is it possible to pass a deleter as a template parameter ...
Can comparison operators for a class in C++23 have explicit object parameter of a type distinct from the class type? Consider for example. struct A { int i; constexpr bool operator==(this int x, int y) { return x == y; } constexpr operator int() const { return i; } }; ... There is a built-in operator==(int, int) candidate for ==, which is not a ...